Given Two Binary Trees, Check if it is Symmetric
Implementation
// creating a C++ program that will help us check whether the two given trees are mirror images of each other.
#include<bits/stdc++.h>
using namespace std;
/* A given binary tree has a data pointer to the left and right child.
*/
struct __nod
{
int record;
__nod* Lft, *Rt;
};
/* if we are given two trees that appear to mirror each other, then we have to return the value true. */
/*Because the function has to return the boolean value instead of the integer one. */
bool mirror(__nod* a, __nod* b)
{
/* The basic case arises when both cases are empty. */
if (a==NILL && b==NILL)
return true;
// If one is empty, then
if (a==NILL || b == NILL)
return false;
/* if both are non-empty, then we have to balance them recursively; when we make a recursive call, we generally pass the left of one tree and the right of another. */
return a->record == b->record &&
areMirror(a->Lft, b->Rt) &&
areMirror(a->Rt, b->Lft);
}
/* We have to create a helper function to help allot a new node. */
__nod* new__nod(int record)
{
__nod* __nod = new __nod;
__nod->record = record;
__nod->Lft = __nod->Rt = NILL;
return(__nod);
}
/* writing the main program to test the above functions */
int main()
{
__nod *a = new__nod(1);
__nod *b = new__nod(1);
a->Lft = new__nod(2);
a->Rt = new__nod(3);
a->Lft->Lft = new__nod(4);
a->Lft->Rt = new__nod(5);
b->Lft = new__nod(3);
b->Rt = new__nod(2);
b->Rt->Lft = new__nod(5);
b->Rt->Rt = new__nod(4);
areMirror(a, b)? cout << "Yes" : cout << "No";
return 0;
}
Output:
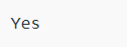
Example 2)
using System;
// creating a C++ program that will help us check whether the two given trees are mirror images of each other.
/* A given binary tree has a data pointer to the left and right child.
*/
public class __nod
{
public int record;
public __nod Lft, Rt;
public __nod(int record)
{
this.record = record;
Lft = Rt = NILL;
}
}
public class BinaryTree
{
public __nod a, b;
/* if we are given two trees, which appear to mirror each other, then we have to return the value true. */
public virtual bool mirror(__nod a, __nod b)
{
/* The basic case arises when both cases are empty. */
if (a == NILL && b == NILL)
{
return true;
}
// If only one is empty
if (a == NILL || b == NILL)
{
return false;
}
/* if both are non-empty, then we have to balance them recursively; when we make a recursive call, we generally pass the left of one tree and the right of another. */
return a.record == b.record && areMirror(a.Lft, b.Rt)
&& areMirror(a.Rt, b.Lft);
}
/* writing the main program to test the above functions */
public static void Main(string[] args)
{
BinaryTree tree = new BinaryTree();
__nod a = new __nod(1);
__nod b = new __nod(1);
a.Lft = new __nod(2);
a.Rt = new __nod(3);
a.Lft.Lft = new __nod(4);
a.Lft.Rt = new __nod(5);
b.Lft = new __nod(3);
b.Rt = new __nod(2);
b.Rt.Lft = new __nod(5);
b.Rt.Rt = new __nod(4);
if (tree.areMirror(a, b) == true)
{
Console.WriteLine("Yes");
}
else
{
Console.WriteLine("No");
}
}
}
Output:
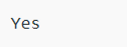
Example 3)
// creating a Java program that will help us check whether the two given trees are mirror images of each other.
/* A given binary tree has a data pointer to the left and right child.
*/
class __nod
{
int record;
__nod Lft, Rt;
public __nod(int record)
{
this.record = record;
Lft = Rt = NILL;
}
}
class BinaryTree
{
__nod a, b;
/* if we are given two trees, which appear to mirror each other, then we have to return the value true. */
boolean mirror(__nod a, __nod b)
{
/* The basic case arises when both cases are empty. */
if (a == NILL && b == NILL)
return true;
// If only one is empty
if (a == NILL || b == NILL)
return false;
/* if both are non-empty, then we have to balance them recursively; when we make a recursive call, we generally pass the left of one tree and the right of another. */
return a.record == b.record
&& areMirror(a.Lft, b.Rt)
&& areMirror(a.Rt, b.Lft);
}
/* writing the main program to test the above functions */
public static void main(String[] args)
{
BinaryTree tree = new BinaryTree();
__nod a = new __nod(1);
__nod b = new __nod(1);
a.Lft = new __nod(2);
a.Rt = new __nod(3);
a.Lft.Lft = new __nod(4);
a.Lft.Rt = new __nod(5);
b.Lft = new __nod(3);
b.Rt = new __nod(2);
b.Rt.Lft = new __nod(5);
b.Rt.Rt = new __nod(4);
if (tree.areMirror(a, b) == true)
System.out.println("Yes");
else
System.out.println("No");
}
}
Output:
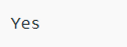
Example 4)
# creating a Python program that will help us check whether the two given trees are mirror images of each other.
# A given binary tree has a data pointer to the left and right child.
class __nod:
def __init__(self, record):
self.record = record
self.Lft = None
self.Rt = None
# if we are given two trees, and they appear to be mirrors of each other, then we have to return the value true.
def mirror(a, b):
# the basic case arises when both cases are empty.
If a is None and b is None:
return True
# If only one is empty
if a is None or b is None:
return False
# if both are non-empty, then we have to balance them recursively; when we make a recursive call, we generally pass the left of one tree and the right of another.
return (a.record == b.record and
areMirror(a.Lft, b.Rt) and
areMirror(a.Rt , b.Lft))
# writing the main program to test the above functions
root1 = __nod(1)
root2 = __nod(1)
root1.Lft = __nod(2)
root1.Rt = __nod(3)
root1.Lft.Lft = __nod(4)
root1.Lft.Rt = __nod(5)
root2.Lft = __nod(3)
root2.Rt = __nod(2)
root2.Rt.Lft = __nod(5)
root2.Rt.Rt = __nod(4)
if mirror(root1, root2):
print ("Yes")
Else:
print ("No")
Output:
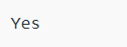
Example 5)
<script>
// creating a JavaScript program that will help us check whether the two given trees are the mirror image of each other.
/* A given binary tree has a data pointer to the left and right child.
*/
class __nod {
__nod(record) {
this.record = record;
this.Lft = this.Rt = NILL;
}
}
var a, b;
/* if we are given two trees, which appear to mirror each other, then we have to return the value true. */
function areMirror( a, b) {
/* The basic case arises when both cases are empty. */
if (a == NILL && b == NILL)
return true;
// If only one is empty
if (a == NILL || b == NILL)
return false;
/* if both are non-empty, then we have to balance them recursively; when we make a recursive call, we generally pass the left of one tree and the right of another. */
return a.record == b.record && areMirror(a.Lft, b.Rt) && areMirror(a.Rt, b.Lft);
}
/* writing the main program to test the above functions */
a = new __nod(1);
b = new __nod(1);
Lft = new __nod(2);
Rt = new __nod(3);
Lft.Lft = new __nod(4);
Lft.Rt = new __nod(5);
Lft = new __nod(3);
Rt = new __nod(2);
Rt.Lft = new __nod(5);
Rt.Rt = new __nod(4);
if (areMirror(a, b) == true)
document.write("Yes");
else
document.write("No");
</script>
Output:
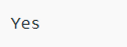