How to get Better in Data Structures and Algorithms?
Introduction
Data structures and algorithms are fundamental computer science concepts that store, organize, and process data efficiently. By understanding different data structures and algorithms and using them effectively, you can become a better programmer and solve complex problems more efficiently.
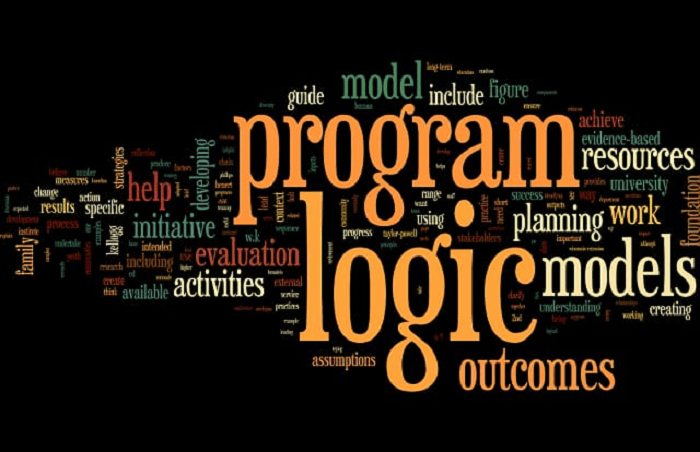
Developing a solid foundation in data structures and algorithms can also help to prepare for technical interviews and exams, as these topics are commonly covered.
Many resources, including online tutorials, lectures, and books, can help you learn about data structures and algorithms. Practising solving problems and implementing algorithms and data structures on your own is also a helpful way to improve your skills.
By staying up-to-date on new developments and techniques in the field and seeking additional learning opportunities, you can improve your skills and become proficient in data structures and algorithms.
What are Data Structures?
A data structure organizes and stores data in a computer to be accessed and modified efficiently. Different types of data structures are suited to other applications, and some are highly specialized for specific tasks.
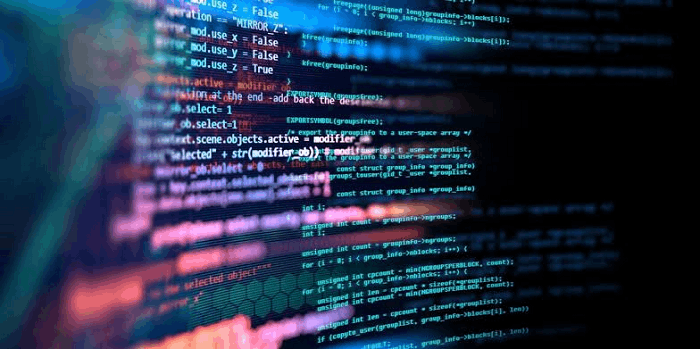
A data structure can be defined as a collection of data values, their relationships, and the functions or operations that can be applied to them. Data structures can be designed to store data efficiently and to support specific operations on that data.
There are many factors to consider when choosing a data structure for a given problem, including data size, the operations that need to be performed, and the efficiency of the data structure for operations.
Data structures are fundamental in computer science and are used in many areas, including software engineering, operating systems, and databases. By understanding different data structures and how to design and implement them, you can become proficient in solving a wide range of problems in computer science.
Several data structures are commonly used in computer science, each with its characteristics and uses. Here is a more detailed description of some common data structures:
- Array: In the world of data structures, arrays are a type of linear data structure. They store the data in a fixed-size sequential collection of elements of the same kind. Arrays are implemented in most programming languages and can store various data types, including integers, floating-point numbers, and strings. Arrays efficiently access and modify elements, as you can constantly access or modify an element at a specific index. However, they have a fixed size and are not better suited for inserting or deleting elements in the middle of the array, as this requires shifting all the elements after the insertion or deletion point.
- Linked List: A linked list is a data structure consisting of a sequence of nodes, where each node stores a value and a reference to the next node. Linked lists are flexible in size, as you can add or remove nodes as needed. However, they are slower to access elements and require more memory because each node needs to store a pointer to the next node. There are several linked lists, including singly linked lists, which have a reference to the next node but not to the previous node, and doubly linked lists, which have connections to both the next and previous nodes.
