SJF Scheduling Program in C
The SJF (shortest job first) or the shortest job next is the programming scheduling in the C. It is one of the CPU scheduling programming. The SJF is defined as the scheduling process or the scheduling policy in the algorithm that prescribes the waiting time process into the execution time next.
There are Two types of SJF are there:
- Non-Preemptive SJF
- Pre-emptive SJF
These are the algorithms are serialized in the order which has the minimum or least average Time.
The SJF can be considered in three aspects:
- Burst Time
- Average TAT (Turn Around Time)
- Average WT (Waiting Time)
Characteristics of the SJF Scheduling in C
- The first advantage of the shortest job first is it calculate the minimum average of the waiting time from all of the scheduling processed algorithms.
- It is one of the Greedy algorithms.
- It can be ready for the CPU process that can wait with the low priority due to it runs indefinitely.
- The shortest First job can be used in the specialized scenario where it estimates the accurate running time.
Algorithm for the SJF
- Firstly, we have to sort all the processes with respective to its arrival time.
- After that we have to select the processes which have the minimum arrival and burst time.
- The processes after the completion we have to sort the processes that will wait for completing the previous processes and we have to select the processes which having the least Burst time value.
Let's take an Example for SJF (shortest job First)
Processes | Burst Time |
P1 | 5 |
P2 | 8 |
P3 | 2 |
P4 | 7 |
1. The process P1 second because it has the burst time value as 5 which is greater than P3 and less than the P2 and P4.
Then the waiting time of the P1 will be consider as 2 (I.e., P1 = 2).
2. Secondly, the process P2 will be executed as it consists of the greatest burst time of the value 8. which is greater than the P1, P3, P4.
Then the waiting time of the P2 will be considered as 14(P1+P3+P4).
3. Later on the P2, the Process P3 will be executed and it has the burst time value as 2, which is less than the P1, P2, P3. It will be executed First.
The waiting time for P3 = 0.
4. Then the Process P4 will be executed it has the Burst time value as 7 which is greater than the P1, P3 and less than the P2.
The waiting time for the process P4 will be considered as 7(P1+P3).
Computing the SJF using an example program.
- The completion Time defined as the time calculated after the process completes the Execution part.
- The Turn Around Time is the difference between Completion time and the Arrival time.
TAT = (Completion – Arrival) Time.
- Waiting Time will be calculated as the difference between the TAT (Turn Around Time) and the Burst Time.
Waiting Time = (TAT – Burst Time).
Let’s see an example program for Non-preemptive SJF
#include <stdio.h> // preprocessor
intmain() // main function
{
intN[20][4]; // we are using matrix to store values
// Time, Average WT & Average TAT
inti, j, x, sum = 0, pos, temp;
float avgwt, avgtat; // Average WT & Average TAT
printf("Enter the number of the processes: ");
scanf("%d", &x);
printf("Enter the Burst Time value:\n");
for (i = 0; i< x; i++) // allocating values for processes
{
printf("Processes: ", i + 1);
scanf("%d", &N[i][1]);
N[i][0] = i + 1;
}
for (i = 0; i< x; i++) // soring the Burst Time value
{
pos = i;
for (j = i + 1; j < x; j++)
if (N[j][1] < N[pos][1])
pos = j;
temp = N[i][1];
N[i][1] = N[pos][1];
N[pos][1] = temp;
temp = N[i][0];
N[i][0] = N[pos][0];
N[pos][0] = temp;
}
N[0][2] = 0;
// Calculating the Waiting Times values in SJF
for (i = 1; i< x; i++)
{
N[i][2] = 0;
for (j = 0; j <i; j++)
N[i][2] += N[j][1];
sum += N[i][2];
}
avgwt = (float)sum / x;
sum = 0;
printf("processes Burst Time Waiting Time Turnaround Time\n");
for (i = 0; i< x; i++) // calculating the TAT values and printing
{
N[i][3] = N[i][1] + N[i][2];
sum += N[i][3];
printf("processes %d %d %d\n", N[i][0],
N[i][1], N[i][2], N[i][3]);
}
avgtat = (float)sum / x;
printf("\nAverage Waiting Time is calculated = %f", avgwt);
printf("\nAverage Turn Around Time is calculated= %f", avgtat);
}
Output:
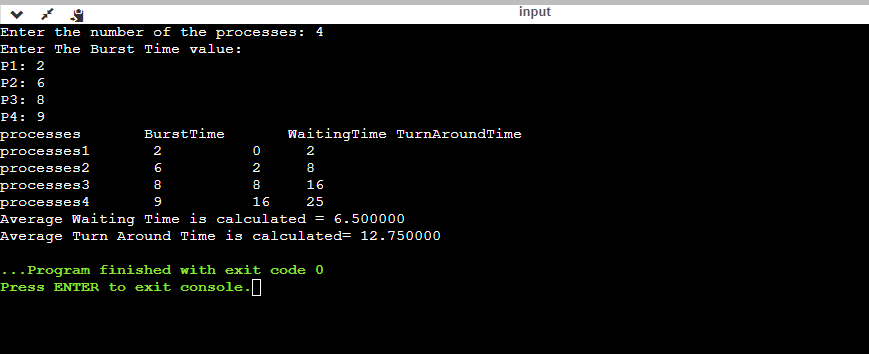
In the following programming we calculated the Average waiting time and the average Turnaround Time with respective to their jobs.
Let's see an example program for pre-emptive SJF scheduling in c:
#include <stdio.h>
intmain()
{
intat[100], bt[100], temp[100];
inti, least, count = 0, time, n;
double wt = 0, tat = 0, end;
float averageWt, averageTat;
printf("\nEnter the Total Number of the Processes:");
scanf("%d", &n);
printf("nEnter the Details of the %d Processes\n", n);
for(i = 0; i< n; i++)
{
printf("\nEnter the Arrival Time of Processes:");
scanf("%d", &at[i]);
printf("Enter the Burst Time of Processes:");
scanf("%d", &bt[i]);
temp[i] = bt[i];
}
bt[99] = 10000;
for(time = 0; count != n; time++)
{
least = 99;
for(i = 0; i< n; i++)
{
if(at[i] <= time &&bt[i] <bt[least] &&bt[i] > 0)
{
least = i;
}
}
bt[least]--;
if(bt[least] == 0)
{
count++;
end = time + 1;
wt = wt + end - at[least] - temp[least];
tat = tat + end - at[least];
}
}
averageWt = wt / n;
averageTat = tat / n;
printf("\n The Average Waiting Time of Processes:%lfn", averageWt);
printf("\n The Average Turn Around Time of Processes:%lfn", averageTat);
return 0;
}
Output:
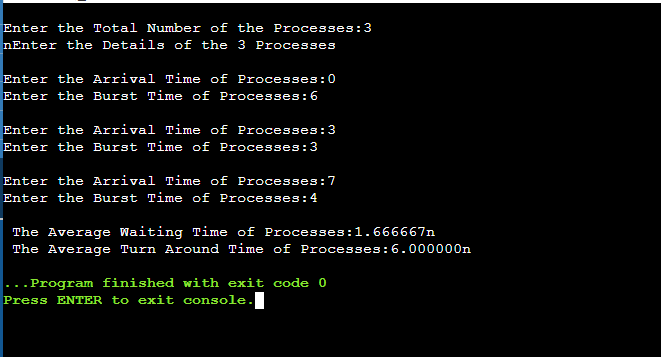