Processing strings using std string stream in C++
A string class object called std: string stream is used to streamline into various variables, just as files can pour into strings. This class's things make use of a string buffer that holds a string of characters. A string object representing this string of characters can be retrieved.
Header Document
#include<stream>
1. Using std: string stream to stream an integer from a string
Using an input string stream object std: string stream from the header is one technique to spray a series. The extraction operator (>>) can be used to stream and store a series after an std:string stream object has been constructed. Until whitespace is encountered or the stream fails, the extraction operator will continue to read.
The std:string stream is illustrated below:
Example 1:
// C++ program to illustrate C++ program that displays std:: string stream
std::istringstream
#include <iostream>
#include <sstream>
#include <string>
using std::istringstream;
using std::string;
using std::cout;
// Driver Number
int main()
{
// Enter a string
string a("1 2 3");
// class of istringstream objects
istringstream my_stream(a);
// n-bit storage variable
int n;
// Count till there is a blank spot.
//is encountered
my_stream >> n;
// the number on paper
cout << n << "\n";
}
Output:
1
To ascertain whether the most recent extraction operation failed, the std::istringstream object can be utilized as a Boolean alternatively. This occurs if there are no more characters in the stream. We can stream the string once more if there are still characters in the stream.
The complete expression my stream >> n is a std::istringstream object that returns a Boolean, i.e., true if the stream is possible; otherwise, return false. The extraction operator >> writes the stream to the variable on the operator's right and returns the std::istringstream object.
The following describes how to use the std::istringstream in the manner described above:
Example 2:
// A C++ program that displays std:: istringstream
#include <iostream>
#include <sstream>
#include <string>
using std::istringstream;
using std::string;
using std::cout;
// Driver Number
int main()
{
// Enter a string
string a("1 2 3");
// class of istringstream objects
istringstream my_stream(a)
// n-bit storage variable
int n;
// examining the stream to determine its condition
// successful printing of outcomes
while (my_stream) {
// stream until space is reached
// encountered
my_stream >> n;
// if my stream contains data
if (my_stream) {
cout << "That stream was successful: "
<< n << "\n";
}
// Failure to print without it
else {
cout << "That stream was NOT successful!"
<< "\n";
}
}
return 0;
}
Output:
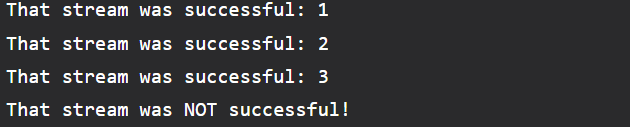
2. Strings of Different Types
The string in the examples is made up entirely of whitespace and int-convertible characters. The column can be used as shown below if it has mixed types or more than one data type in the stream.
The standard is illustrated below in this way:
1st Program:
// A C++ program that displays std:: istringstream
// when an integer follows a character in a string
#include <iostream>
#include <sstream>
#include <string>
using std::istringstream;
using std::string;
using std::cout;
// Driver Number
int main()
{
// Enter a string
string str("1, 2, 3");
// class of istringstream objects
istringstream my_stream(str);
// n-bit variable to hold the number
// and ch, the character
char c;
int n;
// until the input stream is legitimate, navigate
while (my_stream >> n >> c) {
cout << "That stream was successful: "
<< n << " " << c << "\n";
}
Cout << "The stream has failed."
<< "\n";
return 0;
}
Output:
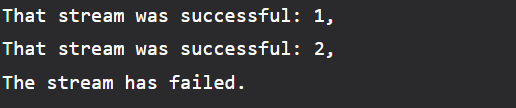
2nd Program:
// C++ example program for std:: istringstream
// tokenize the string with
#include <iostream>
#include <sstream>
#include <string>
using std::istringstream;
using std::string;
using std::cout;
// Driver Number
int main()
{
// Enter a string
string str("abc, def, ghi");
// class Object of istringstream
istringstream my_stream(str);
// To save the stream string
string token;
size_t pos = -1;
// Continue until the stream is legitimate.
while (my_stream >> token) {
// If "," is discovered, tokenize.
// the token string
while ((pos = token.rfind(','))
!= std::string::npos) {
token.erase(pos, 1);
}
// Tokenize the string, and print it.
cout << token << '\n';
}
}
Output:
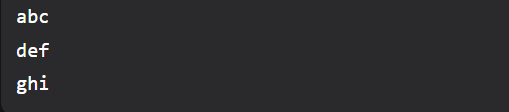