Size of Class in C++
Introduction:
A class in C++ acts as a template for constructing objects with certain characteristics and actions. Comprehending the dimensions of a class is essential for effective memory management and enhancing software efficiency. The memory needed to hold a class's data members determines the class size. Because virtual function tables and padding are examples of possible concealed data members, even classes that appear to be empty have a size larger than zero. In C++, the "sizeof ()" operation lets us find out how much memory a class uses.
There is no such thing as an object when structure is first introduced in C. Thus, it is determined to maintain the zero size of empty structures in C programming exclusively in accordance with the C standard. In C++, the size of an empty structure or class is one byte since calling a function requires that the structure have a minimum of one byte or one byte in size. The same is true for the classes; in order for them to be distinguished from one another, they each need one byte of memory.
When working with embedded devices that have limited memory or resource-intensive applications, this expertise becomes very important. Furthermore, it's important to comprehend how base and derived classes affect the total size when utilizing inheritance or polymorphism. Effective class size management is crucial to C++ development because it guarantees smooth program operation and efficient use of system resources.
Size of empty class:
- The following code shows the size of the empty class:
Program:
#include<iostream> using namespace std; class EmptyClass { }; int main() { cout <<"Size of the Empty Class is = "<< sizeof(EmptyClass); return 0; }
Output:
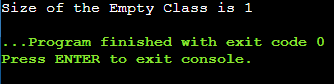
Why does a C++ empty class really require one byte?
To put it simply, a class that has no objects needs no space assigned to it. Since the space has been allocated during class instantiation, the compiler allots one byte to an empty class object in order to uniquely identify it.
Multiple objects in a class may have distinct memory addresses that differ from one another. Imagine that a class has no size at all. What would be kept in such a memory location? It requires memory to be stored when we created an object of an empty class in a C++ application; the smallest amount of memory that may be reserved is 1 byte. As a result, each object created from an empty class will have a distinct address.
- An empty class has a size that is not zero. It is typically 1 byte. To guarantee that the two distinct objects will have distinct addresses, it is nonzero. It is explained in detail in the following code:
Program:
#include <iostream> using namespace std; class Empty_Class { }; int main() { Empty_Class p, q; if (&p == &q) cout << "Same size is Impossible " << endl; else cout << "Fine " << endl; return 0; }
Output:
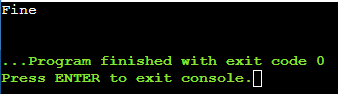
- "new" consistently yields pointers to unique objects for the same reason—different things need to have different addresses. View the example that follows.
Program:
#include <iostream> using namespace std; class Empty_Class { }; int main() { Empty_Class* m = new Empty_Class; Empty_Class* n = new Empty_Class; if (m == n) cout << "Impossible " << endl; else cout << "Fine " << endl; return 0; }
Output:
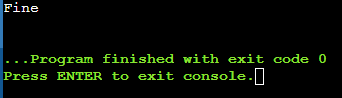
- The final result is not larger than 4. It's interesting to note that there's no requirement for a null base class to be represented with a distinct byte. Compilers are, therefore, free to optimize when base classes are empty.
Program:
#include <iostream> using namespace std; class empty_class { }; class derived_class : empty_class { int p; }; int main() { cout << "Size of the Derived Class is " << sizeof(derived_class); return 0; }
Output:
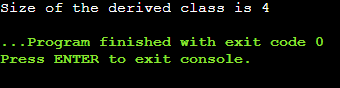
Conclusion:
In conclusion, memory management and speed optimization in C++ greatly depend on the size of a class. We may assess a member object of that class's memory footprint using the "size" operator. It considers the size of its data members, any alignment-related padding, and, in the event of polymorphic classes, maybe virtual table references. Making informed design decisions and ensuring effective resource use are made easier with an understanding of class sizes. Furthermore, platform-specific differences must be taken into consideration, as various compilers and architectures may behave differently when it comes to padding and memory alignment.