What is B tree?
What do you mean by B Tree in Data Structures?
In the technological world, a B tree is simply a well-managed and coordinated tree and an integral part of the data structure world that basically help us prepare ourselves and keep our data preserved and that too in a systematic manner. It generally keeps track of the sorted and organized data and gives permission for various operations such as insertion, deletion, and several others. The B tree is the way to maintain the binary search tree that gives space for the nodes to engage and have two children.
B tree stands united and indestructible as compared to the rest of the trees because they have the characteristic of maintaining the storage systems that will particularly help us in reading and rewriting a lot of files such as the files present in the database and file systems. The B tree is a uniquely special kind of tree that is designed in an m – way. It is popularly used for its participation in disk access. If we have a B tree that has the order m then it is pretty clear that the tree can have m number of keys and m amount of children.
The biggest advantage that we come across while using or implementing the B tree is its massive ability to occupy the enormous number of keys and that too in a single node and also by this, we keep a check that the height of the given tree is comparatively small. There are numerous operations that we can perform while using the B tree in order to maximize its use such as searching, deletion, insertion traversal, and several others.
Algorithm
In this section, we will be seeing the steps through which we can use a B tree in data structures.
BtreeSearch(x, k)
j = 1
while j = n[q] and k ≥ keyi[q] // n[q] means number of keys in q node
do j = j + 1
if j n[q] and k = keyi[q]
then return (q, j)
if leaf [q]
then return NULL
else
return BtreeSearch(cj[q], k)
Application of B tree
- The B tree in the data structure is specifically used to create the index and layout quick access that will particularly be kept safe in an input device e.g., disk.
- Searching for information and data becomes very difficult and cumbersome when it comes to an unorganized set of data, but this can be changed and set to a lot better version by using the indexing system.
- B tree is extensively known for its application in the world of databases and file systems.
- Many servers that we come across also use this kind of approach in order to facilitate work.
- We can also perform multilevel indexing by making use of the indexing attribute of the B tree and it will prove to be very beneficial for our work.
- The B tree is best known for its managing skills as it uses partially occupied containers that eventually lead to maximized applications of various operations such as insertion and deletion.
- B tree is famous for many tasks, but it is especially well known for its application in managing and keeping various keys in a systematic order.
Implementation
// Searching a key on a B-tree in C++
#include <iostream>
using namespace std;
class TreeNode {
int *keys;
int t;
TreeNode **C;
int n;
bool leaf;
public:
TreeNode(int temp, bool bool_leaf);
void insertNonFull(int k);
void splitChild(int i, TreeNode *y);
void traverse();
TreeNode *search(int k);
friend class BTree;
};
class BTree {
TreeNode *root;
int t;
public:
BTree(int temp) {
root = NULL;
t = temp;
}
void traverse() {
if (root != NULL)
root->traverse();
}
TreeNode *search(int k) {
return (root == NULL) ? NULL : root->search(k);
}
void insert(int k);
};
TreeNode::TreeNode(int t1, bool leaf1) {
t = t1;
leaf = leaf1;
keys = new int[2 * t - 1];
C = new TreeNode *[2 * t];
n = 0;
}
void TreeNode::traverse() {
int i;
for (i = 0; i < n; i++) {
if (leaf == false)
C[i]->traverse();
cout << " " << keys[i];
}
if (leaf == false)
C[i]->traverse();
}
TreeNode *TreeNode::search(int k) {
int i = 0;
while (i < n && k > keys[i])
