Dynamic Objects in C++
Objects with memory allocated at runtime, often on the heap, are called dynamic objects in C++. Dynamic objects offer more flexibility than static objects generated on the stack because you can allocate and deallocate memory while a program runs. The objects in C++ can be made at runtime. C++ supports the two operators, new and delete, to conduct memory allocation and deallocation. Dynamic objects are what these objects fall under. Both the new operator and the delete operator are used to create and destroy objects dynamically. Pointers are useful for creating dynamic objects.
A key idea in memory management is represented by dynamic objects in C++, which offer a potent method for runtime memory allocation and management. Here your program runs at the same time, which is different from static objects(static objects are those that contain a specific lifetime and are they are created on the stack,
Dynamic objects are often allocated on the heap, which is a separate section of memory from the stack. The heap provides a more expansive pool of dynamically available memory. This dynamic memory allocation is critical when working with data structures of various sizes or when objects must persist outside of the function or block where they were formed.
A critical aspect is the use of pointers by dynamic objects. When a dynamic object is created, a pointer to the object's memory position is returned. This pointer can be used to access and modify the object's data.
A pointer would be used, for instance, to access and change the value of an integer that was allocated dynamically.
Example:
int* dynamicInt = new int; *dynamicInt = 42;
The above code snippet is used for the creation of a dynamic integer and assigning value to that integer.
The lifespans of dynamic objects are usually variable. Dynamic objects last until expressly destroyed with the delete operator, but static objects are automatically deleted when they exit the scope. You have precise control over how your software uses the memory resources, thanks to this dynamic lifetime management.
delete dynamicInt;
The above function deletes the previously allocated dynamic integer.
The use of dynamic memory allocation extends beyond single objects. Additionally, dynamic arrays can be made. These are particularly helpful when working with data collections whose sizes may change over time. Similar to static arrays, dynamic arrays can be accessed using array subscript notation, but they provide more scaling freedom.
int* dynamicArray = new int[4]; dynamicArray[0] = 10;
The above part of the code is used for the creation of a dynamic integer array that is of size 4 and accessing those elements present in the array.
When working with dynamic objects, effective resource management is essential. Deallocating dynamic memory helps prevent memory leaks, in which memory is allocated but never released, which can cause performance problems and even crashes. Inadequate memory management can also lead to unpredictable behavior, like accessing memory that has already been deallocated.
While dynamic objects have many advantages, they also require explicit memory management, which involves not only allocating memory with new but also making sure that memory is deallocated using delete or delete[] when it is no longer required. In modern C++, smart pointers, like std::unique_ptr and std::shared_ptr, offer safer and more automated ways to manage dynamic objects, lowering the risk of memory-related problems.
Dynamic objects need intentional memory management, they have variable lifetimes, and they are accessed using pointers. The more you know the creation, accessing, and deallocating of dynamic objects, the more memory is managed in that program.
Example 1:
Let us look at the below example program that shows the usage of dynamic objects in C++. Dynamic class is created in this program:
#include<iostream> using namespace std; class Dynamic { int x,y; public: Dynamic() { cout << "Calling a constructor" << endl; x = 1; y = 2; }; ~Dynamic() { cout << "Calling a destructor" << endl; } void show() { cout << "x = " << x << endl; cout << "y = " << y << endl; } }; int main() { Dynamic *ptr; ptr = new Dynamic; ptr->show(); delete ptr; return 0; }
In this program, a dynamic class is created using a destructor and a constructor. The constructor sets x and y, two private integer members, to 1 and 2, respectively. The destructor is in charge of cleanup. The "main" method creates a pointer to a "Dynamic" class object utilizing dynamic memory allocation (new operator). The constructor is called, followed by the "show" method, which displays the x and y values. Following that, the "delete" operator is used to deallocate the object's memory, which invokes the destructor. This aids in the discharge of the object's memory and resources. The software then returns 0, indicating that it was successful.