Linked lists help insert and delete elements, as you can do so constantly by updating the adjacent nodes' pointers. However, they are slower for accessing and modifying elements, as you must traverse the list to find the element you want to access or modify. - Stack: Stacks are linear data structures that store data in a Last In, First Out (LIFO) order. It has two main operations: push, which adds an element to the stack, and pop, which removes the top element from the stack. Stacks are often used for storing temporary data or implementing recursive algorithms, allowing you to store and retrieve data in a last-in, first-out order. Stacks are efficient for inserting and deleting elements, as these operations are constant-time. Still, they need to be better suited for accessing or modifying elements, as you can only access or alter the top element of the stack.
- Queue: A linear data structure that stores data in a First In, First Out (FIFO) order. It has two main operations: enqueue, which adds an element to the end of the queue, and dequeue, which removes the front element from the queue. Queues are often used to store data that needs to be processed in a specific order, as they allow you to store and retrieve data in a first-in, first-out order. Queues are efficient for inserting and deleting elements, as these operations are of constant-time. Still, they need to be better suited for accessing or modifying elements, as you cannot access or modify elements in the middle of the queue.
- Tree: A tree is one of the most popular non-linear data structures available today, which consists of a set of nodes organized in a hierarchy. An individual node in a tree has at least one child node, and all nodes except the root node have a parent node. Trees are often used to represent hierarchical relationships or to store data in sorted order. Some standard trees include binary trees, which have at most two children per node, and n-ary trees, which have at most n children per node. Who can traverse trees in different ways, such as in-order, pre-order, or post-order, and they can be searched using depth-first or breadth-first search techniques.
- Graph: Graphs are non-linear data structures with nodes and edges connecting them. What can use graphs to represent relationships between objects or to model networks? Graphs can be directed or undirected, depending on whether the edges have a direction. They can be weighted or unweighted, depending on whether the edges have a value associated with them—several algorithms for traversing and searching graphs, including depth-first and breadth-first searches.
- Hash table: A hash table can be considered as a data structure that maps keys to values using a hash function. Hash tables are used for fast lookups, insertions, and deletions, and they are particularly efficient when the keys are distributed uniformly. However, they can suffer from collisions, where two keys map to the same table position, leading to slower performance. Hash tables are implemented using an array and a hash function, and they support constant-time operations for inserting, deleting, and looking up elements.
What is an Algorithm?
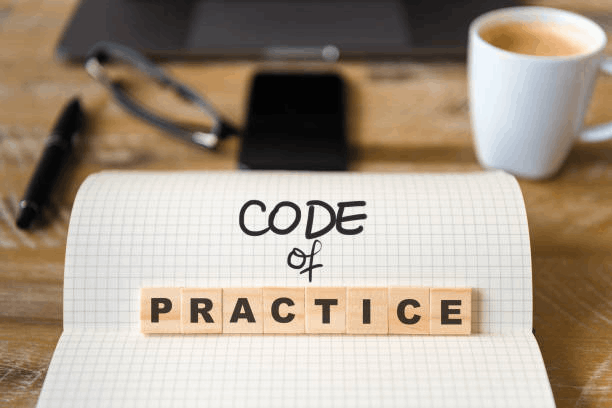
The term "algorithm" refers to a set of well-defined instructions or steps followed in a specific order to solve a problem or perform a task. It is a step-by-step procedure that takes in input data, processes it, and produces an output. Algorithms are essential in computer science and are used to design efficient and reliable software systems.
An algorithm typically consists of the following elements:
- Input: The input to an algorithm is the data that it operates. It can include simple data types, such as integers or floating-point numbers, and more complex data structures, such as arrays and linked lists.
- Output: The output of an algorithm is the result that it produces based on the input data. It can be a single value, such as the sum of a set of numbers, or a more complex data structure, such as a sorted list or a graph.