i++;
if (keys[i] == k)
return this;
if (leaf == true)
return NULL;
return C[i]->search(k);
}
void BTree::insert(int k) {
if (root == NULL) {
root = new TreeNode(t, true);
root->keys[0] = k;
root->n = 1;
} else {
if (root->n == 2 * t - 1) {
TreeNode *s = new TreeNode(t, false);
s->C[0] = root;
s->splitChild(0, root);
int i = 0;
if (s->keys[0] < k)
i++;
s->C[i]->insertNonFull(k);
root = s;
} else
root->insertNonFull(k);
}
}
void TreeNode::insertNonFull(int k) {
int i = n - 1;
if (leaf == true) {
while (i >= 0 && keys[i] > k) {
keys[i + 1] = keys[i];
i--;
}
keys[i + 1] = k;
n = n + 1;
} else {
while (i >= 0 && keys[i] > k)
i--;
if (C[i + 1]->n == 2 * t - 1) {
splitChild(i + 1, C[i + 1]);
if (keys[i + 1] < k)
i++;
}
C[i + 1]->insertNonFull(k);
}
}
void TreeNode::splitChild(int i, TreeNode *y) {
TreeNode *z = new TreeNode(y->t, y->leaf);
z->n = t - 1;
for (int j = 0; j < t - 1; j++)
z->keys[j] = y->keys[j + t];
if (y->leaf == false) {
for (int j = 0; j < t; j++)
z->C[j] = y->C[j + t];
}
y->n = t - 1;
for (int j = n; j >= i + 1; j--)
C[j + 1] = C[j];
C[i + 1] = z;
for (int j = n - 1; j >= i; j--)
keys[j + 1] = keys[j];
keys[i] = y->keys[t - 1];
n = n + 1;
}
int main() {
BTree t(3);
t.insert(8);
t.insert(9);
t.insert(10);
t.insert(11);
t.insert(15);
t.insert(16);
t.insert(17);
t.insert(18);
t.insert(20);
t.insert(23);
cout << "The B-tree is: ";
t.traverse();
int k = 10;
(t.search(k) != NULL) ? cout << endl
<< k << " is found"
: cout << endl
<< k << " is not Found";
k = 2;
(t.search(k) != NULL) ? cout << endl
<< k << " is found"
: cout << endl
<< k << " is not Found\n";
}
Output:
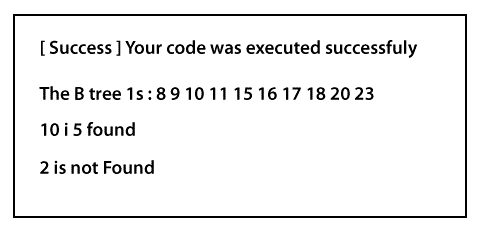
Height in terms of the best and worst case
Let us suppose the height of a given basic B tree is h and that h ≥ - 1. After this, we see that the number of data present in the tree is n and let that n ≥ 0. Let us suppose that the total or highest number of nodes is children that can have to be x then, each one of the nodes will be having x – 1 key. It is pretty clear from the tree that if we have a B tree whose height is h that has a situation where all its nodes are completely occupied and complete. In that case n = x h + 1 data. Therefore, in this scenario the best-case height of a B tree will be:
hmin = [ logx ( n + 1 ) ] – 1
Let us suppose that y will the least number of nodes that will be in an integral node should have and in that case, the situation for a basic B tree will be y = [ x/2 ].
Here the height of a basic B tree in the worst possible case will be:
hmax = [ logy n + 1 / 2].
Complexity
In this section of the article, we are going to discuss the complexity of the B tree in the best, worst and average cases. We will also encounter the space complexity of the same in this section.
Case | Time |
Best case | O(log n) |
Average case | O(n) |
Worst case | O(log n) |
- BEST CASE
In B tree, it usually takes place when we have quick access to the element we are searching and we get towards it faster and we don’t have to perform a rotation to do the same. So, the complexity for the best case of the B tree is O(log n). - AVERAGE CASE
The average case usually occurs when we have to take out the mean of all the possible scenarios which is the sum of all the case / total number of cases. In this case, the time complexity turns out to be O(n). - WORST CASE
The worst-case complexity occurs when the element we have to search is present at the end or suppose isn’t present in the tree or if we have to take part in rotation then we know we have to at least make two rotations and for this, it will consume a lot of time. The complexity of the B tree in the worst case turns out to be O(log n).
Space Complexity
Space complexity is nothing but the total amount of memory or space that a specific algorithm or program takes at the time of the execution of that program. The space complexity of the B tree is said to be O(n).