Java Array vs ArrayList
As we all know, arrays are linear data structures that allow you to add elements to them continuously in memory address space, whereas ArrayList is a Collection framework class. Despite knowing the distinctions between the two, a competent programmer already knows to choose ArrayList over arrays. Now, even with ArrayList, there's a way to specify the data type of the elements that should be saved in the ArrayList, such as an object, string, integer, double, float, and so on.
Java Array
A Java array is an object that stores objects and primitive data types, given the elements stored in an array are of the same data type. The elementsin the array are stored at a contagious memory location. An array has a fixed length, i.e., once the size of the array is declared, it can't be changed. In an array, the index of elements starts with zero. If we want to access the second element, we will be referring to the 1st index. Array inherits Object class, and it is a Data Structure. It implements the following interfaces:
- Serializable
- Cloneable
Let’s see an example.
ArrayExample.java
public class ArrayExample {
public static void main(String[] args) {
int[] a = {1,2,3,4,5,6};
for(int i = 0; i<a.length; i++)
{
System.out.println(a[i]);
}
}
}
Output:
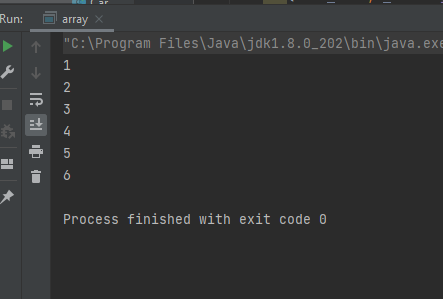
ArrayList in Java
The components of the Java ArrayList class are stored in a dynamic array. It's similar to an array, except there's no limit to how big it may be. At any time, we can add or remove items. As a result, it is far more adaptable than a typical array. The java.util package contains it. It's similar to C++'s Vector.
In Java, an ArrayList can have duplicate entries as well. Internally, the ArrayList keeps track of the insertion order. It is derived from AbstractList and implements the List interface.
Because it implements the List interface, we can utilize all of the List interface's methods here.
- Duplicate items are possible in the Java ArrayList class.
- The ArrayList class in Java keeps track of insertion order.
- The following are the key features of the Java ArrayList class:
- Because the array is indexed, the Java ArrayList allows for random access. The Java ArrayList class does not support synchronization.
- Because a lot of shifting needs to happen if any element is deleted from the array list, manipulation in ArrayList is a little slower than in LinkedList in Java.
Let’ see an example of ArrayList.
ArrayListExample.java
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
int[] arr = new int[2];
arr[0] = 1;
arr[1] = 2;
System.out.println(arr[0]);
ArrayList<Integer> arrL = new ArrayList<Integer>(2);
arrL.add(1);
arrL.add(2);
System.out.println(arrL.get(0));
}
}
Output:
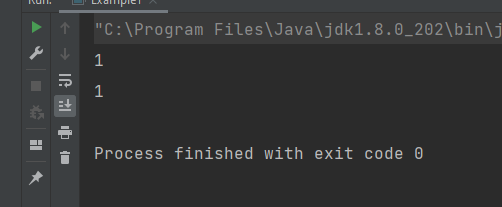
Similarities Between Array and ArrayList
- Add and get method: The Array and Array list performance is similar for add and get operations.
- Both processes take place in constant time.
- Duplicate items: Duplicate elements can exist in both arrays and array lists.
- Null Values: Both may store null values and refer to their elements using indexes.
- Unordered: Neither guarantees the presence of ordered items.
The following table summarizes the difference between Array and ArrayList.
Array | ArrayList |
We use length() method to find the length of Array | We use size() method to find the length of Array List. |
Comparatively Faster. | Comparatively slower. |
It can be one-dimensional as well as Two-Dimensional. | It can be only one-dimensional. |
Once the size of the array is declared, it cannot be changed. | The size of the array list can be changed. |
To access and traverse the elements of an array, we use the array's name with the required index number. | To access or traverse the array list, we need to use methods such as to get () and set () to do so. |
There is no option to delete a certain element. | We can delete a certain element. |
It stores primitive as well as objects. | It stores only objects. |
It is Static in nature. | It is Dynamic in nature. |
It is a part of Core Java Programming. | It is a part of Collection Frameworks. Thus, it has many methods. |
It is used when the need is to use a data structure of Fixed length | It is used when the size is not fixed. |
Conclusion
Arrays and ArrayLists are alternatives, although most developers have ceased using Arrays due to the size issue they pose. Because ArrayLists are less difficult and easier to use, their performance and drawbacks are entirely overlooked. ArrayLists are used in many applications since they reduce time during development and allow developers to push their boundaries. Both have advantages and cons, and the developer must always make the final selection.