Include Guards in C++
In C++ programming, we frequently utilize a class more than once, so it is necessary to create a header file and include it in the main program. Now, occasionally a specific header file is included numerous times, either directly or indirectly which results error.
Let's look at one example to understand better the requirements for including guards:
Create an Animal class in program one and save it as "Animal.h". The program for the same is listed below:
// A header is created by a C++ program.
//The file is called "Animal.h."
#include <iostream>
#include <string>
usingnamespace std;
// Animal Category
class Animal {
string name, color, type;
public:
// The ability to accept input
void input()
{
name = "Dog";
color = "White";
}
// Member variables show function
// variables
void display()
{
cout << name << " is of "
<< color << endl;
}
};
Create a Dog class in program two and save it as Dog.h. Don't forget to include the "Animal.h" header file:
//Header file creation in C++
//referred to as Dog.h
/ /Include the "Animal.h" header file.
#include "Animal.h"
// Canine Class
class Dog {
Animal d;
public:
// Add input to a variable's member
// calling a function from another
//header file,
void dog_input() { d.input();
// a feature that shows the member
// variable calling a function
// a different header file
void dog_display() { d.display(); }
};
Create a main.cpp file in program three and place it above both header files. The program for the same is listed below:
// a C++ application to demonstrate
// comprise guards
#include "Animal.h"
#include "Dog.h"
#include <iostream>
usingnamespace std;
// Driver Number
int main()
{
// Dog-class objects in
// the header file "Dog.h"
Dog a;
// Call Member Function
a.dog_input();
a.dog_display();
return 0;
}
Output:
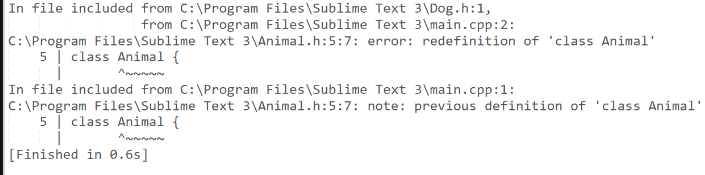
The following error occurs when the program "main.cpp" mentioned above is run:
Explanation:
This problem is caused by the fact that there are two definitions of the Animal Class in the "main.cpp" program, which is created when including Animal.h is used to build the program and define the Animal class. Now let's use include guards to fix the problem.
The C and C++ programming languages use a special construct called a #include guard, often referred to as a macro guard, header guard, or file guard, to avoid the problem of double inclusion when using the include directive.
Solution:
Regardless of how many times a file is included, include guards guarantee it that the compiler will only process it once. Pre-processor directives called have guards ensure that a file will only be included once.
- #ifndef: It checks to see if the specified macros are present.
- #define: It is used to define the macros using.
- #endif: It terminates the directive #ifndef.
Example:
#define ANIMAL(Any word you like but unique to program)
#define ANIMAL(same word as used earlier)
class Animal {
};
#endif
Define the "Animal.h" header file as follows:
// ANIMALS IF DECLARED is checked.
#ifndef _ANIMALS_
//If applicable, define "ANIMALS."
//circumstances fail
#define _ANIMALS_
#include <iostream>
#include <string>
usingnamespace std;
// Animal Category
class Animal {
string name, color, type;
public:
// function to receive input
// component variable
void input()
{
name = "Dog";
color = "White";
}
// feature that shows the
// component variable
void display()
{
cout << name << " is of"
<< color << endl;
}
};
#endif
Output:
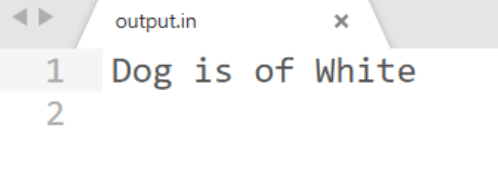
Explanation:
Animal.h is included, and the animal class is declared when primary.cpp executes. The code continues to run smoothly despite the Animal's first line. h header is directed, and _ANIMALS_ is not defined. When the Dog. The h header file, which in turn contained Animal.h, was included, this time _ANIMALS_ was defined in the program; therefore, the first line's #ifndef condition was true, skipping to the last line's #endif. If the pre-processor has that name specified, it skips the entire file and jumps to #endif rather than processing it.