Types Of Structures In C
We can normally store elements of the same datatype with the help of an array in C programming. We can store multiple numbers of elements of a character data type using strings. Still, there is a need in programming where we need to store multiple elements of different data types for example; when we collect data of students where name is of character data type. The second type of data that a roll number has is a number.
Structures
In order to store multiple elements having different data types, the structure is used. The structure can hold "n" number of elements means any number of elements. Now let us move into syntax.
Syntax
struct structurename
{
datatype ele1;
datatype ele2;
.
.
.
.
};
We always need to start with the “struct” keyword and add the structure name, which can be of the programmer's wish. Next, we need to open the parenthesis and declaration. Finally, close the parenthesis after declaring all elements along with datatypes. Adding a semi-colon at the end is important.
Example
struct Customer
{
int customerid;
char name[20];
};
main()
{
int a;
Struct Customer c[25];
}
We know that we need to add the data type and then the variable name while declaring variables in the main function. For structure, the data type name will be "struct structurename," which means here it is "struct Customer" and then we need to add a variable name, which is "c" here now, and the memory will be allocated to the variable.
The structure variable always holds the base address; this only points to the datatype, but we don't have a pointer here because it is an internal pointer value. 24bytes memory is allocated to the structure variable that is "c" as we have named [20]; each character occupies a 1-byte memory character means 20 bytes for 20 characters, and one integer variable, which is 4 bytes in a 64-bit system, so the total is 24 bytes.
Till here, we stored information now; let us move to access part
Accessing
We can access structures simply using the dot "." operators like “c.customerid” and “c.name”.
We must know how to declare the size of the structure before proceeding.
Size of Structure
By using "sizeof" keyword, we can find the size, sizeof(variable name). We can also use sizeof(struct strcutname).
Check the below example to get a quick idea.
#include<stdio.h>
struct Customer
{
int customerid;
char name[20];
};
void main()
{
struct Customer c;
printf("size of Customer structure is %lu \n",sizeof(c));
printf("size of Customer is %lu ",sizeof(struct Customer));
}
Output
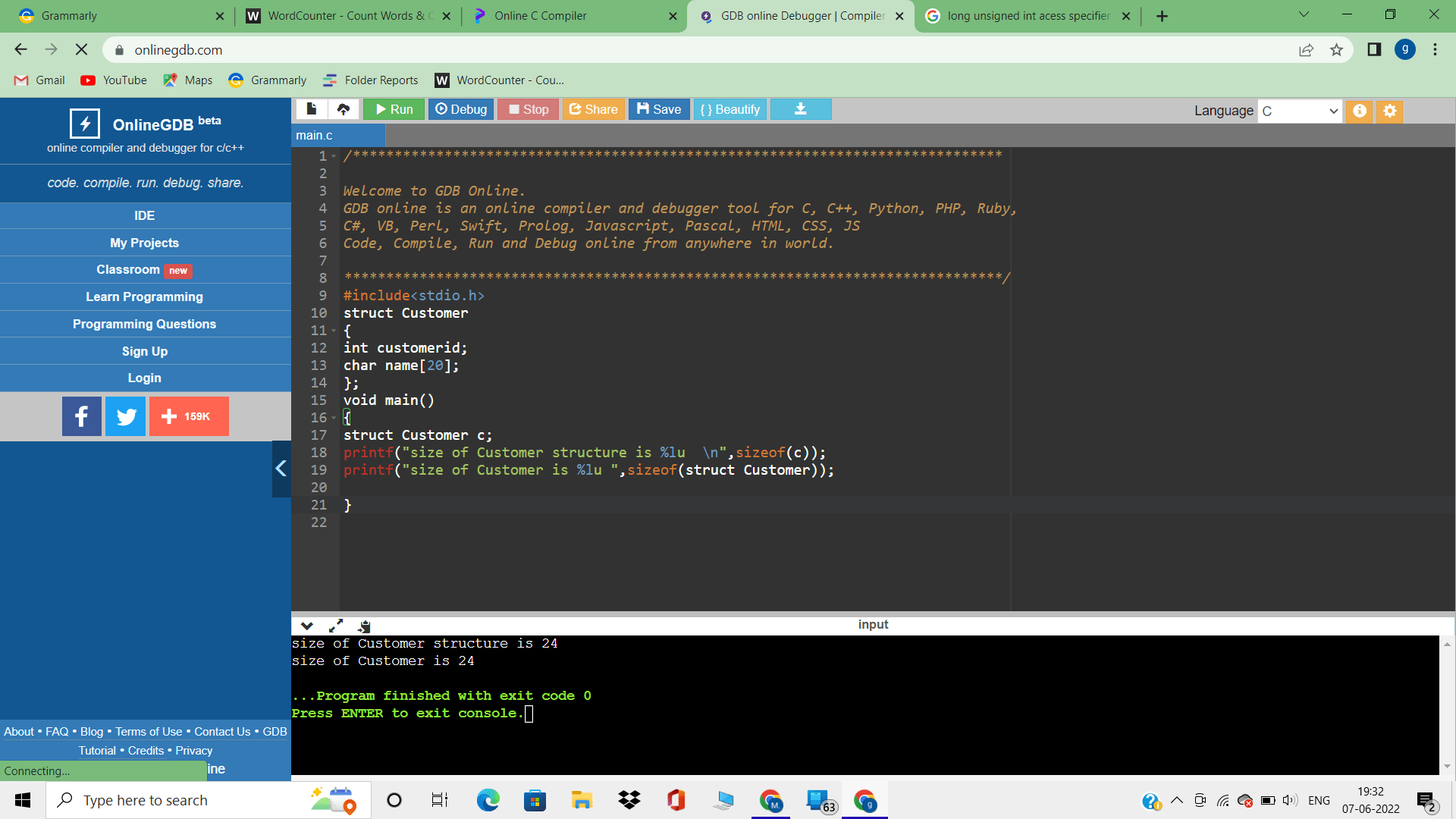
Here we used “%ul” which is for unsigned long or unsigned int; we can also use %d, but the C compiler will show a small warning. Finally, we can pass either variable or datatype name in sizeof().
