Bit Fields in C
The size of a structure in C is specified in bits. The primary goal of it is to use memory efficiently, once we understand that a bit's value must fall within a certain range and not exceed a certain threshold.
Example:
#include<stdio.h>
//presentation of data done
struct date {
//declaring variables
unsigned int d;
unsigned int m;
unsigned int y;
};
int main ()
{
printf (“size of date is %lu bytes \n”, //printing the statement
Size of (struct date));
struct date dt = {31, 12,2014};
printf (“Date is %d%d%d”, dt. d, dt.m, dt.y);
}
Output:
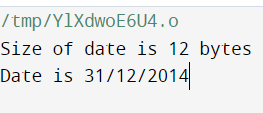
From the above code, we know that the date takes 12 bytes but as we know 4 bytes is taken by unsigned int and we also know date is always from the values 1 to 31 and month is 1 to 12.
Example using signed integers
#include<stdio.h>
//space develop for presentation of date
struct date {
// d taking values between the range of 0 to 31 bytes, 5 bits
int d:5;
// m takes Values between 0 to 12, so 4 bits
int m:4;
int y;
};
int main ()
{
printf (“date size is %lu bytes \n”, size of (struct date));
struct date dt = {31,12,2014};
printf (“date is %d%d%d”, dt. ,dt.m, dt. y);
return 0;
}
Output:
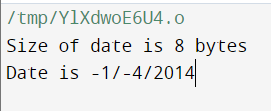
From the above result, we can see that the value obtained is negative because as 31 is equals to 11111 as it is stored in 5-bit signed integer and the most significant bit is 1, to obtain its original value we should calculate it by 2’s compliment, after calculating we obtain 00001 which is equals to decimal number 1 so as the number is negative it shows -1. Similarly, it happens with 12 equals to 1100 stored in 4-bit Signed int by calculating with 2’s compliment we get -4.
Amazing facts related to bit fields in C
- To force alignment special unnamed of bit field, size 0 is used on another boundary
Example:
#include<stdio.h>
//presentation of structure without an alignment force
struct test1 {
unsigned int x: 5;
unsigned int y:8;
};
//presentation of structure with an alignment force
struct test2 {
unsigned int x:5;
unsigned int:0;
unsigned int y: 8;
};
int main ()
{
printf(“size of test1 is %lu bytes\n”, size of (struct test 1));
printf (“size of test2 is %lu bytes\n”, size of (struct test 2));
return 0;
}
Output:
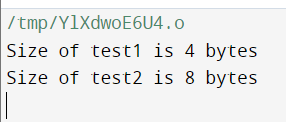
- As they do not start at byte boundary,we may not have pointers to bit field members:
Example:
#include<stdio.h>
struct test {
unsigned int x:5;
unsigned int y:5;
unsigned int z;
};
int main ()
{
struct test t;
//Leaving a line uncommented causes the programme to build and run.
printf (“address of t.x is %p”, &t.x);
//as z is not a member of bit field so below line works fine
printf (“address of t.z is %p”, &t. z);
return 0;
}
Output:

Bit field declaration
Structure of the bit field declaration:
struct {
type [member name] : width;
};
The below table shows the variable elements of bit field:
Sr. No | Elements & Description |
1. | Type: An int type that determines how bit fields clarify and the type may be unsigned or singed int. |
2. | Member _ name: Bit field name |
3. | Width: The bit width of specified type must be greater than or equal to Width and the width are bits number in bit field |
Bit fields can be defined as variables with predefined width, and it can hold more than one bit.
Example: For storing values from 0 to 7 variable is needed and we should define bit field with width 3
struct {
unsigned int age: 3;
} Age;
If we use more than 3 bits, then it will not be allowed.
Example:
#include<stdio.h>
#include<string.h>
struct {
unsigned int age: 3;
} Age;
int main () {
Age. Age=4;
printf (“size of (Age): %d\n”, size of (Age));
printf (“Age. age: %d\n”, Age. age);
Age.age=7;
printf (“Age. age: %d\n”, Age. age);
Age.age =8;
printf (“Age. age: %d\n”, Age. age);
return 0;
}
Output:
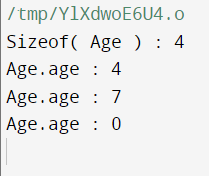