Dijkstra’s vs Bellman-Ford Algorithm
The Dijkstra Algorithm
One of the SSSP (Single Source Shortest Path) algorithms is Dijkstra's. As a result, it finds the shortest path between a source node and all other nodes in the graph.
Although Dijkstra's algorithm is known to work with weighted graphs, it does so with non-negative edge weights. We'll get to the bottom of why this is.
Theoretical Concept
We begin with a source node and set its distance to zero in Dijkstra's algorithm. The source node is then moved to a priority queue with a cost of zero.
After that, we go through a series of steps. We extract the node with the lowest cost in each step, update its neighbours' distances, and, if necessary, push them to the priority queue. Naturally, each of the neighbouring nodes is given a new cost, which is equal to the cost of the extracted node plus the cost of the edge we just passed through.
We'll keep going until there are no more nodes in the priority queue to extract. The calculated distances are then returned.
Dijkstra's algorithm has a complexity of O(V + E. log(V)), where V is the number of nodes in the graph and E is the number of edges
Proof of the concept
We always extract the node with the lowest cost in Dijkstra's algorithm. In the case of non-negative edges, we can show that this approach is correct.
Assume that the node with the lowest cost is u. Also, let's say we want to extract v, a node with a higher cost than u. To put it another way, we have:
Dist_u < Dist_v
If we extract v first, we won't be able to reach u at a lower cost. The reason for this is that v has a higher price tag. As a result, any path leading from v to u will have a cost equal to the cost of v plus the distance between v and u. To put it another way, we're attempting to demonstrate that:
Dist_u > Dist_v + Path(v, u)
Dist u, on the other hand, is smaller than Dist v, as we already know. The last equation can never be true because Path (u, v) has a non-negative weight. As a result, extracting the node with the lowest cost is always the best option.
Limitations
As a result, we proved that Dijkstra's algorithm is the best. However, we made the assumption that all of the edges have non-negative weights in order to do so. As a result, Path(u, v) will always be nonnegative.
Dijkstra's algorithm fails to calculate the shortest paths correctly when working with graphs with negative weights. The reason for this is that Path(u, v) could be negative, making it easier to get to u from v at a lower cost. As a result, we can't prove that choosing the node with the lowest cost is the best option.
The Benefits and Drawbacks of Dijkstra's algorithm
The main benefit of Dijkstra's algorithm is its near-linear complexity. Dijkstra's algorithm, however, cannot be used when working with negative weights.
Also, calculating the shortest path between any pair of nodes using Dijkstra's algorithm is not a good option when working with dense graphs where E is close to V2.
Dijkstra's time complexity is O(V + E . log(V)), so this is the case. The complexity is O(V + V2 . log(V)) because E is nearly V2.
We'll use Dijkstra's algorithm to find the shortest path between each pair of nodes in the graph. As a result, the overall complexity is O(V2 + V3 . log(V)).
The Floyd-Warshall algorithm, which has a time complexity of O(V3), can calculate the same thing, so this isn't a good enough complexity. As a result, it can achieve the same result with less effort.
The Bellman-Ford Algorithm
The Bellman-Ford algorithm, like Dijkstra's, is one of the SSSP algorithms. As a result, it finds the shortest path between a starting source node and all of the nodes in a weighted graph. The Bellman-Ford algorithm, on the other hand, is based on a different concept than Dijkstra's.
Theoretical Concept
In the Bellman-Ford algorithm, we start by setting all node distances to infinity, with the exception of the source node, which is set to zero. Then we go through the V - 1 steps.
We iterate over all of the graph's edges in each step. If necessary, we update the respective distances of v for each edge from node u to v. The new possible distance is equal to the distance between u and v plus the edge's weight.
We stop the algorithm after V - 1 steps, when all of the nodes have the correct distance.
The time complexity of the Bellman-Ford algorithm is O(V . E), where V is the number of vertices and E is the number of edges in the graph. We perform V steps, which explains the complexity. We visit all of the graph's edges in each step.
Proof of the concept
The Bellman-Ford algorithm assumes that all nodes will have correct distances after V - 1 steps. Let's see if this assumption is correct.
