C++ Math Functions
C++ Math Functions: Like other programming languages, C++ offers plenty of mathematical functions needed for various purposes. These functions are defined mainly in the math library in C++. Let us look at multiple categories to understand multiple functions along with their descriptions through the following tables.
Exponential Functions
Function | Description |
log(x) | It evaluate the natural algorithm of argument x. |
log10(x) | It evaluate the common algorithm of x. |
modf(x) | Breaks number into a fraction and integer parts |
exp2(x) | Evaluates base 2 exponents of x. |
log2(x) | Evaluates base 2 logarithms of x. |
scalbn(x,n) | It evaluates the product of x raised to power n. |
exp(x) | It evaluates the exponential e raised to the power x. |
idexp(float x, int e) | It evaluates the product of x and 2 raised to the power 2. |
log1p(x) | It evaluates the natural algorithm of x plus one. |
There are many such relevant exponential functions present in the library of C++. Let us now look at some other math functions.
Maximum and minimum difference
Function | Description |
fmin() | Returns smallest number among two numbers(x and y). |
fmax(x,y) | Returns largest among two numbers(x and y). |
fdim(x,y) | Calculates positive difference of x and y. |
Power functions
Function | Description |
pow(x,y) | It evaluates power of x raised to y. |
sqrt(x) | It evaluates the square-root of x. |
hypot() | Calculates hypotenuse of right angled triangle. |
cbrt(x) | Finds the cube-root of x. |
Trigonometric functions
Function | Description |
cos | Evaluates cosine. |
sin | Evaluates sine. |
tan | Evaluates tangent. |
acos, asin, atan | Evaluates arc cos, arc sine and arc tangent functions. |
These tables above are some basic math functions used in C++. Let us now look at some other function which needs proper description.
Note: In order to use the functions in our program we need to include <math.h> or <cmath> in our program.
Other functions
- double sin(double): It provides verified sine value by taking angle in degree.
- double cos(double): It provides verified cosine value by taking angle in degree.
- double tan(double): It provides verified tangent value by taking angle in degree.
- double sqrt(double): It provides non-negative square-root by taking the number as an argument.
- int abs(int): Returns positive absolute value regardless of positive or negative sign.
- double pow(double, double): It provides one argument as a base and the other as an exponent.
- double floor(double): It provides integer value less than or equal to the argument.
- double atan2(double, double): This function returns the arc tangent of (double a)/(double b).
- double ceil(double): It provides the smallest integer as double, not less than the argument provided.
Let’s look at some programming example to understand the working of these functions.
Example 1:
#include<iostream> #include<math.h> using namespace std; int main() { short int si = 1200; int i = -200; long int li = 4300; float f = 23.41; double d = 210.317; cout<<"Square root of(si): "<<sqrt(si)<<endl; cout<<"Power of(li, 3): "<<pow(li, 3)<<endl; cout<<"Sine of(d): "<<sin(d)<<endl; cout<<"Adsolute of(i) : "<<abs(i)<<endl; cout<<"Floor value of(d): "<<floor(d)<<endl; cout<<"Square-Root of(f): "<<sqrt(f)<<endl; cout<<"Power of(d, 2): "<<pow(d, 2)<<endl; }
Output:

Explanation:
In the above code, we have used some of the functions present in the <math.h> library to demonstrate how these functions work in the program. The functions used in the program are pre-defined in the library of mathematical functions in C++. We have defined several functions and have initialized them with some random values of their respective data type. The values in the output are the result after mathematical calculations.
Example 2:
#include <iostream> #include <math.h> using namespace std; int main() { cout << exp(5) << "\n"; cout << log(4) << "\n"; cout << log10(3) << "\n"; cout << exp2(2) << "\n"; cout << log2(1) << "\n"; cout << logb(0) << "\n"; return 0; }
Output:
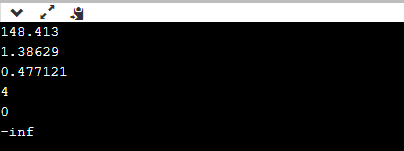
Explanation:
The above code is another demonstration of using logarithmic functions present in the C++ math functions library. We have used different types of logarithmic functions to display their values after evaluation.
Insights:
Being the superset of C, C++ supports a huge number of useful mathematical functions. The benefit of using these functions is the reduction of space in the memory to define them while writing codes specifically for these operations. It reduces human efforts and errors as the compiler itself takes care of these things. Therefore, instead of focusing on implementation, these functions can directly simplify a large set of operations. Moreover, by using these functions, we can simplify the program itself.