Difference between while and do-while loop in C
Introduction
Both 'While' and 'Do-While' loops in C mostly have a similar concept, and the code of both loops runs for mainly similar purposes. Both loops process the program/code in the same manner. But, there is a sole and significant difference between them. In the do-while loop, the execution of the body takes place at least one time before checking the termination condition. Then it executes the command according to the termination condition. Whereas, in the case of the while loop, the termination condition is checked before the execution of the body.
In other words, in the do-while loop, the body is executed first, and after that, it checks whether the termination condition results in true or false. In contrast, in the while loop, it first executes the termination condition and checks whether the results in true or false. If the result is True, then the statement is executed, otherwise not.
Difference between the syntax
Syntax of while loop:
while (termination condition) {
// the body of the loop
}
Syntax of the do-while loop:
do
{
/* main body used for the loop */
}
while (termination condition);
Processing of Loop
While loop in C:
- Initially, we use the parentheses for storing the termination condition of the While loop.
- Then, there can be two outcomes.
- Firstly, if the result is true, the control will automatically execute the While loop.
- It will continue to evaluate again and again until the termination condition is false.
- Secondly, if the result is false, the control will come out of the While loop, and the While Loop will be dismissed.
Do-while loop in C:
- Initially, one-time execution of the body of Do-While takes place. After that, it executes the termination condition.
- It then checks whether the termination command results in true. If yes, it will print the desired outcome on the screen, and it will continue to execute the termination condition again and again.
- One more thing is that the execution will run multiple times until the condition become false.
- As soon as the results become false after the execution of the termination command, then the loop will be automatically dismissed.
Digrammatical Representation of loop with a flow chart
While loop in C:
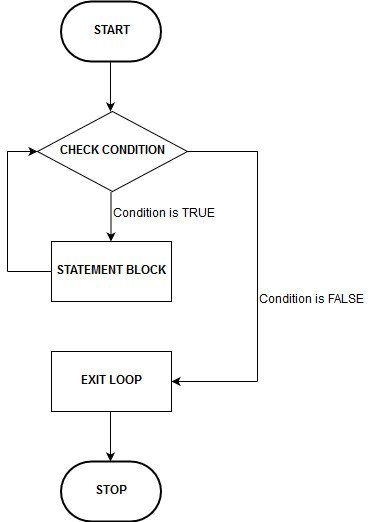
Do while loop in C:
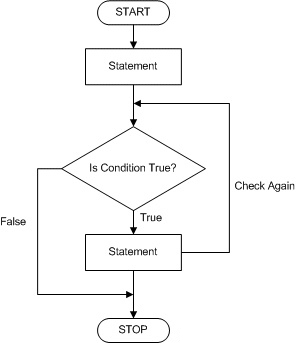
Examples of Loop
While Loop:
/* Printing integers from 1 to 10 */
#include <stdio.h>
int main ()
{
int a = 1; // initializing the value of a to 1
while (a <= 10) // termination condition
{
Printf ("the value of ‘a’ is %d\n", a); // body of the while-loop
++a; // increment/ decrement statement
}
return 0;
}
Output:
the value of a is 1
the value of a is 2
the value of a is 3
the value of a is 4
the value of a is 5
the value of a is 6
the value of a is 7
the value of a is 8
the value of a is 9
the value of a is 10
Explanation of Code:
The first step is to initialize the value of int a.
Then, we applied a termination condition (while (a <= 10)) through which we can avoid the code from running to infinity.
After that, the main body of the while-loop is implemented just like [printf ("the value of ‘a’ is %d\n", a);]. The main body can print the desired output that we want (see output).
Then, we placed an increment statement (++a;), which increments the value of a one by one.
The code will print "the value of a is 1" as we initialized a to 1. After that, it will perform the increment command and will add 1.
It will evaluate again and checks that '2' is also less than 10. Therefore, the code will print "the value of a is 2".
This evaluation will take place several times until the value becomes 11. The code will terminate the entire process when the termination condition becomes false.
Do-while loop:
/* code for adding integers until the user assigns the value zero */
#include <stdio.h>
int main()
{
double number, total = 0;
do
{
printf("Enter a number: ");// body of do-while loop
scanf("%lf", &number); // it is executed only once
total += number;
}
while(number != 0.0);
printf("Sum = %.2lf",total);
return 0;
}
Output:
Enter a number: 3
Enter a number: 4
Enter a number: -5
Enter a number: 6
Enter a number: 0
Sum = 8.00
Explanation:
In the above program, we used the do-while loop, which processes the code in the following manner:
It lets the user enter the numbers, and the loop is executed until the user inserts 0. If the condition becomes false, the loop terminates, and the code adds all the entered numbers before zero and displays the sum of those numbers on the screen.