Substring in C++
A substring is a function in c++ that is imported from the string library. An element of a string is called a substring. A substring contains two parameters length and position of the string.
The syntax for substring:
String_name.substr(pos,length)
- Pos represent the position of the string from where the string is divided
- Length represents upto where the substring has to be created.
Example:
#include <iostream>
//imporint string.h to use substr()
#include <string.h>
using namespace std;
int main() {
string str1 = "example and tutorial website";
//substring will be created with a length of 3
string str2 = str1.substr(11, 3);
//substring will be created with a length of 6
string str3 = str1.substr(0, 6);
cout << "Substring starting at position 11 and length 3 is: " << str2 <<endl;
cout << "Substring starting at position 0 and length 6 is: " << str3;
return 0;
}
Output:
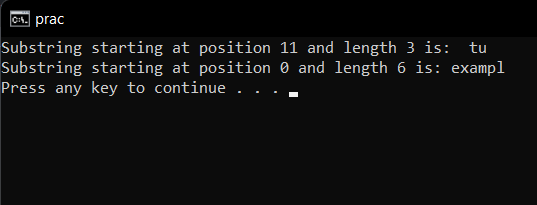
Explanation :
In the above code, we have given a string and using the substring function, we have given the position of the string from where we need to get the substring and length of the string. In the above case, the space between the words is also counted as a character.
Example:
#include <iostream>
//imporint string.h to use substr()
#include <string.h>
using namespace std;
int main()
{
string myString = "This sentence is for the example";
//the substring is created from the parent string
string subString = myString.substr(1, 9);
cout << subString;
return 0;
}
Output:
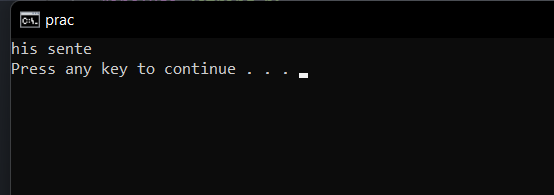
Explantion:
In the above example, we have given a string. “This sentence is for example,” using the substring keyword. We have created a substring by giving the parameter as the postion of the string, which is 1, and the length of the string as 9. Hence, we have created a substring of the given string.