C++ String Concatenation
C++ String Concatenation
In this section, we will learn about C ++ String Concatenation, what it does, how it works, and will also see its programs.
What is the String Concatenation?
The + operator can be used to form a new string between strings to link them together. That is known as concatenation.
There are different ways to perform concatenation of strings. We will be discussing each one of them.
Using strcat() function.
The strcat) (function is set to the header file of "string.h."
Syntax:
char * strcat(char * init, const char * add)
The string init and add should be of character array(char *). This function concatenates the added string to the init string end.
Example:
#include <bits/stdc++.h> using namespace std; int main() { char init[] = " init"; char add[] = " join this "; strcat(init, add); cout << init << endl; return 0; }
Output:

Using append() function.
A string in C++ is actually an object that contains functions to perform some string operations. For, e.g., you can also use the function append() to concatenate strings.
Syntax:-
String & string::append ( const string& str )
Here str is the std::string class object, which is an instantiation of the basic string class template which uses char as a type of its character. At the end of the0 init string, the append function appends the add(variable) string.
In the above example, we added space on output after firstName to create a space between Mike and Johnson. You can, however, also add a space with quotes ("or"):
#include <iostream> #include <string> using namespace std; int main () { string firstName = "Mike"; string lastName = "Johnson"; string fullName = firstName.append(lastName); cout << fullName; return 0; }
Output:
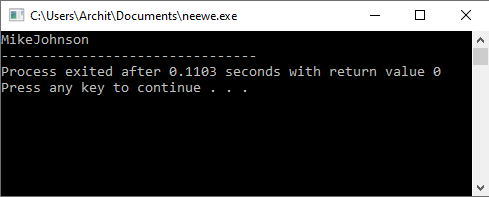
Whether you want to use + or append() is up to you. The key difference between the two is that the function append() is much quicker. Nonetheless, it may be better to only use + for checking and so on.
Using ‘+’ Operator
Syntax:
string new_string = string init + string add;
It is the simplest way to merge two strings. The + operator actually puts the two strings together and returns a concatenated string.
Example:
#include <bits/stdc++.h> using namespace std; int main() { string cha1("Hello,"); string cha2("my name is mike johnson."); string cha3("I am a software developer"); string cha4(" in Company "); string cha5(" from Us"); string cha6(" As a good developer"); // Appending the string. cha1 = cha1 + cha2 + cha3 + cha4 + cha5 + cha6; cout << cha1 << endl; return 0; }
Output:
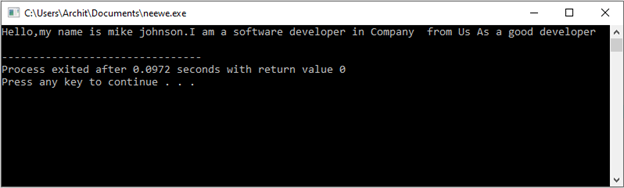
Example
#include <iostream> using namespace std; int main() { string s1, s2, result; cout << "Enter string s1: "; getline (cin, s1); cout << "Enter string s2: "; getline (cin, s2); result = s1 + s2; cout << "Resultant String = "<< result; return 0; }
Output:

Without using function
For this, we concatenate two separate strings without using strcat() as the concatenation function.
Example:
#include<iostream> #include<string.h> using namespace std; int main() { //declare strings char string1[100]; char string2[100]; //input first string cout<<"Enter first string : "<<endl; cin.getline(string1,100); //input second string cout<<"Enter second string : "<<endl; cin.getline(string2,100); //variable as loop counnters int k, f; //keep first string same for(k=0; string1[k] != '\0'; k++) { ; } //copy the characters of string 2 in string1 for(f=0; string2[f] != '\0'; f++,k++) { string1[k]=string2[f]; } //insert NULL string1[k]='\0'; //printing cout<<string1<<endl; return 0; }
Output:
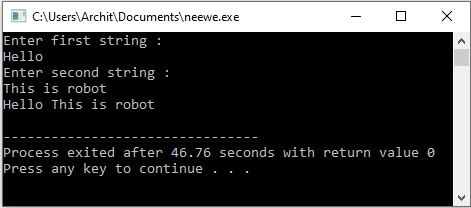