Output:
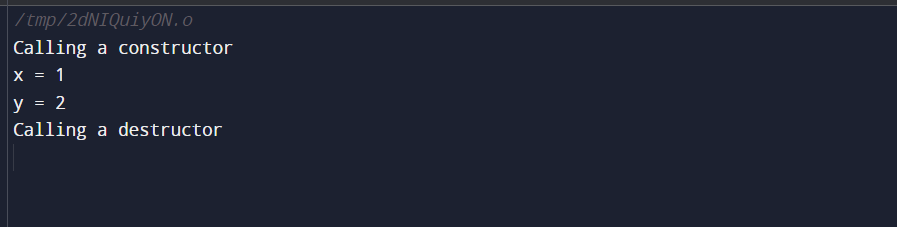
Example 2:
Here is yet another program that uses dynamic objects to show off C++. Dereference is the address of dynamic objects returned by the new operator to construct a reference to them.
#include<iostream> #include<string.h> using namespace std; class person{ private: int age; char hallticket_no[20]; public: void setdata(int age_in, char hallticket_no_in[20]) { age = age_in; strcpy(hallticket_no, hallticket_no_in); } void outdata() { cout << "Age of the Person:" << age << endl; cout << "Hallticket no: " << hallticket_no << endl; } }; int main() { person &p1 = *(new person); p1.setdata(26, "A12A4"); p1.outdata(); person &p2 = *(new person); p2.setdata(56, "A12A5"); p2.outdata(); person &p3 = *(new person); p3.setdata(32, "A12A6"); person &p4 = p3; p3.outdata(); p4.outdata(); return 0; }
The "person" class is defined in this C++ program, and its private data members are "age" (an integer) and "hallticket_no" (a character array). The class has two methods: "outdata" displays the age and hall ticket number and "setdata" allows you to set these parameters. Several "person" objects are generated using dynamic memory allocation and have their data set using the "setdata" method in the "main" function. The data for p1, p2, and p3 is set up as dynamic objects when they are formed. p4 is a reference to p3, not a new object, hence p4 and p3 have access to the same data. After the data is set for the objects p3 and p4, age and hall ticket number are displayed using the "outdata" method.
Output:
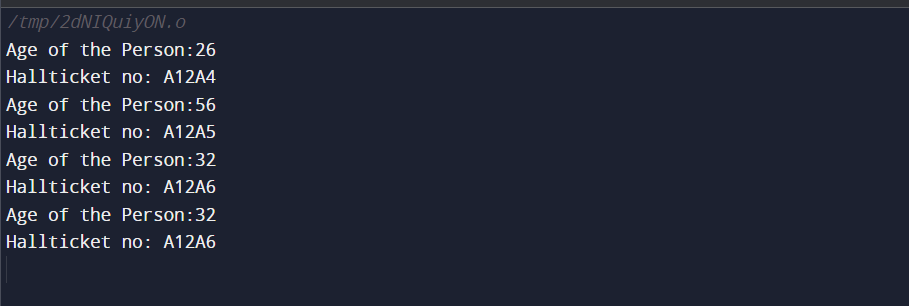
Dynamic Objects and Pointers:
Pointers are nothing but variables that are used for storing of memory addresses. They enable you to have indirect access to and control over memory-based data. Pointers, a fundamental component of C++, are closely related to dynamic objects in the following aspects.
- Creation of Dynamic Objects: Dynamic object creation takes place with the help of a new operator. This new operator returns a pointer for the newly allocated memory. Use this pointer to access and control the item.
- Deallocation of Memory: Dynamic objects must be explicitly deallocated when they are no longer needed. This is done by using the delete operator, which takes a pointer to the dynamically allocated object as its argument.
delete dynamicInt; delete[] dynamicArray;
In the first line, the dynamically allocated integer is deallocated, and in the second line, the dynamic integer array is deallocated.
- Accessing data: Dereference the pointer to gain access to the data kept in a dynamic object. The value of the object can be retrieved or changed using the dereference operator (*).
*dynamicInt = 42;
The value is assigned to a dynamically assigned integer.
- Dynamic Arrays: Pointers are also used to generate dynamic arrays. You can browse over the components of a dynamic array using pointer arithmetic. For illustration:
dynamicArray[0] = 10;
The elements of the dynamic array are accessed in the above code.
When working with dynamic objects and pointers, proper memory management is essential. Memory leaks can happen if memory is not dealt with properly, and strange behavior might happen if memory is accessed after it has been dealt with. Smart pointers, such as std::unique_ptr and std::shared_ptr, are available in modern C++ and automate various memory management tasks, making it safer and easier to work with dynamic objects.