- Steps or operations: The steps or operations of an algorithm are the specific actions performed on the input data to produce the output. These can include comparisons, calculations, and data manipulations, typically ordered within a particular sequence.
- Termination: An algorithm is said to terminate when it has completed all of its steps and produced the desired output.
The efficiency of an algorithm refers to how quickly it completes its task and how much resources it consumes, such as time and memory. Some algorithms are more efficient than others for solving the same problem, and the choice of which algorithm to use can depend on factors such as the size of the input data and the desired performance and accuracy of the solution.
Types of Algorithms
Many different types of algorithms are used in computer science. They can be classified based on various criteria, such as their performance, complexity, and the problem they are used to solve. Here are some common types of algorithms:
- Sorting algorithms: Sorting algorithms rearrange items into a specific order, such as ascending or descending. Some standard sorting algorithms include bubble sort, insertion sort, selection sort, merge sort, and quick sort.
- Searching algorithms: It is used to find a specific item in a set of items. Some standard searching algorithms include linear search, binary search, and depth-first search.
- Graph algorithms: Graph algorithms are used to solve problems on graphs, which are data structures that consist of a set of nodes and edges connecting them. Some standard graph algorithms include breadth-first search, depth-first search, and shortest path algorithms such as Dijkstra's algorithm and A*.
- Divide and conquer algorithms: It is used to solve problems by dividing them into smaller subproblems, solving the subproblems, and then combining the solutions to the subproblems to get the answer to the original problem. Some examples of divide and conquer algorithms include merge sort and quick sort.
- Dynamic Programming algorithms: They solve optimization problems by breaking them into smaller subproblems and storing the solutions in a table to avoid recomputing them. Some standard dynamic programming algorithms include the knapsack problem and the travelling salesman problem.
- Greedy algorithms: Greedy algorithms solve optimization problems by making the locally optimal choice at each step and hoping that these choices lead to a globally optimal solution. Some examples of greedy algorithms include the Huffman coding algorithm and Dijkstra's algorithm for finding the shortest path in a graph.
10 Ways to Get Better in Data Structures and Algorithms?
Improving your skills in data structures and algorithms is an essential part of becoming a proficient computer programmer. Here are some suggestions for how to get better in these areas:
1. Practice, Practice, Practice!
Practising data structures and algorithms is critical to improving your skills and understanding of the material. When you practice, you can apply what you have learned to solve real problems, which can help you consolidate your knowledge and identify areas where you need to improve.
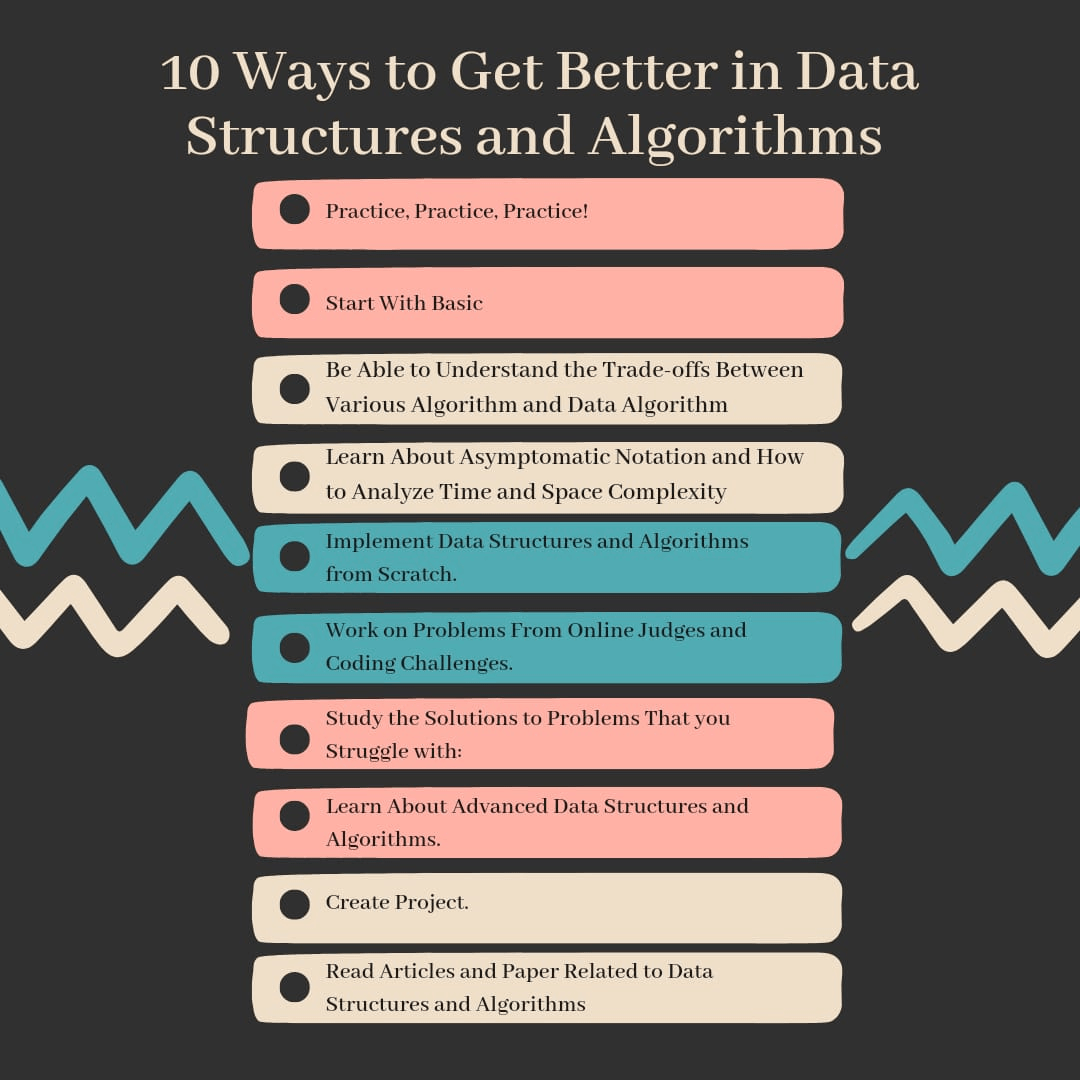
There are many ways you can practice data structures and algorithms, including:
- Solve practice problems: One of the best ways to practice is to solve problems on your own or with others. Many online resources provide practice problems and challenges, such as coding websites and online judges.
- Write code to implement: Another way to practice is to write code to implement data structures and algorithms. It will help you gain a deeper understanding of how they work and how to use them effectively.
- Participate in programming competitions: Programming competitions, such as hackathons and coding challenges, are a great way to practice solving problems under time pressure and to learn from other participants.
- Work on projects that require data structures and algorithms: Another way to practice is to work on projects that require data structures and algorithms. It can help you apply your knowledge to real-world scenarios and gain experience using them in a practical context.
- Review and analyse the solutions to problems: After you have solved a problem, it is helpful to review your answer and think about how you could have approached it differently or more efficiently. It will help you learn from your mistakes and improve your problem-solving skills.
Practice with a focus on specific topics or techniques: To focus on particular topics or Techniques, you can try to solve problems that require using those topics or techniques. For example, you can practice solving problems requiring specific data structures or algorithms or implementing specific algorithms from scratch.
You can improve your skills by consistently practising data structures and algorithms and becoming more proficient in solving complex problems.
2. Start With the Basics:
Starting with the basics is an excellent way to improve data structures and algorithms. The following steps can help you:
- Learn the fundamental concepts: Learning the fundamental concepts of data structures and algorithms is essential before you start solving problems. It includes understanding the basics of data structures such as arrays, linked lists, stacks, and queues and algorithms such as sorting and searching.
- Practice solving simple problems: Once you have a basic understanding of the concepts, you can start practising by solving simple problems. It will help you get a feel for problem-solving and build confidence.