Now, let us move into types of structures. Mainly, we have two types of structures.
1. Global Structure
The structure definition which is common for all functions that are entire program, is called global structure.
Global Structure Declaration
#include<stdio.h>
struct globalstruct
{
int a,b;
};
void main()
{
struct globalstruct g;
}
int try()
{
struct globalstruct l;
//It also executes here
}
Output
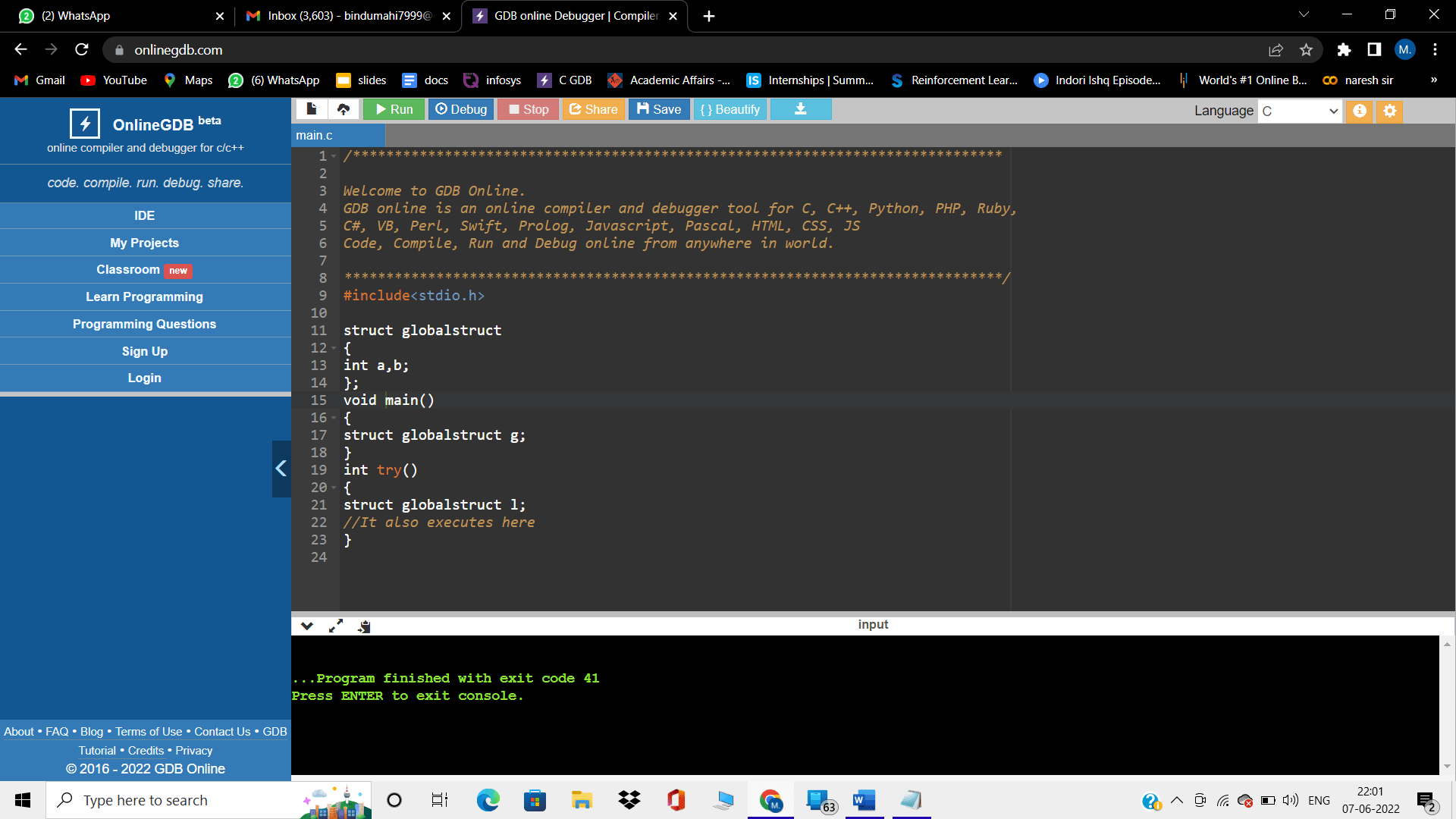
Here program executed successfully.
