Purpose of a Function Prototype in C
A function prototype in C is a declaration of a function that specifies the function's name, return type and parameters. It has the following syntax:
return_type function_name(parameter_list);
For example, the prototype for a function that takes two integers and returns their sum might look like this:
int add(int a, int b);
Function prototypes are typically placed in header files, which are included in source files that call the function. This allows the compiler to verify that the function is being called with the correct number and type of arguments.
Function prototypes are also useful for documenting the intended use of a function and for creating function pointers.
Purpose of function prototype
The purpose of a function prototype in C is to provide the compiler with information about a function's name, return type, and parameters. This allows the compiler to check the correctness of calls to the function, ensuring that the function is called with the correct number and type of arguments.
Function prototypes serve several important roles in C programming:
- They allow the compiler to check the correctness of calls to the function, ensuring that the function is called with the correct number and type of arguments. If a function is called with the wrong number or type of arguments, the compiler will issue an error.
- They document the intended use of the function, including the names and types of its parameters and its return type. This can be helpful for other programmers who may use the function in their own code.
- They allow the creation of function pointers, which are variables that store the address of a function. Function pointers can be used to call functions dynamically, based on the value stored in the function pointer.
- They allow the compiler to allocate space for the function's return value and parameters on the stack before the function is called. This is necessary because the compiler needs to know the size of these values in order to allocate the necessary space on the stack.
Overall, the purpose of a function prototype in C is to provide the compiler with information about a function's signature, which allows it to check the correctness of calls to the function and perform other necessary tasks related to function execution.
Here are some additional details about function prototypes in C:
- Function prototypes are typically placed in header files, which are included in source files that call the function. This allows the compiler to verify that the function is being called with the correct number and type of arguments.
- A function prototype consists of the function's return type, name, and parameter list. The parameter list specifies the types of the function's parameters in the order they are expected to be passed to the function. For example, the prototype for a function that takes two integers and returns their sum might look like this:
int add(int a, int b);
- If a function takes no parameters, the parameter list is left empty. For example, the prototype for a function that takes no parameters and returns an integer might look like this:
int getAnswer();
- In C, it is not required to provide a function prototype for every function. However, it is generally considered good practice to do so, as it helps ensure that the function is called correctly and helps document the intended use of the function.
- Function prototypes can also be used to declare functions with variable-length argument lists. These functions use the ellipsis (...) notation in their parameter list to indicate that they can take any number of arguments. For example, the prototype for a function that takes a variable number of integers and returns their sum might look like this:
int sum(int num_values, ...);
Here is an example of a function prototype in C:
#include <stdio.h>
// Function prototype for add()
int add(int a, int b);
int main(void) {
int x = 3, y = 4;
int z = add(x, y); // Correctly calls add()
printf("%d + %d = %d\n", x, y, z);
return 0;
}
// Function definition for add()
int add(int a, int b) {
return a + b;
}
Output:
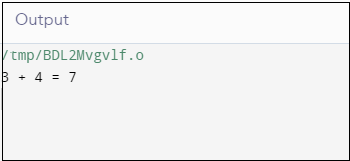
In this example, the function prototype for add() is placed at the top of the file, before the main() function. This allows the compiler to verify that the call to add() in main() is correct. The function definition for add() is placed after main() and it contains the actual code for the function.
When the program is compiled and run, it will print "3 + 4 = 7" to the console.