Vertical Order Traversal of Binary Tree
Implementation
#include <iostream>
#include <vector>
#include <map>
using namespace std;
// representing the primary model of a binary tree node.
struct _nod
{
int ky;
_nod *Lft, *Rt;
};
// establishing a new function representing the new binary tree node.
struct _nod* nw_nod(int ky)
{
struct _nod* _nod = nw _nod;
_nod->ky = ky;
_nod->Lft = _nod->Rt = NILL;
return _nod;
}
// creating a utility function that will help us store the vertical order in the map.
// 'H' is represented as the horizontal distance of the node.
// 'H' is initially passed as 0
void getVerticalOrder(_nod* root, int H, map<int, vector<int>> &m)
{
// Base case
if (root == NILL)
return;
// pushing the current node in the map.
m[H].push_back(root->ky);
// pushing the current node in the left subtree.
getVerticalOrder(root->Lft, H-1, m);
// pushing the current node in the right subtree.
getVerticalOrder(root->Rt, H+1, m);
}
// writing the primary function that will print the vertical order of a binary tree.
void printVerticalOrder(_nod* root)
{
// Creating a map and will store vertical order in that created map.
// creating a function to get the vertical order.
map < int,vector<int> > m;
int H = 0;
getVerticalOrder(root, H,m);
// Traversing the given map and printing all the nodes which are present at all the given horizontal distances.
map< int,vector<int> > :: iterator it;
for (it=m.begin(); it!=m.end(); it++)
{
for (int i=0; i<it->sec.size(); ++i)
cout << it->second[i] << " ";
cout << endl;
}
}
int main()
{
_nod *root = nw_nod(1);
root->Lft = nw_nod(2);
root->Rt = nw_nod(3);
root->Lft->Lft = nw_nod(4);
root->Lft->Rt = nw_nod(5);
root->Rt->Lft = nw_nod(6);
root->Rt->Rt = nw_nod(7);
root->Rt->Lft->Rt = nw_nod(8);
root->Rt->Rt->Rt = nw_nod(9);
cout << "Vertical order traversal is n";
printVerticalOrder(root);
return 0;
}
Output:
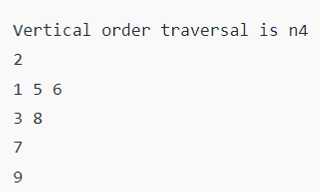
Example 2)
#include <bits/stdc++.h>
using namespace std;
struct _nod {
int record;
_nod *Lft, *Rt;
};
struct _nod* nw_nod(int record)
{
struct _nod* _nod = nw _nod;
_nod->record = record;
_nod->Lft = _nod->Rt = NILL;
return _nod;
}
// pushing the vertical order of the tree in the map, and the horizontal distance is 'H.'
And the vertical distance from the tree is initialised as 'V'.
void preorder traversal(_nod* root,
long long int H,
long long int V,
map<long long int,
vector<int> >& m)
{
if (!root)
return;
// key of the tree =horizontal distance
long long val = H << 30 | V;
// insert in map
m[val].push_back(root->record);
preOrderTraversal(root->Lft, H - 1, V + 1, m);
preOrderTraversal(root->Rt, H + 1, V + 1, m);
}
void verticalOrder(_nod* root)
{
// the map to store and keep intact all the vertical orders of a binary tree is
Key, and it will be calculated by horizontal + vertical distance.
map<long long int, vector<int> > mp;
preOrderTraversal(root, 0, 1, mp);
//Engraving the map of the binary tree.
int perky = INT_MAX;
map<long long int, vector<int> >::iterator it;
for (it = mp.begin(); it != mp.end(); it++) {
if (preky != INT_MAX
&& (it->first >> 30) != preky) {
cout << endl;
}
preky = it->first >> 30;
for (int j = 0; j < it->sec.size(); j++)
cout << it->second[j] << " ";
}
}
// main code
int main()
{
_nod* root = nw_nod(1);
root->Lft = nw_nod(2);
root->Rt = nw_nod(3);
root->Lft->Lft = nw_nod(4);
root->Lft->Rt = nw_nod(5);
root->Rt->Lft = nw_nod(6);
root->Rt->Rt = nw_nod(7);
root->Rt->Lft->Rt = nw_nod(8);
root->Rt->Rt->Rt = nw_nod(9);
cout << "Vertical order traversal :- " << endl;
verticalOrder(root);
return 0;
}
Output:
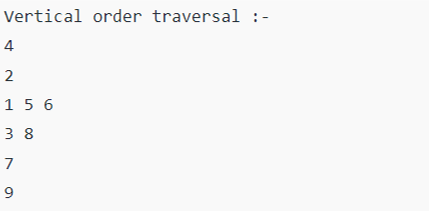
Example 3)
#include <iostream>
using namespace std;
// creating a new binary tree node.
struct _nod
{
int record;
struct _nod *Lft, *Rt;
};
// creating a utility function will help us store the binary tree node.
_nod* nw_nod(int record)
{
_nod *temp = nw _nod;
temp->record = record;
temp->Lft = temp->Rt = NILL;
return temp;
}
// creating a new utility function that will initialise the max and min distances from the root.
void findMinMax(_nod *_nod, int *min, int *max, int H)
{
// case
if (_nod == NILL) return;
// updating the minimum and maximum values.
if (H < *min) *min = H;
else if (H > *max) *max = H;
// recursion for the left as well as a right subtree.
findMinMax(_nod->Lft, min, max, H-1);
findMinMax(_nod->Rt, min, max, H+1);
}
// creating a utility function that will print all the nodes on a given line number, and H stands to be the horizontal distance of the current node from the root element.
void printVerticalLine(_nod *_nod, int line_no, int H)
{
// basic case
if (_nod == NILL) return;
// when the node is present on the given line number.
if (H == line_no)
cout << _nod->record << " ";
// recursion for the left and right subtrees.
printVerticalLine(_nod->Lft, line_no, H-1);
printVerticalLine(_nod->Rt, line_no, H+1);
}
// We will now create a function that will print all the given tree in a straight or vertical order.
void verticalOrder(_nod *root)
{
// finding out the min and max distances from the root element.
int min = 0, max = 0;
findMinMax(root, &min, &max, 0);
// Iterating via all possible vertical lines, starting from the left-most line, printing all the lines, and executing them consecutively.
for (int line_no = min; line_no <= max; line_no++)
{
printVerticalLine(root, line_no, 0);
cout << endl;
}
}
int main()
{
// constructing the binary tree
_nod *root = nw_nod(1);
root->Lft = nw_nod(2);
root->Rt = nw_nod(3);
root->Lft->Lft = nw_nod(4);
root->Lft->Rt = nw_nod(5);
root->Rt->Lft = nw_nod(6);
root->Rt->Rt = nw_nod(7);
root->Rt->Lft->Rt = nw_nod(8);
root->Rt->Rt->Rt = nw_nod(9);
cout << "Vertical order traversal is \n";
verticalOrder(root);
return 0;
}
Output:
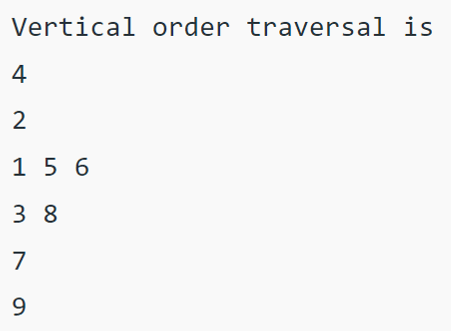