Remove an element from an array in C
A collection of objects or pieces of the same data type stored in a single memory block is known as an array. A data structure called an array is used in C programming to hold values of basic data types like int, char, float, etc. We'll start by looking at how to delete or remove a certain element from an array in C.
The user must specify the point from where the array's element should be deleted to remove a specific element from an array. The element's deletion has no impact on the array's size. We must also establish whether it is feasible to delete data from an array.
Let's say, for instance, that an array has seven elements, arr[] = (12,27,16,4,10,15,5); and the user wants to delete an element 8. The user must first locate the 4th element, the 8th element, to decide whether or not the deletion is possible. No element should be higher than the total number of elements in an array. The user wishes to remove the element in the eighth place of the array, which is not allowed because there are 7 elements in it.
Steps to remove an element from an array
Step 1: Enter the array's size using the num variable, define the position using the position variable, and the counter value using the i variable.
Step 2: Insert elements into an array using a loop until i num is satisfied.
Step 3: At this stage, the user or programmer should provide the location of the precise element they wish to remove from an array.
Step 4: Compare an element's position (pos) to the total number of elements (num+1). The element cannot be deleted if the position is greater than num+1; move on to step 7 instead.
Step 5: Shift the remaining array members to the left while removing the specific element.
Step 6: After an element has been deleted or removed from an array, display the final array.
Step 7: Stop the programme or leave it.
Remove element from array Program
#include <stdio.h>
#include<conio.h>
int main()
{
int array[100]; //Array Initialization
int position, c, n;//declare the variables
printf("Enter number of elements in array\n");/* asks the user to specify the array's size */
scanf("%d", &n);
// put the array's elements in
printf("Enter %d elements\n", n);
for (c = 0; c < n; c++)
scanf("%d", &array[c]); // making loop ends.
printf("Enter the location where you wish to delete element\n");
// seeks the user's position
scanf("%d", &position);
if (position >= n+1)
printf("Deletion not possible.\n");
else
{
for (c = position - 1; c < n - 1; c++)
array[c] = array[c+1];//for loop ends
printf("Resultant array:\n");
//Displays array
for (c = 0; c < n - 1; c++)
printf("%d\n", array[c]);//for loop ends
}
return 0;
}//main function ends
Output
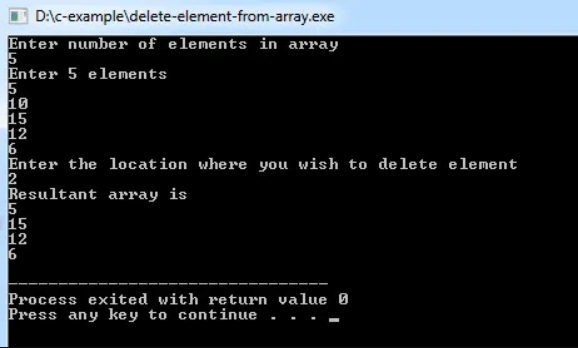
The array's size is unaffected by the deletion of an element. Additionally, the likelihood of deletion is examined.
A user-defined size array with a deleted element is provided.
Operations involved:
Step 1: Array input
Step 2: Element to be deleted
Step 3: Traversing the array till we reach the element
Step 4: Delete that element, and the resultant array size is reduced by one unit
For instance, if an array has five elements and you try to delete the element at position 6, it will not work.
Example
#include <stdio.h>
#include <conio.h>
int main()
{
int array[100]; // initialising an array.
int position, c, n; // variables must be declared
printf("Enter number of elements in array\n");
// enter the array's size
scanf("%d", &n);
printf("Enter %d elements\n", n);
// Put the array's components in.
for ( c = 0 ; c < n ; c++ )
scanf("%d", &array[c]); //for loop ends
printf("Enter the location where you wish to delete element\n");
scanf("%d", &position);
if ( position >= n+1 )
printf("Deletion not possible.\n");
else
{
for ( c = position - 1 ; c < n - 1 ; c++ )
array[c] = array[c+1]; //for loop ends
printf("Resultant array is\n");
//displays array
for( c = 0 ; c < n - 1 ; c++ )
printf("%d\n", array[c]);
//for loop ends
}
return 0;
}//main function ends.
Output
The array's element count should be entered.
5
Enter 5 components.
4
12
22
34
45
When deleting an element, enter its desired position.
2
The resultant array is
4
22
34
45