- Gradually increase the difficulty of the problems: As you become more comfortable with the basics, you can gradually increase the plight of the problems you solve. It will help you build your skills and improve your problem-solving abilities.
- Focus on specific topics or techniques: To improve your skills in a specific area, you can focus your Practice on particular topics or techniques. For example, you can practice solving problems requiring specific data structures or algorithms or implementing specific algorithms from scratch.
- Review and analyze your solutions: After you have solved a problem, it is helpful to review your solution and think about how you could have approached it differently or more efficiently. It will help you learn from your mistakes and improve your problem-solving skills.
Remember to be patient and consistent in your Practice, and keep going even if you struggle with specific problems. A systematic approach will allow you to improve your skills in data structures and algorithms, but with dedication and persistence, you can make progress.
3. Be Able to Understand the Trade-Offs Between Various Algorithms and Data Structures:
Different data structures and algorithms have additional time and space complexities, and it's essential to understand the trade-offs between them so you can choose the right one for a given problem.
Understanding the trade-offs between different data structures and algorithms is essential in improving your skills. The following suggestions will help you accomplish this:
- Learn about the different characteristics of each data structure or algorithm: understand the trade-offs; it is helpful to learn about the other attributes of each data structure or algorithm. It can include information such as the time and space complexity, the performance characteristics, and the specific applications for which it best suits.
- Experiment with different data structures and algorithms: To gain a deeper understanding of the trade-offs, you can try experimenting with other data structures and algorithms. For example, you can try implementing different algorithms to solve the same problem and compare the results.
- Analyze the time and space complexity of different data structures and algorithms: To understand the trade-offs in efficiency, you can analyze the time and space complexity of other data structures and algorithms. It will help you understand the relative performance of each one and choose the most efficient solution for a given problem.
- Seek resources and guidance: Many resources can help you understand the trade-offs between data structures and algorithms. These can include books, articles, online courses, and advice from more experienced programmers.
Remember that the trade-offs between different data structures and algorithms can depend on the specific problem you are trying to solve and the desired performance and accuracy of the solution. By understanding these trade-offs, Who will better equip you to choose the proper data structure or algorithm for a given problem?
4. Learn About Asymptotic Notation and How to Analyze Algorithms' Time and Space Complexity:
It will help you understand the performance of different algorithms and how they scale as the input size increases.
Asymptotic notation is a mathematical tool used to describe the behaviour of algorithms as their input size grows to infinity. It allows us to compare the efficiency of different algorithms and predict the performance of an algorithm on massive inputs.
Asymptotic notation can be divided into three types:
- Big O notation: This notation describes the upper bound on the running time of an algorithm. It describes the worst-case scenario for the algorithm's running time. For example, an algorithm with a running time of O(n) means that the running time will never exceed a multiple of n, even for significant inputs.
- Big O notation: This notation is used to describe the lower bound on the running time of an algorithm. It describes the best-case scenario for the algorithm's running time. For example, an algorithm with a running time of O(n) means that the running time will always be at least a multiple of n, even for minimal inputs.
- Big T notation: This notation describes the tight bound on the running time of an algorithm. It describes the average-case scenario for the algorithm's running time. For example, an algorithm with a running time of T(n) means that the running time will always be a multiple of n for all inputs.
If you want to determine the complexity of an algorithm in terms of time and space, you will need to consider the following factors:
- The size of the input: The complexity of an algorithm is often directly related to the input size. For example, an algorithm that processes a list of n elements will generally have a complexity of at least O(n).
- The number of operations performed: The more functions an algorithm performs, the slower it will be. You should consider the number of parts required to solve the problem and how they scale with the input size.
- The type of operations performed: Some functions are more expensive than others. For example, performing a search on a sorted list is generally faster than a search on an unsorted list.
Considering these factors, you can determine an algorithm's time and space complexity and compare it to other algorithms to choose the most efficient one for a given problem.
5. Implement Data Structures and Algorithms From Scratch.
Implementing data structures and algorithms from scratch can significantly improve your understanding of these concepts and how they work. It is also a valuable skill to have as a software engineer, as you may be asked to implement these concepts in your work.