2. Local Structure
The structure which is only for a specific function is called Local Structure. Simply the declaration of structures inside a particular function here.
Declaration of local structure
#include<stdio.h>
void main()
{
struct localstruct
{
int a,b;
};
struct localstruct l;
//It will work here
}
int try()
{
struct localstruct l;
//It won't execute here
}
Output:
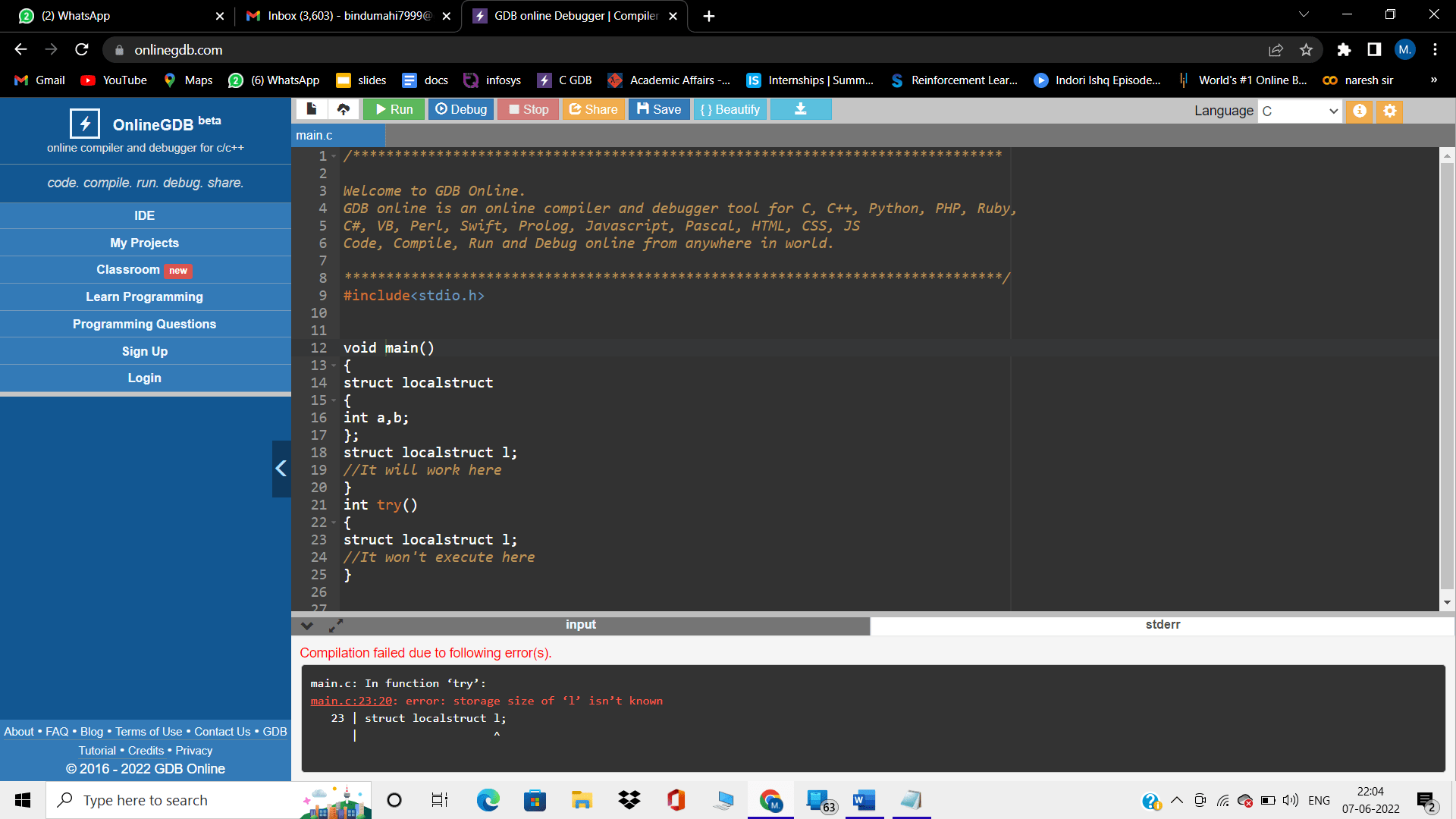
Here we can access it only with the main function. That is why we got a compilation error that “l” is not known. Simply it is like the local and global variables concept.
Advantages
1. It is possible to create datatypes with different data types, unlike arrays and strings. For example, if we want to store student records with the students' names, roll numbers, grades, and addresses, the items will have different types. For example, the roll number will have an integer number type, the name will have a string type, the grades will have a floating-point type, and the address will be a string type.
2. It reduces complexity and increases productivity.
3. It eliminates a lot of the burden of storing in different files as it is heterogenous.
4. It enhances the maintainability of code.
5. It is very much suitable for some mathematical operations also.