Difference between "int main( ) and int main(void)" in C/C++
int main( ) function
In the C/C++ programming language, int main() indicates a function that returns an integer at the end of the program execution.
In general, a value of '0' indicates that the program was successfully executed.
Syntax of “int main( ) function”
int main() {
/* statement-1*/
/* statement-2*/
return 0;
}
int main(void) function
int main(void) indicates that the function accepts “NO arguments.”
Difference between “int main( ) and int main(void)
In C++ programming language, there is no variation; both int main( ) and int main(void) are the same.
Both int main( ) and int main(void) operate effectively in the C programming language, but the int main(void) function is regarded as more technically effective since it explicitly indicates that the main( ) function can be called even without parameters.
When a function signature in the C programming language does not provide an argument, it indicates that the function may be called with any number of parameter values or without any parameter values.
Example of int main( ) function in C programming language
Here a function declared as ‘letters( )’ can accept any number of parameters.
/* Program Code Compiles and runs fine in C language */
#include <stdio.h>
void letters( )
{}
int main( ){
letters( );
letters(‘a’);
letters(“abc”);
letters(‘ab’,“abcd”);
printf(“abcd\n”);
return 0;
}
Output:
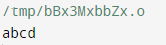
In the above program code, calling letters( ), letters('a'), and letters("abc") will not give any error because any number of arguments can be sent to the function without any parameters.
Example for int main( ) function in C++ programming language:
Here a function is declared as "letters( )."
/* Program Code Fails in compilation in C++ language */
#include <iostream>
using namespace std;
void letters()
{}
int main(){
letters( );
letters('a');
letters("abcd");
printf("abcd\n");
return 0;
}
Output:
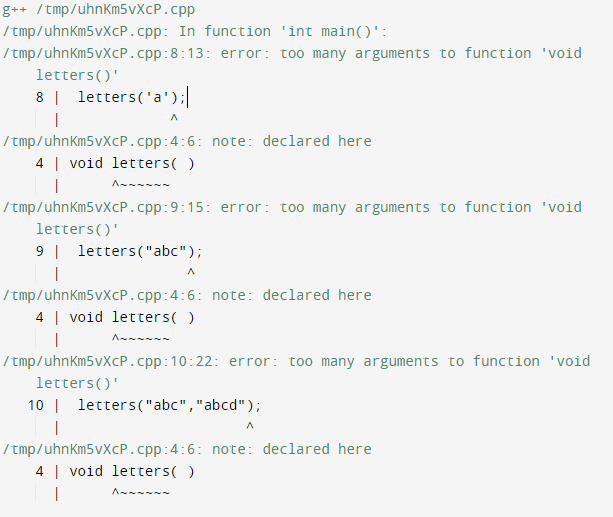
In the above program code, calling letters( ), letters('a'), letters("abcd") will give us some errors because we can't pass any number of arguments to the function "letters( )."
Example for int main(void) function in C programming language
Here a function is declared as "letters(void)." However, using letters(void) limits the function's ability to accept any argument and results in an error.
/* Program Code Fails in compilation in C language */
#include <stdio.h>
void letters(void)
{}
int main( ){
letters( );
letters(‘a’);
letters(“abc”);
letters(‘ab’,“abcd”);
printf(“abcd\n”);
return 0;
}
Output:
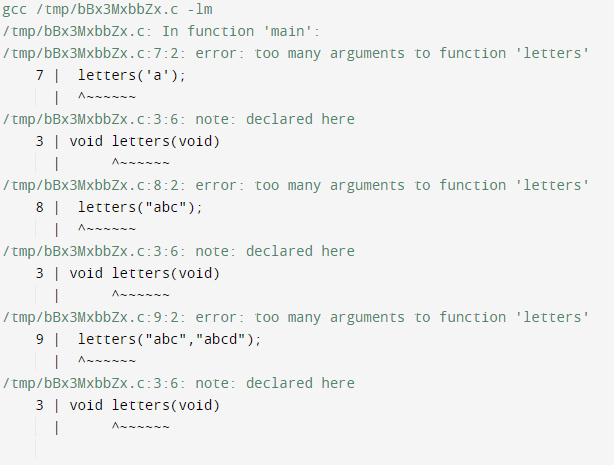
The preceding program code will throw an error since we used 'letters(void),' which implies we can't send any arguments to the method 'foo' as we could with 'letters( ).'
Example for int main( ) function in C++ programming language:
Here a function declared as "letters( )."
/* Program Code Fails in compilation in C++ language */
#include <iostream>
using namespace std;
void letters(void)
{}
int main(){
letters( );
letters('a');
letters("abcd");
printf("abcd\n");
return 0;
}
In the preceding program code, calling letters( ), letters('a'), letters("abcd") will give us some errors because we can't pass any number of arguments to the function.
“letters(void).” in C++ programming language.
Output:
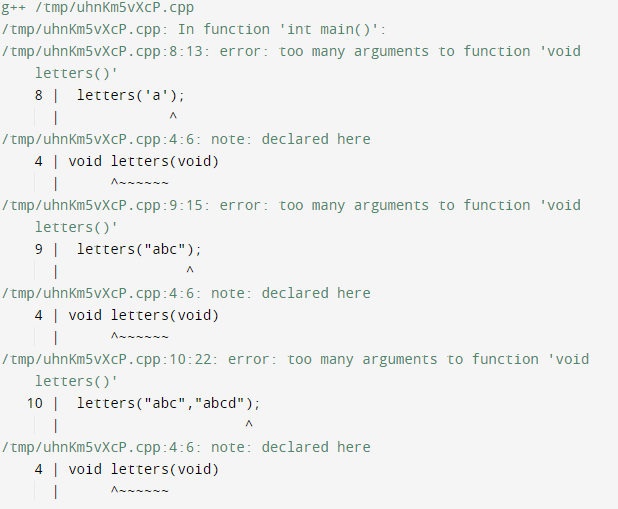
Some more differences between int main( ), main( ), void main( )
Just like any other function, main( ) is also a function with the additional property that the program code execution always begins with the 'main.' Its return types are 'int' and 'void.'
1. int main - 'int main' indicates that our function must return an integer at the completion of execution, which we do by returning ‘0’ integer at the end of the program. The standard for "successful program execution" is 0.
2. main - The undefined return type in the C'89 version is 'int' by default. In C'89, ‘main( )’ is identical to ‘int main.' However, in the C'99 version, this is not permitted, and so ‘int main( )’ must be used.
3. void main - The American National Standards Institute (ANSI) standard states "no" to the "void main( )" therefore, using it is incorrect. If this is the case, the 'void main( )' should be avoided.
As a result, the preferable method is “int main( )” method.
Conclusions
In C++ programming language, letters(void) and letters() are equivalent, but not in C language. The same is true for the 'main' function. If ‘main’ function does not accept any arguments, the preferable form is “int main(void).”