C++ Range-based For Loop
In C++ language, the range-based for loop was added, which is far superior than the ordinary For loop. The implementation of a range-based for loop doesn't really need much code. It's a sequential generator that iterates each container element across a set of values (from beginning to end). This for
loop is specifically used with collections such as arrays and vectors.
Syntax:
for (range_of_declaration : range_of_expression ) {
// program code
}
- Range_of_declaration: It's used to define a variable with the same type as the accumulated items indicated by the range expression or a value to that type.
- Range_of_expression: It defines a range expression that represents the appropriate sequence of items.
- Program code: It specifies the content of the range-based for loop, which comprises one or even more lines to be repeated until the range-expression is finished.
We may use the auto keyword to automatically identify the data type of the range expression if we are not familiar with the data type of the contained items.
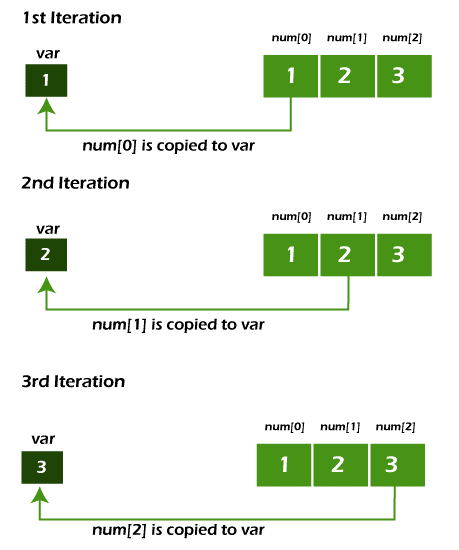
Fig. Ranged for Loop working
Range based For Loop Using Array:
This method is explained below using an example.
Example:
#include <iostream>
using namespace std;
int main() {
// initializing an array
int numArrays[] = {10, 20, 30, 40, 50};
// using ranged for loop to print the array of given elements
for (int x : numArrays) {
cout << x << " ";
}
return 0;
}
Output:
10 20 30 40 50
Explanation:
We declared and initialised an int array namely numArrays in this example. To print the items in numArrays, we utilised the ranged for loop.
The first iteration accepts the value of an array's first element, which is 10, the second iteration uses the input of 20 and prints it, and so on.
The ranging for loop generates a sequence the array from start to finish automatically. The loop's number of iterations does not need to be specified.
Range based For Loop Using Vector:
This method is explained below using an example.
Example:
#include <iostream>
#include <vector>
using namespace std;
int main() {
// declaring and initializing vector
vector<int> num_vect = {10, 20, 30, 40, 50};
// printing the vector elements
for (int x : num_vect) {
cout << x << " ";
}
return 0;
}
Output:
10 20 30 40 50
Explanation:
We declared and initialised a vector<int> array namely num_vect in this example. To print the items in num_vect, we utilised the ranged for loop.
The first iteration accepts the value represented by int x of an array's first element, which is 10, the second iteration uses the input of 20 and prints it, and so on.
Declaring a Collection inside the For Loop:
This method is explained below using an example.
Example:
#include <iostream>
using namespace std;
int main() {
// defining the collection inside the loop itself
for (int x : {10, 20, 30, 40, 50}) {
cout << x << " ";
}
return 0;
}
Output:
10 20 30 40 50
Explanation:
The collection has been defined within the loop itself, i.e.
Ranged_Expression = {1, 2, 3, 4, 5}
This is also a legal technique to use the rangedg for loop, and it functions similarly to using a real array or vector.
Best Practices for C++ Ranged For Loops:
In each iteration of the for loop in the examples above, we declared a variable to hold every element of the collection.
int nums[3] = {10, 20, 30};
// copying the elements of nums to vars
for (int vars : nums) {
// programming code
}
But it's preferable to write the range based for loop like follows:
// accessing the memory location of elements of nums
for (int &vars : nums) {
// programming code
}
We should notice how & is used before var.
int vars: nums - In each iteration, replicates every element of nums here to vars variable. This is detrimental to computer memory.
int &vars: nums - Doesn't really replicate all of nums' elements to vars. Instead, nums' elements are accessed straight from nums. This is more practical.
The reference operator is represented by the & symbol and the C++ pointers will teach us more about it.
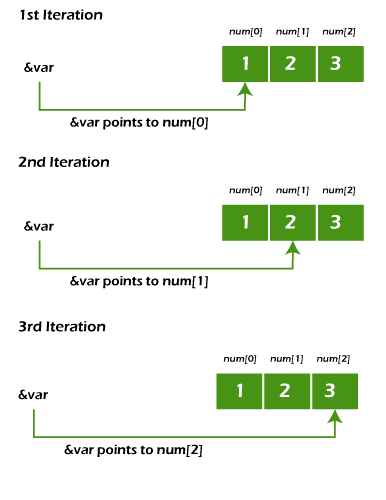
Fig. Address pointer working in ranged for Loop
Note: It is preferable using the const keyword in ranged declaration if we are not changing the array/vector/collection inside the loop.
Advantages of using Range-based for loop:
- It's simple to use, and the syntax is straightforward.
- The number of components in a container does not need to be calculated in a range-based for loop.
- It detects the containers' beginning and ending components.
- We can simply change the container's size and components.
- It does not duplicate the components in any way.
- It's a lot quicker than the standard for loop.
- The auto keyword is commonly used to determine the data type of container components.
Disadvantages of using Range-based for loop:
- It is unable to traverse a section of a list.
- It can't be utilised to go backwards in time.
- It is not suitable for usage in pointers.
- It does not include a current element index.