Jump statements in C++
Introduction:
Jump statements are a C++ tool for changing a program's control flow. In C++, there are three main jump statements: return, break, and continue. There's also the goto statement, which is discouraged and seldom used since it might result in jumbled code that is difficult to comprehend and maintain.
1. Break:
The break statement is commonly employed in switch statements and loops (such as for, while, and do-while).
When a loop or switch statement encounters a break, the code instantly ends the loop or switch and moves on to the next line of code.
When a given circumstance arises, it is frequently used to end a loop early.
Example 1:
#include <iostream> int main() { for (int i = 1; i <= 10; i++) { if (i == 6) { std::cout << "Encountered 6. Breaking out of the loop." << std::endl; break; // Exit the loop when i is 6 } std::cout << "i = " << i << std::endl; } std::cout << "Loop exited." << std::endl; return 0; }
Output:
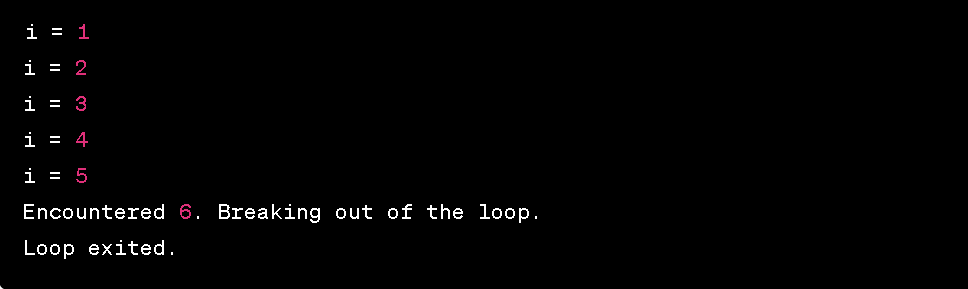
Explanation:
This example shows a for loop that runs through the numbers 1 through 10. An if statement inside the loop determines whether the current value of i equals 6. The loop ends instantly when I reach 6 since the break statement is then executed.
As you can see, the loop ends and the message "Encountered 6. Breaking out of the loop." is printed when I reach the age of 6. After the loop, the programme keeps running the code, which is why "Loop exited." is shown.
Example 2:
#include <iostream> int main() { int i = 1; while (i <= 10) { if (i == 7) { std::cout << "Encountered 7. Breaking out of the loop." << std::endl; break; // Exit the loop when i is 7 } std::cout << "i = " << i << std::endl; i++; } std::cout << "Loop exited." << std::endl; return 0; }
Output:
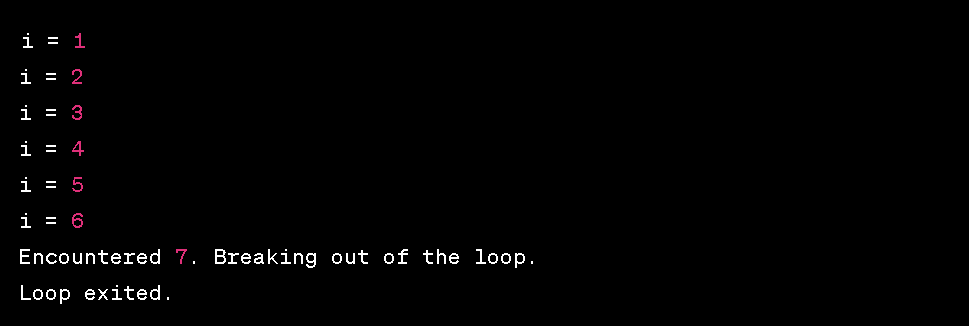
Explanation:
This example uses a while loop to cycle between 1 and 10. The break statement is run and the loop is ended when the value of i reaches 7.
It's evident that the loop ends when i reaches 7, and the programme moves on to the code that follows, displaying "Loop exited" in the process.
2. Continue:
In loops, the continue statement is also employed. When "continue" is met, the loop's current iteration is skipped, and the next one is started.
It is employed to, in response to a situation, bypass specific loop iterations.