In C++, dynamic objects and pointers are crucial for controlling memory usage and creating adaptable data structures. Pointers are used to access and interact with dynamic objects, which are created on the heap and have changeable lifetimes. Effective memory management and data manipulation in C++ programs depend on an understanding of the interaction between dynamic objects and pointers.
Dynamic Objects in Functions:
In C++, dynamic objects are essential when interacting with functions. Writing effective and memory-safe C++ code requires an understanding of how to pass dynamic objects as function parameters, return dynamic objects from functions, and manage memory within functions. We'll go into detail about each of these topics in this explanation.
Dynamic Objects Passing as Function Arguments:
You can use dynamically allocated memory within a function by passing dynamic objects as function arguments. A dynamic object's memory address (a pointer) is passed to a function when the object is passed. Let us see how it goes:
- Passing via Pointer: In the example below pointer is sent to the dynamic object which gives it an indirect access and control over the data of the object.
void modifyDynamicObject(int* dynamicInt) { *dynamicInt = 42; } int main() { int* dynamicInt = new int; modifyDynamicObject(dynamicInt); delete dynamicInt; return 0; }
In the above code snippet, the operator "new" is used to construct a dynamic integer on the heap. It is also used for updating it within a function and setting it to 42, and lastly it is used to deallocate the memory using delete. This process is used to prevent the code from memory leaks. To prevent resource leaks in C++, dynamic memory management must be done correctly.
- Passing by Reference: As an alternative, you can pass the dynamic object by reference, which is frequently preferable because it enables pointer dereferencing-free modification of the object.
void modifyDynamicObject(int& dynamicInt) { dynamicInt = 42; } int main() { int* dynamicInt = new int; modifyDynamicObject(*dynamicInt); delete dynamicInt; return 0; }
In this code, a dynamic integer is created on the heap using the new function, and its address is given to a pointer called dynamicInt. The dynamic integer is taken by reference by the function modifyDynamicObject, allowing for direct alteration of its value to 42. DynamicInt keeps the changed value after the function call. Finally, by using delete to deallocate the memory allocated on the heap correctly, memory leaks are avoided, which shows how to create dynamic objects, modify them via references, and free up memory in C++.
- Ownership and Responsibilities: Keep in mind that even when you give a dynamic object to a function, you remain in charge of managing its memory. You must make sure that the dynamic object is properly deallocated when it is no longer required if the function does not assume ownership of it.
Returning Dynamic Objects from Functions:
When you need to construct objects within a function's scope and return them to the caller, you frequently return dynamic objects from functions. To achieve this, you can use the new operator to allocate memory for the dynamic object inside the function and then return a pointer to that object. Here is how to accomplish it:
int* createDynamicInt() { int* dynamicInt = new int; *dynamicInt = 42; return dynamicInt; } int main() { int* returnedDynamicInt = createDynamicInt(); delete returnedDynamicInt; return 0; }
The createDynamicInt function in this code allocates memory for an integer on the heap in a dynamic manner, initializes it with 42, and then returns a pointer to this dynamic integer. The returned pointer is given to returnedDynamicInt in the main function. The dynamically allocated integer is used, and then it is appropriately deallocated using delete, guaranteeing responsible memory management and avoiding memory leaks, which serves as an example of how to create, return, and clean up dynamic objects in C++.
It's vital to understand that you give the caller ownership of a dynamic object when you return it from a function. To prevent memory leaks, the caller is now in charge of deallocating the memory when it is no longer required.
Using Memory in Functions:
To avoid memory leaks and guarantee effective resource use, proper memory management within functions is essential. Here are some crucial factors to take into account while handling dynamic objects inside of functions:
- When handling dynamic objects, take exception safety into account. To avoid resource leaks, ensure that allocated memory is appropriately deallocated whenever an exception is triggered.
- Consider having functions return smart pointers (such as std::shared_ptr or std::unique_ptr) to streamline memory management. Smart pointers intelligently manage dynamic object memory, lowering the possibility of memory leaks.
- Test each of your functions rigorously, especially if dynamic memory management is involved. To find and fix problems caused by memory leaks or inappropriate memory access, use memory debugging tools.
- Avoid returning pointers to objects that have been deallocated or that have lost their intended scope. Dangerous behavior may result from dangling pointers.
- Make careful to deallocate the memory that your function allocated for dynamic objects when the objects are no longer required. As necessary, use the delete or delete[] operator.
