How to create the Processes with Fork in C++
In this article, we are going to explain the different methods that help us to create processes with a fork(). There are two methods to do so. These methods are as follows:
- By using getpid().
- By using getppid().
Now, we will discuss them individually.
1. By Using getpid()
In this method, a unique id is generated when a new process is started. This unique id is called a process id. With the help of this getpid() function, the program returns the process id. It is also used to generate the unique and temporary file name.
Syntax:
pid_t getpid();
Example:
#include <unistd.h>
#include <stdio.h>
int main()
{
int n1 = fork();
int n2 = fork();
if (n1 > 0 && n2 > 0) {
printf("it is parent class\n");
printf("%d %d \n", n1, n2);
printf(" my process id is %d \n", getpid());
}
else if (n1 == 0 && n2 > 0)
{
printf("it is First child class\n");
printf("%d %d \n", n1, n2);
printf("my process id is %d \n", getpid());
}
else if (n1 > 0 && n2 == 0)
{
printf("It is Second child class\n");
printf("%d %d \n", n1, n2);
printf("my process id is %d \n", getpid());
}
else {
printf("It is third child class\n");
printf("%d %d \n", n1, n2);
printf(" my process id is %d \n", getpid());
}
return 0;
}
Output:
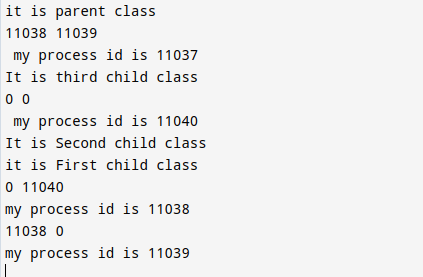
2. By Using getppid()
This method returns the process id of the parent class.
Syntax:
pid_t getppid();
Example:
#include <unistd.h>
#include <stdio.h>
int main()
{
int n1 = fork();
int n2 = fork();
if (n1 > 0 && n2 > 0)
{
printf(" It is the parent class\n");
printf("%d %d \n", n1, n2);
printf(" my process id is %d \n", getpid());
printf(" my parentid is %d \n", getppid());
}
else if (n1 == 0 && n2 > 0)
{
printf("It is the First child class\n");
printf("%d %d \n", n1, n2);
printf("my process id is %d \n", getpid());
printf(" my parentid is %d \n", getppid());
}
else if (n1 > 0 && n2 == 0)
{
printf("It is the second child class\n");
printf("%d %d \n", n1, n2);
printf("my process id is %d \n", getpid());
printf(" my parentid is %d \n", getppid());
}
else {
printf("It is third child class\n");
printf("%d %d \n", n1, n2);
printf(" my process id is %d \n", getpid());
printf(" my parentid is %d \n", getppid());
}
return 0;
}
Output:
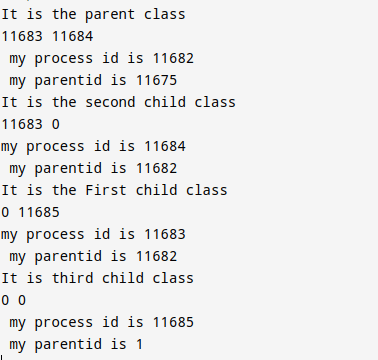