Object Slicing in C++
In this article, we will learn about Object slicing. When an object from a derived class is assigned to an object from a base class in C++, these extra attributes are removed (not considered). This entire process is known as object slicing. Object slicing is the process of giving the object of the base class priority over additional components of a derived class that are either sliced or not used.
In the C++ programming language, it is feasible to assign an object from a base class to an object from a derived class, but not the other way around. We can utilise a dynamic pointer to solve this slicing difficulty.
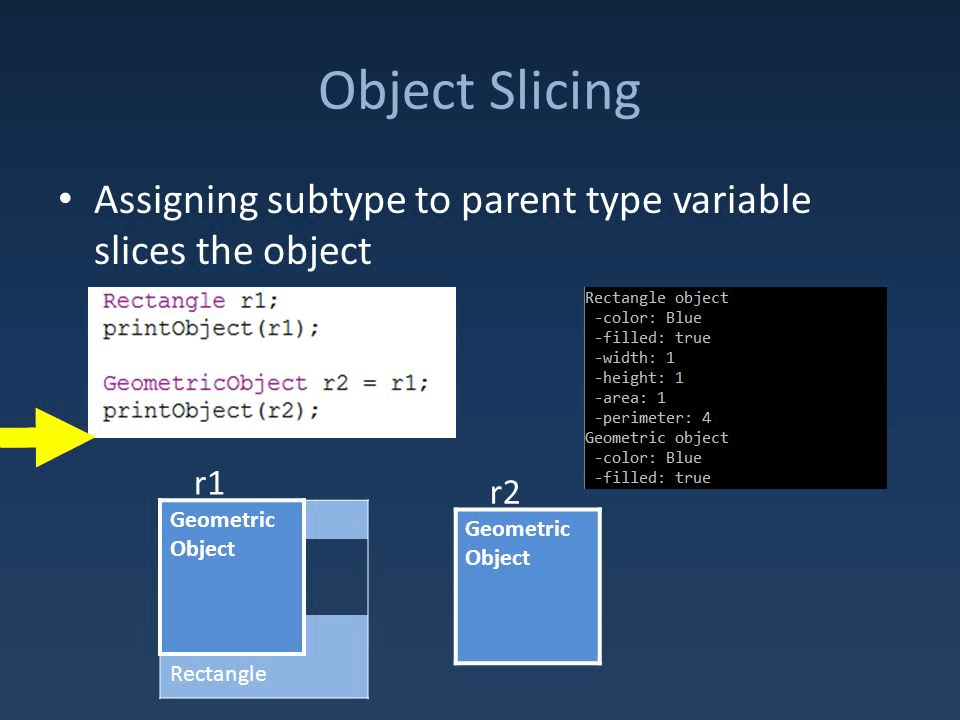
Example 1:
// The mechanism or operation of the object slicing
// approach is illustrated in x C++ program.
#include <iostream>
using namespace std ;
// Based class
class Based {
protected :
int j ;
public:
Based ( int x ) {
j = x ;
}
virtual void
display ( ) // virtual function that is initially declared in the base class
// and then re-declared in the derived class
{
cout<< "I am Base class object, j = " << j <<endl ;
}
} ;
// Derived class
class Derived : public Based {
int j ;
public:
Derived(int x, int y)
: Based(x)
{
// assigning the value to the data members of
// derived class
j = y ;
}
virtual void display()
{
cout<< "I am Derived class object, j = " << j
<< ", j = " << j <<endl ;
}
} ;
// Global method, Base class
// object is passed by value
void somefunction( Basedobj ) {
obj.display() ;
}
int main()
{
Based y(55) ;
Derived d(34, 43) ;
somefunction(y) ;
// Object Slicing, the member j of d is
// sliced off
somefunction(d) ;
return 0 ;
}
Output:

Using pointers or references, we can avoid the aforementioned unwanted behaviour. Object slicing is avoided when pointers or references to objects are provided as function arguments because each form of pointer or reference requires the same amount of memory. For instance, object slicing is prevented if the global function myfunction() in the program above is changed to the following.
Example 2:
// The mechanism or operation of the object slicing
// approach is illustrated in x C++ program.
#include <iostream>
using namespace std ;
// Based class
class Based {
protected :
int j ;
public:
Based ( int x ) {
j = x ;
}
virtual void
display ( ) // virtual function that is initially declared in the base class
// and then re-declared in the derived class
{
cout<< "I am Base class object, j = " << j <<endl ;
}
} ;
// Derived class
class Derived : public Based {
int j ;
public:
Derived(int x, int y)
: Based(x)
{
// assigning the value to the data members of
// derived class
j = y ;
}
virtual void display()
{
cout<< "I am Derived class object, j = " << j
<< ", j = " << j <<endl ;
}
} ;
// rest of code is similar to the above code except this part
void somefunction( Based&obj )
{
obj.display();
}
// rest of code is similar to above code except this part
int main()
{
Based y(55) ;
Derived d(34, 43) ;
somefunction(y) ;
// Object Slicing, the member j of d is
// sliced off
somefunction(d) ;
return 0 ;
}
Output:

If we use pointers, the result is the same.
When an object of a derived class is supplied to a function that also accepts an object of a base class as an argument, object slicing is used.
Example 3:
// The mechanism or operation of the object slicing
// approach is illustrated in x C++ program.
#include <iostream>
using namespace std ;
// Based class
class Based {
protected :
int j ;
public:
Based ( int x ) {
j = x ;
}
virtual void
display ( ) // virtual function that is initially declared in the base class
// and then re-declared in the derived class
{
cout<< "I am Base class object, j = " << j <<endl ;
}
} ;
// Derived class
class Derived : public Based {
int j ;
public:
Derived(int x, int y)
: Based(x)
{
// assigning the value to the data members of
// derived class
j = y ;
}
virtual void display()
{
cout<< "I am Derived class object, j = " << j
<< ", j = " << j <<endl ;
}
} ;
// rest of code is similar to the above code except this part
void somefunc (Based *objp)
{
objp->display();
}
int main()
{
Based *xp = new Based(99) ;
Derived *yp = new Derived(89, 98);
somefunc(xp);
somefunc(yp); // No Object Slicing
return 0;
}
Output:

By making the base class function pure virtual and forbidding object creation, object slicing can be avoided. A class that includes a pure virtual method cannot have its objects created.