- Use the new operator to allot heap space if your function needs to build dynamic objects.
- If your function accepts ownership of dynamic objects supplied as arguments or returns ownership to the caller, make sure to specify this in the documentation.
- If your function accepts ownership of dynamic objects supplied as arguments or returns ownership to the caller, make sure to specify this in the documentation.
In C++, dynamic objects can be maintained within functions as well as supplied as function parameters and returned from them. To ensure effective and secure dynamic object handling in your C++ code, follow good memory management procedures, define ownership rules clearly, and take exception safety into account.
Challenges in Memory Management:
Managing memory in C++ can be difficult, and using dynamic memory allocation can lead to a number of frequent problems. We'll outline three major memory management difficulties here:
1. Dangling Pointers:
When a pointer keeps pointing to a memory location even after the memory it refers to has been deallocated or exits its scope, this is known as a dangling pointer. A dangling pointer can cause unexpected behavior, crashes, or data corruption when used to access or alter memory. Dangerous situations where this can happen include:
Using delete or delete[] to restore an object that has been removed, returning a function's pointer to a local variable, and gaining access to a pointer to an item that has left its range.
After allocating memory, pointers must be set to nullptr and kept valid throughout use in order to prevent dangling pointers.
2. Memory Corruption and Double Free:
When you try to deallocate memory that has already been released, you make a memory management mistake known as a double free. Memory corruption, crashes, and erratic program behavior may result from this. Double-freefrequently happens in circumstances where:
With delete or delete[], a pointer can be erased many times, and using delete along with the free function releases memory.
Make sure you only deallocate memory once for each allocated block and refrain from combining memory management operations like delete and free in the same program to prevent double freeing and memory corruption.
3. Memory Fragmentation:
When memory is divided into small, non-contiguous chunks, memory fragmentation occurs, making it difficult to allocate large blocks of memory even when there is enough free memory. fragmentation can take two different forms:
- Internal fragmentation: This takes place when allocated RAM is squandered because of padding or alignment needs. Although it doesn't decrease memory availability, it can lower overall memory efficiency.
- External Fragmentation: This condition occurs when free memory is dispersed over the heap in irregular, disjoint chunks, making it difficult to allocate big objects. Even when there is plenty of free RAM overall, external fragmentation can cause memory allocation errors.
Memory waste and fragmentation can be reduced by employing techniques like memory pooling, bespoke memory allocators, or dynamic data structures.
Writing reliable, effective, and bug-free C++ applications depends on addressing these memory management issues. Best practices include using smart pointers (std::unique_ptr and std::shared_ptr), adhering to the RAII (Resource Acquisition Is Initialization) tenets, and employing memory management libraries and debugging tools.
Dynamic Objects and Object-Oriented Programming:
In C++, dynamic objects and object-oriented programming (OOP) are two key ideas that frequently work together.
1. Classes and Dynamic Objects:
A class is a model for constructing objects in object-oriented programming (OOP). It specifies how objects of that class should be structured and behaved. Dynamic objects use dynamic memory allocation to construct instances of a class on the heap.
2. Dynamic Object Creation:
A class that is defined acts as a template. Use the new keyword, the class name, and any necessary constructor parameters to create a dynamic object of that class.
Example:
MyClass* dynamicObject = new MyClass(arg1, arg2);
3. Obtaining Dynamic Object Access:
Pointers are used to access dynamic objects. To access the dynamic object's members (data members and member functions), use the arrow operator (->). For illustration:
dynamicObj->memberFunction(); dynamicObj->dataMember = value;
You can interact with the class's declared attributes and behaviors using this syntax.
4. Lifetime Administration:
The lifespan of dynamic objects varies. Until expressly eliminated using the delete operator, they remain in existence. Dynamic objects can stay outside the confines of the function or block where they were created, in contrast to local (stack-based) objects, which are automatically destroyed when they leave their scope. They are thus appropriate in situations when items must endure beyond the local focus.
Benefits of OOP's Dynamic Objects:
Flexibility in handling resources and data is made possible by the ability to build and manage dynamic objects with various lives and sizes.
Encapsulation, which involves grouping data and methods that work with that data in a class, is encouraged by OOP concepts. By enabling you to restrict access to the object's data using member functions, dynamic objects aid in the enforcement of encapsulation.
