Kth Ancestor Node of Binary Tree
Kth ancestors of a node of binary tree are the nodes which start from the root node of binary tree and which we get by following below steps:
- Traverse the tree starting from the root node, and create a list of all the nodes that are encountered along the way.
- Find the position of the target node in the list.
- If the position of the target node is greater than or equal to k, return the (position - k) element in the list.
- If the position of the target node is less than k, return null, as the target node does not have a kth ancestor.
The kth ancestor of a node in a binary tree is the (k+1) parent of the node, with the parent of the node being the 1st ancestor. For example, if k=1, the 1st ancestor of a node is its parent, and if k=2, the 2nd ancestor of a node is its grandparent. If a node does not have a kth ancestor (because the node is the root of the tree or because the kth ancestor does not exist), then the kth ancestor of the node is considered to be null. In general, the kth ancestor of a node in a binary tree can be found by starting at the root of the tree and traversing the tree until the target node is encountered. If the target node is found, the kth ancestor can be found by going up the tree k levels from the target node. If the target node is not found or if the kth ancestor does not exist, then the kth ancestor is considered to be null.
The concept of the kth ancestor of a node in a binary tree is a way to refer to a specific ancestor of a node, where the ancestor is defined as a parent, grandparent, great-grandparent, and so on. The kth ancestor of a node is the (k+1) parent of the node, with the parent of the node being the 1st ancestor.
For example, consider the following binary tree as for the kth node as:
1
/ \
2 3
/ \ / \
4 5 6 7
In this tree, the 1st ancestor of node 4 is node 2, the 2nd ancestor of node 4 is node 1, and the 3rd ancestor of node 4 is null, since node 4 does not have a 3rd ancestor.
To find the kth ancestor of a node in a binary tree, you can traverse the tree starting from the root node and create a list of all the nodes that are encountered along the way. Then, you can find the position of the target node in the list, and if the position is greater than or equal to k, return the (position - k) element in the list. If the position of the target node is less than k, then the target node does not have a kth ancestor and you can return null.
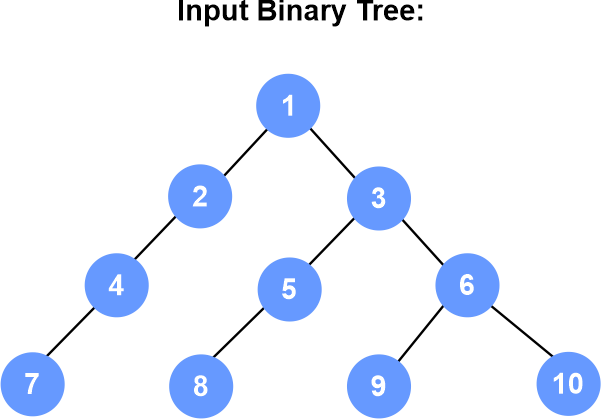
Use of the Kth node of Binary Tree
The kth ancestor of a node in a binary tree can be useful in a variety of applications. Here are a few examples of how it could be used:
- Navigation: The kth ancestor of a node can be used to navigate up the tree from a specific node. For example, if you want to move up the tree from a given node by k levels, you can use the kth ancestor function to find the node that is k levels above the current node. The navigation of the node of the tree can be done from any up of node from root node to leaf node of the binary tree of data structure.
- Data analysis: The kth ancestor of a node can be used to analyze the relationship between nodes in a tree. For example, you might use the kth ancestor function to find the common ancestor of two nodes, or to find the lowest common ancestor of a group of nodes. The exact number of relationships between various nodes of binary tree can be evaluated in this.
- Data structure design: The kth ancestor of a node can be used as a building block for more complex data structures, such as tree-based data structures that support efficient queries and updates. Overall, the kth ancestor of a node in a binary tree is a useful tool for navigating and analyzing tree-structured data.
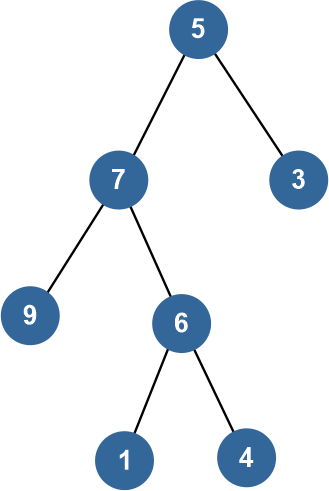