Example 1:
#include <iostream>
int main() {
for (int i = 1; i <= 10; i++) {
if (i % 3 == 0) {
std::cout << "Skipping the number " << i << " (divisible by 3)." << std::endl;
continue; // Skip the current iteration when i is divisible by 3
}
std::cout << "i = " << i << std::endl;
}
std::cout << "Loop exited." << std::endl;
return 0;
}
Output:
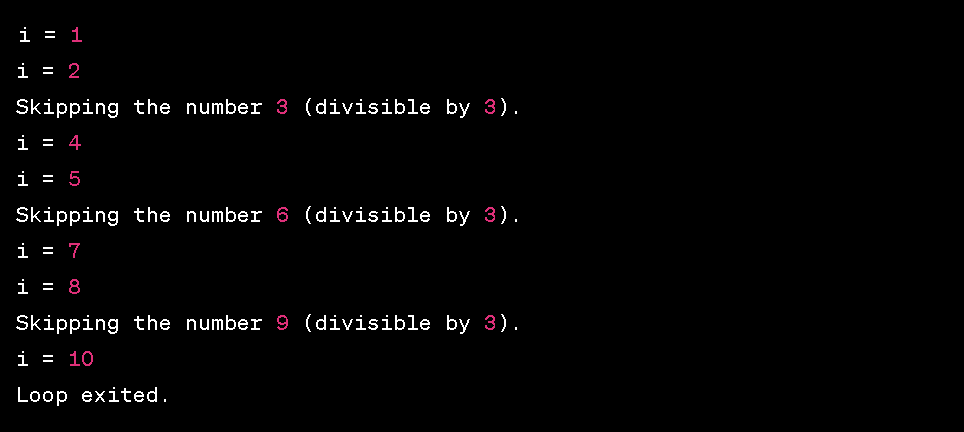
Explanation:
This example shows a for loop that runs through the numbers 1 through 10. An if statement inside the loop determines if the current value for i is divisible by 3. The continue statement, which skips the current iteration and moves on to the next, is performed when i is divisible by 3.
As you can see, the loop skips the current iteration and prints the message "Skipping the number..." when i is divisible by 3. Then, after the following iteration, the programme ends the loop, causing the message "Loop exited" to appear.
Example 2:
#include <iostream> int main() { int i = 1; while (i <= 10) { if (i % 2 == 0) { std::cout << "Skipping the even number " << i << "." << std::endl; i++; continue; // Skip the current iteration when i is even } std::cout << "i = " << i << std::endl; i++; } std::cout << "Loop exited." << std::endl; return 0; }
Output:
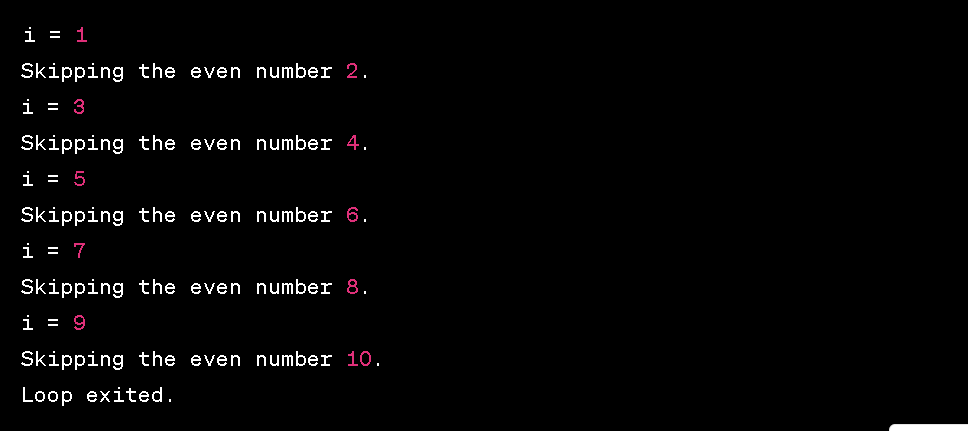
Explanation:
This example uses a while loop to cycle between 1 and 10. An if statement inside the loop determines if the current value of i is even. The continue statement is performed when i is even, skipping the current iteration and moving on to the next.
Asthe loop's current iteration is skipped and the message "Skipping the even number..." is written when i is even. Then, after the following iteration, the programme ends the loop, causing the message "Loop exited" to appear.
3. Return:
If a function has a return type, using the return statement will terminate the function and return a value.
The function ends and returns control to the caller function upon execution of return.
Example 1:
#include <iostream> int add(int a, int b) { int sum = a + b; return sum; // Returns the sum of a and b } int main() { int result = add(5, 3); std::cout << "The result of the addition is: " << result << std::endl; return 0; }
Output:

Explanation:
The add function in this example accepts two integer inputs, a and b. The function computes the sum of a and b and returns the result using the return statement.
Three and five are the parameters passed to the add method. It uses the return statement to retrieve the number 8, which is the result of adding 5 and 3. The addition's result is allocated to the result variable in the main function and subsequently reported to the console.
Example 2:
#include <iostream> // Function to calculate the square of a number double square(double number) { double result = number * number; return result; // Returns the square of the input number } int main() { double num = 4.5; double squared = square(num); std::cout << "The square of " << num << " is " << squared << std::endl; return 0; }
Output:

Explanation:
The square of a given integer may be found using the square function, which is used in this example. The input number is multiplied by itself inside the function, and the return statement is used to output the outcome.
The square function is called with the integer 4.5 in the main function, and it returns the square of 4.5, or 20.25. The console is then updated with this value.