Any acyclic path within the graph can have a maximum of V nodes, implying that it has V - 1 edges. Because there are more than V nodes, a path with more than V - 1 edges is said to be a cycle. As a result, it must return to the same node multiple times.
Any shortest path will not go through cycles, we can guarantee. Otherwise, we might have been able to break the cycle and find a better path.
Returning to the Bellman-Ford algorithm, we can be confident that the algorithm will cover all possible shortest paths after V - 1 steps. As a result, the algorithm is guaranteed to produce an optimal result.
Limitations
As a result, we demonstrated that the Bellman-Ford algorithm provides the best solution to the SSSP problem. The first flaw in our argument is that going through a cycle can improve the shortest path!
When we have a cycle with a negative total sum of edges, this is the only case where this is correct. We usually can't calculate the shortest path in this case because we can always get a shorter path by iterating the cycle one more time. As a result, the term "shortest path" has no meaning.
Even if the graph has negative weights, our proof still holds as long as there are no negative cycles.
In fact, the Bellman-Ford algorithm can be used to check for the presence of negative cycles. We only need to save the distances we calculated after performing V - 1 steps as an update. Then, in the same manner as before, we perform one more step (number V).
After that, we look to see if there is a node with a better path. If that's the case, there must be at least one negative cycle causing this node to take a shorter path.
Undirected graphs are the subject of the second limitation. Although it is true that an undirected graph can always be transformed into a directed graph, Bellman-Ford fails to handle undirected graphs with negative weights.
If the edge between u and v has a negative weight in the Bellman-Ford algorithm, we now have a negative cycle. Going from u to v and back to v forms a cycle with a weight equal to twice the edge between u and v.
Advantages and Drawbacks
The Bellman-Ford algorithm's main advantage is its ability to handle negative weights. The Bellman-Ford algorithm, on the other hand, is significantly more complex than Dijkstra's. As a result, Dijkstra's algorithm has a wider range of applications, because graphs with negative weights are a rare occurrence.
The Bellman-Ford algorithm, as previously stated, can handle both directed and undirected graphs with non-negative weights. However, as long as there are no negative cycles, it can only handle directed graphs with negative weights.
We can also use the Bellman-Ford algorithm to check for negative cycles, as previously mentioned.
Bellman Ford's and Dijkstra's algorithms differ in the following ways:
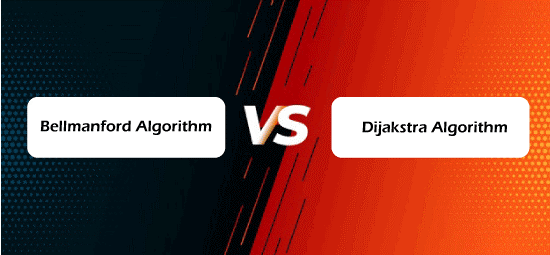
Both Bellman Ford's and Dijkstra's algorithms are single-source shortest path algorithms, which means they calculate the shortest distance between each vertex of a graph from a single source vertex. There are, however, some significant differences between them. In Bellman Ford's algorithm, we use the Dynamic Programming approach, and in Dijkstra's algorithm, we use the Greedy approach. Let's look at some of the other key differences between these two approaches. –
Bellman Ford’s Algorithm | Dijkstra's algorithm |
When there is a negative weight edge, Bellman Ford's Algorithm detects the negative weight cycle. | When there is a negative weight edge, Dijkstra's Algorithm may or may not work. However, it will not work if you are in a negative weight cycle. |
The vertices in the result contain information about the other vertices to which they are connected. | The vertices in the result contain all network information, not just the vertices to which they are connected. |
It is simple to implement in a distributed manner. | It is difficult to implement in a distributed manner. |
It takes longer to complete than Dijkstra's algorithm. It has an O time complexity (VE). | It takes less time to complete. O is the time complexity (E logV). |
The algorithm is implemented using a Dynamic Programming approach. | The algorithm is implemented in a greedy manner. |
As can be seen, Dijkstra's algorithm performs better in terms of reducing time complexity. When we have negative weights, however, we must use the Bellman-Ford algorithm. The Bellman-Ford algorithm can also be used to determine whether or not the graph contains negative cycles.
Just keep in mind that the Bellman-Ford algorithm can only help us with directed graphs in the case of negative weights or even negative cycles.