Features of OOPS in C++
What is OOPs?
The main reason programmers prefer C ++ language over C is because of the support of object-oriented programing in C++. As the name suggests, object-oriented programming or OOPs uses objects, or a program is designed using classes and objects in object-oriented programming. In OOPs, an object is a real-world entity, such as a pen, a chair, a table, etc. There are mainly three OOPs features that can make it different from non-OOPs language, which are inheritance, data binding, polymorphism, etc.
Features of OOPs
OOPS provides many features, which are:
- Classes and objects
- Abstraction
- Inheritance
- Polymorphism
- Dynamic Binding
- Message Passing
1. Classes and objects
When it comes to object-oriented programming, objects are the basic building blocks. Memory is taken up by objects, which contain data and methods or functions that operate on it. Class in a program acts as a blueprint for the object. An object is created for the class, and then we can use the object name to access the class or class member functions. The class generally will not take up any space in the memory
Example:
#include <iostream>
using namespace std;
class Student {
Public:
int id;
string name;
};
int main() {
Student s1; //creating an object of Student class
s1.id = 305; //assigned the value of id using object
s1.name = "example.com";; //assigned the value of name using object
cout<<s1.id<<endl;
cout<<s1.name<<endl;
return 0;
}
Output:
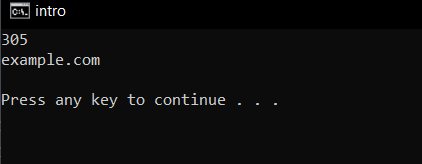
Explanation:
In the above example, we have created a class with the name student. In the main class, we created an object for the class, and then, using the object name, we assigned values to the variables and then
Abstraction
A user's attention is diverted from irrelevant information by abstraction. This means that the process of abstraction involves hiding irrelevant information from the user. For example, if we open an app on our phone, the information about how the app opens in the background is hidden from the user. This is known as abstraction. This is very useful as the user will not know the unnecessary information in the abstraction process. We can use private keywords in the code so that other users cannot access the code.
#include <iostream>
using namespace std;
class Abstraction
{
private:
//private only this class can access the private variables
int a, b;
public:
void set(int x, int y)
{
a = x;
b = y;
}
void display()
{
cout<<"a = " <<a << endl;
cout<<"b = " << b << endl;
}
};
int main()
{
//object is created for the Abstraction class
Abstraction obj;
obj.set(50, 60);
obj.display();
return 0;
}
Output:
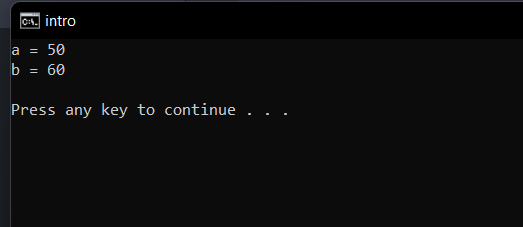
Explanation:
In the above code, we have created a class in which we have written code with some variables under private specifier. The variables in the private code are only accessible to the code in that class. In the main part of the code, we have set the value of the varialbes in the private section of the code using the function members.
Encapsulation
The encapsulation or combination of data and its methods or functions is the process by which they are combined. In oops, we can do encapsulation by making the function members in the class private and only the public function to access the private functions.
Polymorphism
Polymorphism means having many forms. Polymorphism helps the coder to create an overloading function. Polymorphism means we can create different functions that can perform similar operations.
#include <iostream>
using namespace std;
class Animal {
public:
void eat(){
cout<<"Eating...";
}
};
class Dog: public Animal
{
public:
void eat()
{ cout<<"Eating bread...";
}
};
int main(void) {
Dog d = Dog();
d.eat();
return 0;
}
Output:
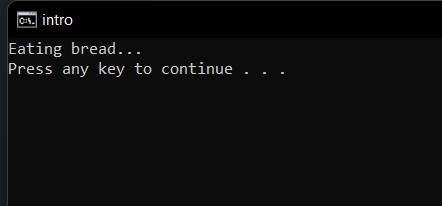
Explanation:
In the above code, we have created two classes, Animal and dog, and we have inherited the animal characteristics into the dog class. In the main class, when we created an object for the dog class, we called the function present in the dog class to eat().
Dynamic binding
In oop, function calls are resolved at runtime through dynamic binding. When a function call is made, runtime decisions are made about what code to execute.
Message Passing
The messages used in OOPS allow objects to communicate with one another. An object message consists of the object name, method name, and actual data. When objects communicate, information is passed back and forth between the objects.