New Operator in C++
Dynamic memory allocation in C++ means manually allocating the memory by the developer duing run-time. The dynamic memory is allocated in the heap section of the RAM, whereas the static memory is allocated in the stack section. Therefore, during dynamic allocation, data is stored in the heap data structure in the RAM. This process of dynamic allocation is done using the new operator. This new operator can be used for predefined and custom data types. These kinds of dynamically allocated constructors are used to reduce memory usage.
Syntax
The syntax for a new operator in C++
pointer_variable_of_data_type = new data type;
Examples for using a new operator:
int *ptr = NULL;
ptr = new int();
Here, the pointer is declared with variable ptr, and we have assigned null to the variable. We can pass the parameters in the int().
We can use the new operator to use in the array, such as:
int* myarray = NULL;
myarray = new int[10];
Example:1
#include <iostream>
using namespace std;
int main() {
// declare an int pointer
int* pointInt;
// declare a float pointer
float* pointFloat;
// dynamically allocate memory
pointInt = new int;
pointFloat = new float;
// assigning value to the memory
*pointInt = 39;
*pointFloat = 69.45f;
cout << *pointInt << endl;
cout << *pointFloat << endl;
// deallocate the memory
delete pointInt;
delete pointFloat;
return 0;
}
Output:
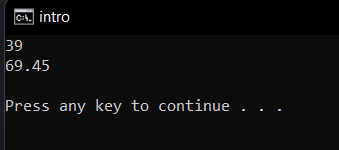
Explanation:
In this code, we have declared two pointers in the main function in the above example. These are pointInt, which stores the integer value, and pointFloat to store the float value in the same function. We have used a new statement for the above two variables and then passed two values for the variables. Then we printed the two variables using the cout statement.
Example:2 Using new statement for arrays:
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter total number of students: ";
cin >> num;
float* ptr;
// memory allocation of num number of floats
ptr = new float[num];
cout << "Enter GPA of students." << endl;
for (int i = 0; i < num; ++i) {
cout << "Student" << i + 1 << ": ";
cin >> *(ptr + i);
}
cout << "\nShowing GPA of students." << endl;
for (int i = 0; i < num; ++i) {
cout << "Student" << i + 1 << ": " << *(ptr + i) << endl;
}
// ptr memory is released
delete[] ptr;
return 0;
}
Output:
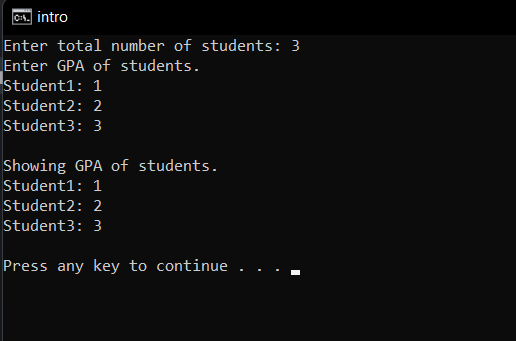
Explanation:
We have created an array using a new statement in the above example. Using for loop, we have entered the values in the array, and then we printed the values in the array using the cout statement. At last, we have used the delete statement, which is used to deallocate memory dynamically.
Example:3
#include <iostream>
using namespace std;
class Student {
string name;
public:
Student() {
// Constructor
cout << "Greeting from constructor";
}
};
int main() {
// creating student object using the new keyword
Student * student = new Student();
return 0;
}
Output:
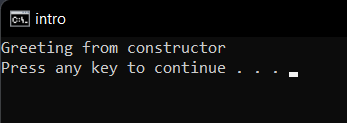
Explanation:
In the above code, we have created a class Student inside which we have declared a constructor with the same name as the class. Inside the class, we have declared a written cout statement. In the main function, we created an object for the class using the new statement.
Example: 4
#include<iostream>
using namespace std;
class example
{
int num1;
int num2;
int *ptr;
public:
// default constructor (here, it is a dynamic constructor also)
example()
{
num1 = 0;
num2 = 0;
ptr = new int;
}
//dynamic constructor with parameters
example(int x, int y, int z)
{
num1 = x;
num2 = y;
ptr = new int;
*ptr = z;
}
void display()
{
cout << num1 << " " << num2 << " " << *ptr;
}
};
int main()
{
example obj1;
example obj2(20, 50, 1);
obj1.display();
cout << endl;
obj2.display();
}
Output:
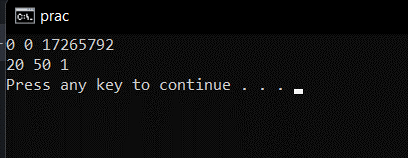
Explanation:
We used the dynamic constructor in the above example and passed a few arguments. Similarly, as in the previous example, we have created a variable with a new statement. Unlike in the previous example, we have passed a few arguments