Difference between exit() and _Exit() in C++
Before understanding the difference between the exit() and _Exit(), one must know about exit() and _Exit() functions.
The exit() function in C/C++
The exit() method in the C language kills the calling process without running the remaining code.
The calling function is terminated instantly, and no more processes are run using the exit() function.
It terminates processes by calling the exit() method. It is defined in the C language's "stdlib.h" header file. It gives no results back.
The syntax for exit() is as follows:
The value is returned to the parent process by the st_val.
void exit(int st_val) ;
Example
#include<bits/stdc++.h>
using namespace std ;
void my_function(void) {
cout << "Exiting from program" ;
}
int main() {
atexit(my_function) ;
exit(10) ;
cout<< "hello" ;
}
Output:
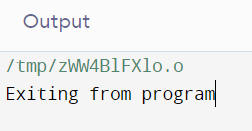
The above program prints "Exiting from program." This is available in the my_function method.
Example 2
#include <stdio.h>
#include <stdlib.h>
int main() {
int x = 10 ;
printf("The value of x : %d\n", x) ;
exit(0) ;
printf("Calling of exit()") ;
return 0 ;
}
Output:
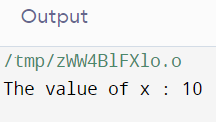
A variable 'x' is assigned a value in the above-displayed program.
The printf() method prints the variable's value, and the exit() function is called. When exit() is called, the execution is instantly terminated, and the statement in the printf() is not printed.
The following commands are used to invoke exit():
int x = 10 ;
printf ("The value of x: %d\n", x) ;
exit(0);
Example
// C/C++ example program for the exit() method.
#include <stdio.h>
#include <stdlib.h>
// the code logic
int main(void)
{
printf("START") ; //prints START
exit(0);
//
When the exit(0) method is used, the program is ended.
printf("End of program") ; // the line mentioned will not be printed.
}
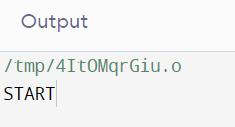
Explanation: The printf function is called in the program above, and the value is printed there first.
The exit() method is then used, which immediately terminates the program and skips printing the statement in the printf().
The _Exit() method in C/C++
The C/C++ _Exit() method provides a common program shutdown without any cleanup activities. For instance, it doesn't run any atexit-registered functions.
When a process is terminated correctly, it returns control to the host environment using the function _Exit(). It doesn't carry out any cleanup operations.
The syntax for the _Exit() method is as follows :
void _Exit(int st_val) ;
The value returned to the parent process has the name st _val.
Here the exit_code represents the program's exit status, which can be 0 or non-zero.
Return Value: The _Exit() function returns nothing.
Example
#include <stdio.h>
#include <stdlib.h>
int main() {
int x = 10 ;
printf("The value of x : %d\n", x) ;
_Exit(0) ; //_Exit()
printf("Calling of _Exit()") ;
return 0 ;
}
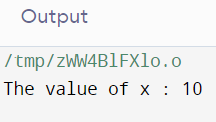
Example
// C++ program to demonstrate use of _Exit() method in C/C++
#include <stdio.h>
#include <stdlib.h>
// Driver Code
int main(void)
{
int exit_code = 10 ; //assigning the value to exit_code
printf("Termination using _Exit") ;
_Exit(exit_code) ;
}
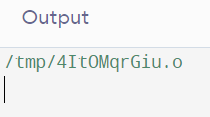
Here, the result will be nothing.
The program doesn't produce any output, and this is because of the _Exit() method.
_Exit() vs exit()
The functionality of exit() and _Exit() in C/C++ is fairly similar.
Before ending the program, the exit() function performs cleanup tasks like connection closure and buffer flushes, and this is the sole distinction between exit() and _Exit().
Let's use an illustration to grasp the distinction further. We have used exit() in the following program.
Program: Using exit() method
// C++ program that displays differences
// between the exit() and the _Exit() methods
#include <bits/stdc++.h>
using namespace std ;
void funct(void) { cout << "Exiting" ; }
// main method code
int main()
{
atexit(funct) ;
exit(10) ; //exits the program
}
Output:
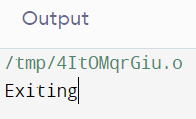
When exit() is encountered, the program is instantly ended.
Now, we switch the exit() to the _Exit() method.
Program: Using _Exit() method
// C++ program that displays differences
// between the exit() and the _Exit() method.
#include <bits/stdc++.h>
using namespace std ;
void funct(void) { cout << "Exiting" ; }
int main()
{
atexit(funct) ;
_Exit(10) ;
}
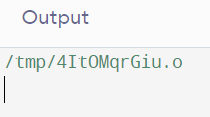
Nothing is printed because there is no output.
The same program gives a different outcome for the exit() and _Exit() methods.