Pthreads or POSIX Threads in C++
The thread API for C/C++ is implemented by pthreads or POSIX threads. It enables the multithreading system, which enables parallel and distributed processing, and the creation of new concurrent process flows. It accomplishes this by breaking the program into smaller jobs whose operations can be interspersed to execute concurrently.
A multithreaded program has two or more concurrently running components. In such a program, each component is referred to as a thread, so each thread specifies a unique path of execution.
There was no capability created for multithreaded applications before C++ 11. Instead, this feature is totally provided by the operating system.This article will teach you how to create multithreaded C++ programs using POSIX, assuming you are using the Linux operating system. On various Unix-like POSIX systems, including FreeBSD, NetBSD, GNU/Linux, Os X, and Solaris, POSIX Threads, or Pthreads, offers API.
Developing Threads
To construct a POSIX thread, use the syntax shown below.
#include <pthread.h>
pthread_create (thread1, attr1, start_routine1, arg1)
A new thread is created and made executable; in this case via pthread create. You can call this routine any number of times anywhere in your code. The parameters' description is as follows:
Thread: A secret, individual ID for such new thread that the function returned.
Attr: An object with an opaque attribute that can be used to modify thread attributes. A thread attributes objects can be specified or NULL for the default settings.
Start routine: The C++ program that the process will run after it has been established.
Arg: A coherent argument that starts routine may accept. It must be sent as a linked cast of type void and passed via reference. If no input is to be given, NULL may be used.
The maximum thread that a process may spawn depends on the implementation. Threads are peers once they are started, and peers can start new threads. No suggested hierarchy or interdependence exists between the threads.
Stopping Threads
The procedure listed below is used to end a POSIX thread. The pthread exit is used here to end a thread specifically. The pthread exit() procedure is often invoked when a thread has finished its task but is no longer needed.
Other threads will keep running even if main() exits with pthread exit() before all of the tasks it has spawned have finished. If not, they will be ended automatically after the main() is finished.
Ac++ program that creates multiple threads, and each thread increments the global variable. However, we see unexpected results. We expect that the value of the global variable will be the sum of all the increments done by each thread. But we are seeing the global variable increasing much more than the value we are expecting.
Example:
#include <iostream>
#include <pthread.h>
#include <unistd.h>
using namespace std;
int global = 0;
void *function(void *arg) {
cout<< "Thread id is " <<pthread_self() <<endl;
for (int i = 0; i < 10000; i++) {
global++;
}
return NULL;
}
int main() {
pthread_tthread_id;
for (int i = 0; i < 5; i++) {
pthread_create(&thread_id, NULL, function, NULL);
}
pthread_exit(NULL);
cout<< "Global value is " << global <<endl;
return 0;
}
Output:
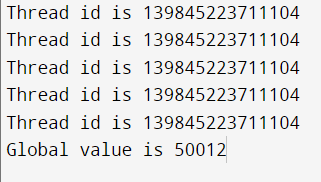
Joining and Detaching Threads
Following are the two routines that can be used to join or detach threads:
pthread_join (threadid1, status)
pthread_detach (threadid1)
The pthread join() method only blocks the caller thread until the specified 'threadid' thread exits. One of a thread's properties determines if it is actively recruiting or detached when it is created. You can only join topics that were made to be joinable. A separate thread cannot ever be joined after being formed.
Instead, we must use the pthread join command to bring all of the threads back into the original thread once they have completed their task to maintain our threads synchronised. The program will end once all the different threads have joined because the main thread will only exit once that happens.
The main goal of using Pthreads is to enhance program performance. A thread uses a lot lower operating system overhead than the cost of launching and managing a process. Compared to process management, thread management uses fewer system resources.