Dynamic objects make it easier for various objects to respond to the same interface or function call in a way that is unique to their class. Polymorphism is a crucial OOP concept, which makes it possible to reuse code and handle various object kinds with flexibility.
Another OOP concept known as inheritance enables you to build new classes of basic classes. Instances of derived classes can be instances of dynamic objects, which add their unique features while inheriting the properties and characteristics of their parent classes.
By enabling the construction of objects with adjustable lifetimes and sizes, dynamic objects in C++ play a crucial role in Object-Oriented Programming. They facilitate the application of OOP concepts like encapsulation, inheritance, and polymorphism, which help to create modular, well-organized software systems. To prevent problems like memory leaks, they demand careful memory management.
Usage Patterns for Dynamic Objects:
Without a doubt, let us delve deeper into some of the more typical use cases for dynamic objects, such as dynamic data structures, file management and I/O, and dynamic polymorphism (virtual functions):
1. Dynamic Data Structures:
Dynamic data structures are collections of data that can change in size while a program is running, and dynamic objects are essential to their implementation. These data structures are extremely adaptable and are utilized in many different applications, including computer graphics and databases. Linked lists, trees, stacks, and queues are a few examples of typical dynamic data structures.
Use Cases:
Dynamic objects are frequently used to implement linked lists. A linked list's nodes are dynamically allocated memory objects. It enables effective element insertion and removal at different list locations.
Dynamic objects are used to implement dynamic arrays, commonly referred to as resizable arrays or vectors. The data is copied over to a new dynamic object when the array needs to expand beyond its initial capacity.
Dynamic objects are used as the nodes in tree architectures like binary search trees (BST) and balanced trees. For the purpose of adding and removing nodes as the tree topology changes, dynamic memory allocation is necessary.
2. File Handling and I/O:
When working with file management and input/output operations, especially while using complex data structures, dynamic objects are helpful. Data structures that must be read into memory and changed dynamically can be found in files.
Use Cases:
Dynamic objects can be used to interpret and store variable-length records, like lines of text or structured data, while reading data from a file. Dynamic objects, on the other hand, may be serialized and written back to files.
When reading from or writing to a database, database records can be represented using dynamic objects, enabling flexible data processing.
Complex data structures like trees and graphs may be stored in files. These memory structures are created and modified by dynamic objects.
3. Virtual Functions (Dynamic Polymorphism):
Through the usage of virtual functions, dynamic objects are essential to establishing dynamic polymorphism in C++. With dynamic polymorphism, the proper function implementation is chosen at runtime, and objects from various derived classes can be treated as if they were objects of the same base class.
Use Cases:
It is common to use dynamic polymorphism in situations where there is a base class and one or more derived classes. Each derived class offers its implementation of the virtual functions that are defined in the base class.
In plugin architectures, where various plugins (dynamic objects) adhere to a standard interface established by a base class, dynamic polymorphism is utilized.
Runtime method binding is made possible when a pointer or reference to an object of a base class truly points to an object of a derived class. It happens when you call a virtual function on an object of a base class that actually points to an object of a derived class.
Best Practices for Dynamic Objects:
1. Regulations for Resource Management:
When the need arises to assign dynamic memory, make use of 'new'. Only apply RAM to elements that genuinely need dynamic storage. In most cases, opt for automatic (stack-based) variables. When finished with dynamic objects, ensure the memory is correctly freed using 'delete' or the appropriate resource management processes, as failure to do so can lead to memory leaks.
Whenever feasible, utilize standard containers that manage memory allocation and deallocation autonomously. This includes stack variables and types like std::vector and std::string.
Follow the principle of RAII (Resource Acquisition Is Initialization) for effective resource management. This principle suggests that the lifecycle of resources like memory and file handles should be tied to the object's scope. It is essential to employ destructors for handling resource cleanup.
Within your program, use only the new and delete functions from C++ or the malloc and free functions from C. Combining them can cause issues like memory double-freeing.
2. Use of Smart Pointers:
In dealing with dynamic entities, the preference should be for smart pointers rather than raw pointers. If ownership is clearly defined, std::unique_ptr is suggested, whereas std::shared_ptr is recommended when multiple objects have the potential for shared possession.
The incidence of memory leak is reduced as smart pointers autonomously free up memory once it's not in use. One should employ std::shared_ptr judiciously to avoid circular references that can constrain proper deallocation of objects due to their constant zero reference counts.