Recursion in Fibonacci
Fibonacci heap is considered to be a particular execution of the heap data structure that ultimately helps in making use of not just any number but the Fibonacci numbers. It is basically a group of trees that contains the min-heap and the max-heap traits. In this particular kind of heap, the trees can be of any given shape, they can even have single nodes all over.
The Fibonacci generally has a quicker bring-down run-time facility than the others. They are considered to be a lot likely in behavior with the binomial heap with the mere difference that they have a less tightly – packed structure. The binomial ones extract and merge the heap quickly whereas the Fibonacci ones wait until the extract – min function is called.
In this article, we are going to discuss the recursion in the Fibonacci heap. Recursion is basically a function that calls itself constantly until a condition is provided where it has to stop. We have to provide a base condition in order to stop the function from reoccurring; otherwise, the function will crash ultimately. A recursive function is generally used when the code is smaller as it becomes very complex when we have a lengthy or complex code.
The recursive function turns out to be very less efficient and useful when it comes to memory usage and implementation speed. In some cases, it turns out to be very slow in processing. A question might arise if this function is slow for the execution part then why do we use it, that’s because it is a very useful technique through which we can simply minimize the length of our code and make it more user-friendly as well as readable.
SYNTAX
int numb (int n)
{
if (n = = 0)
return 1;
else
return n * numb(n – 1);
}
STRUCTURE OF FIBONACCI
The Fibonacci numbers or digits are considered to be the ones in which each term in the series is a sum of the previous two numbers provided.
F1 = F2 = 1,
Fn = Fn-1 + Fn-2
The very first Fibonacci series to exist in nature is:-
1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89……………..
In this article, we are going to discuss the recursion in the Fibonacci heap.
ALGORITHM
In this section, we will see the steps which will tell us the working of recursion.
- START
- Insert an integer that is not negative in nature suppose, ‘ o ‘.
- Now in this step, lay out the condition which is:-
If (o = = 0 || o = = 1)
Return o;
Else
Return Fibonacci (o – 1) + Fibonacci(o – 2 );
- Print the oth Fibonacci number.
- END
IMPLEMENTATION
To understand the working of the recursion in Fibonacci, we first have to take the numbers of the same series as a medium of input from the user or client using the scanf function. For this, we are simply using a predefined function that will take the input from the user or client and return the Nth Fibonacci number using the recursion method as discussed above. This method will simply terminate if the number occurring is less than 3.
Here is the implementation of the same:-
#include < stdio.h >
#include < conio.h >
int Fibonacci ( int number );
int main ( ) {
int numbers, counter;
printf( “ Enter the numbers of the Fibonacci series: “ );
scanf( “ %d ”, &numbers);
/*Nth number = ( N – 1)th number + ( N – 2 )th number;
*/
Printf ( “Fibonacci series till %d number \n “, numbers );
For ( counter = 0; counter < numbers; counter + +) {
Printf( “ %d “, Fibonacci (counter));
}
getch ( );
return 0;
int Fibonacci (int number) {
if (number < 3)
return number;
return Fibonacci( number – 1) + Fibonacci(number – 2);
}
Output:
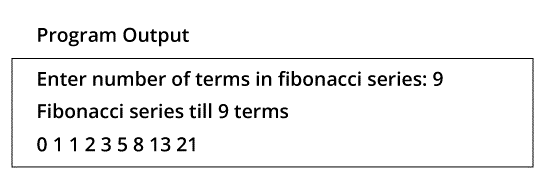
COMPLEXITY
In the recursion in Fibonacci, we can observe that each of the nodes generates two extra states and the total amount of states generated is 15. We know that the total number of states is equal to 2^n for the nth Fibonacci number. A fun fact is that each of the states gives rise to another Fibonacci function ( ) which does nothing rather than call itself. Hence, the total amount of time taken in order to calculate the nth number will be O(2^n).
SPACE COMPLEXITY
Space complexity is nothing but the total amount of memory or space that a specific algorithm or program takes at the time of the execution of that program. The space complexity of recursion in Fibonacci is said to be O(nm).