To implement data structures and algorithms from scratch, you will need a strong foundation in computer science principles and a good understanding of the problem you are trying to solve. To get started, follow these steps:
- Understand the problem: Make sure you clearly understand the problem you are trying to solve and the requirements.
- Research existing solutions: Look for data structures and algorithms that solve similar problems. With the knowledge you will acquire, you will be able to get a better understanding of the types of solutions that are possible and help you understand the trade-offs between different approaches.
- Choose a data structure or algorithm: Based on your understanding of the problem and existing solutions, choose the data structure or algorithm that you think will be most effective.
- Design your solution: Break down the problem into smaller parts and devise a plan using your chosen data structure or algorithm.
- Implement your solution: Write code to implement your solution in a programming language of your choice.
- Test your solution: Test your solution using a variety of test cases to ensure that it is correct and efficient.
- Optimize your solution: Optimize your solution to improve its performance. It may involve improving the algorithm's efficiency, using a more efficient data structure, or making other changes.
It is essential to remember that implementing data structures and algorithms from scratch is challenging and may require significant time and effort. However, the knowledge and skills you will gain from this process will be valuable for your future career as a software engineer.
6. Work on Problems from Online Judges and Coding Challenges:
Working on problems from online judges and coding challenges is a great way to improve your skills in data structures and algorithms. These platforms often provide a wide range of issues of varying difficulty levels, which allows you to challenge yourself and learn at your own pace.
Here are some tips for working on problems from online judges and coding challenges:
- Start with easier problems: Begin with issues that are relatively easy to solve, as this will help you build up your skills and confidence. You can gradually move on to more complex problems as you become more proficient.
- Practice regularly: Make time to practice regularly, as this will help you retain the knowledge and skills you have gained. It is generally more effective to practice for shorter periods regularly rather than for more extended periods infrequently.
- Use multiple resources: Utilize various resources, such as online tutorials, textbooks, and blogs, to deepen your understanding of data structures and algorithms. It will help you learn different approaches and perspectives on solving problems.
- Understand the problem: Make sure you fully understand it before working on it. Take the time to read the problem statement carefully and consider any edge cases or constraints that may affect your solution.
- Break down the problem: Break the problem into smaller, more manageable parts. It will make it easier to solve and help you identify which data structures and algorithms are most appropriate for solving the problem.
- Test your solution: Test your solution using a variety of test cases to ensure that it is correct and efficient. It will help you identify and fix any errors or inefficiencies in your answer.
7. Study the Solutions to Problems That You Struggle with:
If you get stuck on a problem, try understanding the solution and thinking about why it works. It will help you learn from your mistakes and improve your skills.
Studying the solutions to problems you struggle with is an effective way to improve your data structures and algorithms skills. Here are some tips for looking for answers to the issues that you work with:
Understand the problem: Before studying the solution, make sure you fully understand the problem. Take the time to read the problem statement carefully and consider any edge cases or constraints that may affect the answer.
- Break down the solution: Break the solution into smaller, more manageable parts. It will make it easier to understand and help you identify which data structures and algorithms are used in the solution.
- Understand the logic behind the solution: Try to understand the logic behind the answer and how it solves the problem. It will help you internalize the solution and apply it to similar situations in the future.
- Practice implementing the solution: Try to implement the solution on your own, using the programming language of your choice. It will help you better understand the solution and improve your coding skills.
- Test the solution: Test the solution using a variety of test cases to ensure that it is correct and efficient. It will help you identify any errors or inefficiencies in the key.
8. Learn About Advanced Data Structures and Algorithms:
There are many advanced data structures and algorithms that you can learn to improve your skills in data structures and algorithms. Some examples of advanced data structures and algorithms include:
- Heap: The heap is an entirely binary tree where each node satisfies the heap property, which states that the value of each node is greater than or equal to the importance of its children. There are two types of heaps: min heaps, in which the value of each node is greater than or equal to the values of its children, and max heaps, in which the value of each node is less than or equal to the importance of its children. Heaps are often used to implement priority queues and can also be used to sort data efficiently.
