Factorial of a Number in C++ using while Loop
What is a factorial?
The factorial of a number is the product of all the positive numbers less than or equal to n, indicated by n!
According to the standard for an empty product, the value of 0! is 1.
As an example,
1. 11! = 11 * 10 * 9 * 8 * 7 * 6 * 5 * 4 * 3 * 2 * 1 = 39916800
2. 7! = 7 * 6 * 5 * 4 * 3 * 2 * 1 = 5040
Program Code:
This computer code demonstrates how to get the factorial of an integer using a while loop in the C++ programming language.
#include <iostream>
using namespace std;
int main()
{
/* variables declaration */
int num_1, fact_1 = 1;
int n = 1;
cout << "Enter an integer to calculate the factorial of:" << endl;
cin >> num_1;
/* finding the factorial using the while loop */
while(n <= num_1)
{
fact_1 = fact_1 * n;
n ++;
}
cout << "Factorial of the given integer is : " << fact_1 << endl;
return 0;
}
Output:
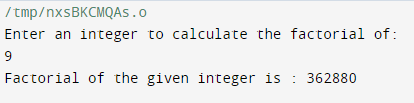
What is the process of the factorial method?
1. First, the machine reads the integer to determine its factorial from the user.
2. The value of "n" is then multiplied by the value of "fact_1" in a while loop.
3. The while loop runs until the value of "n" is less than or equal to "num_1." Finally, the provided number's factorial value is displayed.
The above code example is implemented in the following steps:
1. Let us suppose the user typed the number 9.
2. It assigns the values num_1 = 6, n = 1, and fact_1 = 1.
3. The loop then continues until the while loop's condition is met.
3.1 While loop condition is true, n <= num_1 (1 < 9 )
fact_1 = fact_1 * n (fact_1 = 1 * 1) So fact_1 = 1
n++ (n = n+1) So n = 2
3.2 While loop condition is true, n <= num_1 (2 < 9 )
fact_1 = fact_1 * n (fact_1 = 1 * 2) So fact_1 = 2
n++ (n = n+1) So n = 3
3.3 While loop condition is true, n <= num_1 (3 < 9 )
fact_1 = fact_1 * n (fact_1 = 2 * 3) So fact_1 = 6
n++ (n = n+1) So n = 4
3.4 While loop condition is true, n <= num_1 (4 < 9 )
fact_1 = fact_1 * n (fact_1 = 6 * 4) So fact_1 = 24
n++ (n = n+1) So n = 2
3.5 While loop condition is true, n <= num_1 (5 < 9 )
fact_1 = fact_1 * n (fact_1 = 24 * 5) So fact_1 = 120
n++ (n = n+1) So n = 6
3.6 While loop condition is true, n <= num_1 (6 < 9 )
fact_1 = fact_1 * n (fact_1 = 120 * 6) So fact_1 = 720
n++ (n = n+1) So n = 7
3.7 While loop condition is true, n <= num_1 (7 < 9 )
fact_1 = fact_1 * n (fact_1 = 720 * 7) So fact_1 = 5040
n++ (n = n+1) So n = 8
3.8 While loop condition is true, n <= num_1 (8 < 9 )
fact_1 = fact_1 * n (fact_1 = 5040 * 8) So fact_1 = 40320
n++ (n = n+1) So n = 9
3.9 While loop condition is true, n <= num_1 (9 <= 9 )
fact_1 = fact_1 * n (fact_1 = 40320 * 9) So fact_1 = 362880
n++ (n = n+1) So n = 10
3.10 While loop condition is false, n>num_1 (10 > 9)
It is exiting the while loop.
4. Finally, it produces the output seen below
The Factorial of 9 is 362880
5. As a result, the program code execution is complete.
Pseudocode
Pseudocode for the number's factorial
INPUT num_1
SET fact_1 = 1, n = 1
WHILE n <= num_1 DO
COMPUTE fact_1 = fact_1 * n
INCREASE n by 1
END LOOP
PRINT fact_1
Algorithm for computing the factorial of a number
Step 1: Start
Step 2: Read the user's input number.
Step 3: Declare and set the variables "fact_1 = 1" and "n = 1."
Step 4: Continue the loop until n <= num_1.
Step 5: Update fact_1 = fact_1 * n
Step 6: Increase the n value by 1 (iterate)
Step 7: Check if Step 4 is true continue else go to Step 9
Step 8: To obtain the factorial of a given integer, print fact_1.
Step 9: Stop