Containership in C++
In C++, it is possible to create an object in one class into another class, and that object is a member of another class. This relationship is known as a containership. We can also call it has_a relationship. The class containing the object in one class into another is known as the container class. The object that is present in the container class is known as a contained object, and the object contains another object known as a container object.
The iterator method can access all the objects available in the container class. The container class has the capacity to hold mixed and similar objects in its memory. A container class may be heterogeneous or homogeneous type. The main usage of the containership is to make the OOPs concept very easy for the C++ language.
The C++ standard container class has the following:
- Std::map: It is used to handle the array matrix.
- Std::vector: It provides some additional features like adding and removing the object from the container class.
- Std::string: It is the collection of characters in the array.
Syntax for the containership class:
class first {
.
.
};
class second {
first f;
.
.
};
Difference between Containership and Inheritance
Containership: Containership is a process in which one class uses the definition of another class. When a class gets the details of another class then the class is called containment, composition, or aggregation. The data member of the class becomes the object of the new class. Hence, the other class is known as containership. The composition is also called a has_a relationship.
Inheritance: Inheritance is a type of process in which a new class is derived from an old class. The reusability of the code is supported by inheritance. With the help of inheritance, some additional features can be added to a new class. Once a class is written, it is not possible to write it again. It is also called an is_a relationship.
Example 1:
#include <iostream>
using namespace std;
class first {
public:
void showf()
{
cout << "Hello, it is the output from first class\n";
}
}
class second {
first f;
public
second()
{
f.showf();
}
};
int main()
{
second s;
}
Output:
Hello, it is the output from first class
Explanation
In the above program, the second class has the object of the first class. This is another type of inheritance. This type of inheritance is known as a has_a relationship.
Example 2:
#include <iostream>
using namespace std;
class first {
public:
first()
{
cout << "Hello, it is the output from first class\n";
}
};
class second {
first f;
public:
second()
{
cout << "Hello, it is the output from second class\n";
}
};
int main()
{
second s;
}
Output:
Hello, it is the output from first class
Hello, it is the output from second class
Explanation
In this program, the second class is not inherited from the first class. But, we have the object of the first class in the second class. The default constructer of the first class is known as the first, and the second class's constructer is called the second.
Example 3:
#include <iostream>
using namespace std;
class first {
private:
int num;
public:
void showf()
{
cout << "Hello, it is the output from first class\n";
cout << "it's num value = " << num << endl;
}
int& getnum()
{
return num;
}
};
class second {
first f;
public:
second()
{
f.getnum() = 50;
f.showf();
}
};
int main()
{
second s;
}
Output:
Hello, it is the output from first class
it's num value = 50
Explanation
In this program, in containership, we can define the class only publically, not protected or private. They can get the value of the first class with the help of the getnum function.
Example 4:
#include<iostream>
using namespace std;
class cDate
{
int mDay,mMonth,mYear;
public:
cDate()
{
mDay = 1;
mMonth = 02;
mYear = 1998;
}
cDate(int d,int m ,int y)
{
mDay = d;
mMonth = m;
mYear = y;
}
void display()
{
cout << "day" << mDay << endl;
cout <<"Month" << mMonth << endl;
cout << "Year" << mYear << endl;
}
};
class cEmployee
{
protected:
int mId;
int mBasicSal;
cDate mBdate;
public:
cEmployee()
{
mId = 5;
mBasicSal = 30000;
mBdate = cDate();
}
cEmployee(int, int, int, int, int);
void display();
};
cEmployee :: cEmployee(int i, int sal, int d, int m, int y)
{
mId = i;
mBasicSal = sal;
mBdate = cDate(d,m,y);
}
void cEmployee::display()
{
cout << "Id : " << mId << endl;
cout << "Salary :" <<mBasicSal << endl;
mBdate.display();
}
int main()
{
cEmployee e1;
e1.display();
cEmployee e2(2,20000,11,11,1999);
e2.display();
return 0;
}
Output:
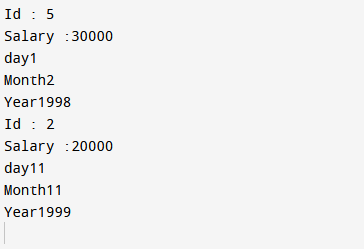