- Graph algorithms: Graph algorithms are used to process data structures represented as graphs. Use these algorithms to find the shortest path between two nodes in a graph, detect cycles in a graph, or find the minimum spanning tree of a graph.
- Dynamic programming: It solves problems by breaking them into smaller subproblems and storing the solutions in a table. It allows for more efficient solutions, as subproblems that have already been solved do not need to be solved again. Dynamic programming is often used for optimization problems, such as finding the shortest path between two nodes in a graph.
- Divide and Conquer: The concept of divide and conquer refers to a technique for solving problems by dividing them into smaller subproblems and then recursively solving them. This approach is often used to solve problems that can be divided into smaller subproblems similar to the original problem, such as sorting algorithms.
- Randomized algorithms: Randomized algorithms use random numbers to solve problems. These algorithms are often used when it is difficult or impossible to predict the input to a problem. Who can use them to solve problems such as finding the minimum element in an array or generating random numbers?
By learning about these and other advanced data structures and algorithms, you can improve your skills in data structures and algorithms and become more proficient in solving complex problems.
9. Create a Project:
Here are a few ideas for projects you can work on to improve your skills in data structures and algorithms:
- Implement a data structure from scratch: Choose a data structure, such as a linked list or a binary tree, and try to implement it yourself. It will help you understand how the data structure works and how to use it effectively.
- Solve a well-known problem: Many well-known issues have been studied extensively, such as the travelling salesperson problem or the shortest path problem. Try solving one of these problems using data structures and algorithms.
- Create a program to sort a large dataset: Choose a sorting algorithm, such as quicksort or mergesort, and use it to sort a large dataset. Measure the performance of your algorithm and try to optimize it for efficiency.
- Build a search engine: Create a program to search a large dataset for specific information. It could involve implementing a search algorithm, such as a binary search, and building a data structure, such as an index, to facilitate the search.
- Design a recommendation system: Create a program to recommend items to users based on their past behaviour. It could involve using data structures such as graphs or matrices to represent the relationships between objects and users.
- Create a program to optimize a scheduling problem: Choose a problem, such as scheduling jobs on a set of machines, and use data structures and algorithms to find an optimal solution.
Remember to start small and gradually work your way up to more complex projects as you improve your skills. Feel free to ask for help if you need it or guidance if you get stuck – many resources available can offer support.
10. Read Articles and Papers Related to Data Structures and Algorithms:
There are many ways to improve your understanding of data structures and algorithms. Reading articles and papers is a great way to learn about new techniques and approaches and deepen your understanding of familiar topics.
Here are a few suggestions for finding articles and papers to read:
- Search online for articles and papers on topics you are interested in. Many websites and databases provide access to technical reports and documents, such as ACM Digital Library, IEEE Xplore, and arXiv.
- Follow researchers and organizations in the field on social media or through email newsletters to stay updated on new publications and developments.
- Consider joining a professional association or society related to data structures and algorithms, such as the Association for Computing Machinery (ACM) or the Institute of Electrical and Electronics Engineers (IEEE). These organizations often provide access to various resources, including articles, papers, and online courses.
- Look for online articles or tutorials explaining different data structures and algorithms. Many websites, provide detailed explanations and examples of various data structures and algorithms.
- Join online communities or forums related to data structures and algorithms. These communities can provide a space for discussing and learning with other interested people.
By reading articles and papers and staying up-to-date with the latest research, you can gain a deeper understanding of data structures and algorithms and become more proficient in these critical topics.
Conclusion:
To conclude, getting better at data structures and algorithms involves a combination of Practice, learning, and engagement with other people interested in these topics. By dedicating time to implementing different data structures and algorithms on your own, reading relevant articles and research papers, attending workshops or lectures, and joining online communities, you can gain a deeper understanding of these tools and how to use them to solve problems. You can become proficient in using data structures and algorithms to solve various issues with effort and dedication.