Method overriding in C++
What is method overriding?
Using the same function in derived class as their base class is referred to as function/method overriding in c++. Method overriding is an example of polymorphism. With it, you can provide a specific function implementation that its base class has already implemented. In order to access the overridden function, one must use the scope resolution operator "::"
Example:1
#include <iostream>
using namespace std;
class Animal {
public:
//defined a class eats in the class function.
void eat(){
cout<<"Eating...";
}
};
class Dog: public Animal
{
public:
//creating the same function as in the base class.
void eat()
{
cout<<"Eating bread...";
}
};
int main(void) {
//created an object for the dog class.
Dog d = Dog();
//When implemented eat function in the eat function, the dog class will be printed
d.eat();
return 0;
}
Output:
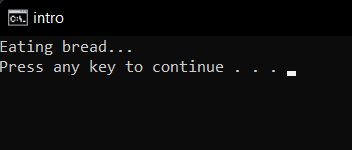
Explanation:
In the above code, we created a class Animal and declared the member function eat. Then we declared another class, Dog. We inherited the animal class properties into the Dog class. In the dog class, we created the same member function as in the aminal function. The function will be overwritten when we create the member function the same as the base class. This process is known as method overriding. We have created an object for the dog class in the main function. So when we printed the eat function using the dog object, the statement in the eat function in the dog class will be printed.
Example:2
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
//creating the same function as in the base class.
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
//created an object for the derived class.
//When implemented a print function in the derived class, then the print function in the derived class will be printed
derived1.print();
return 0;
}
Output:
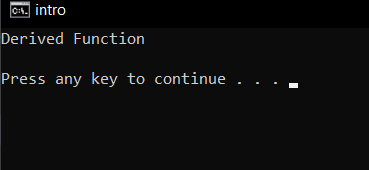
Explanation:
In the above code, we created a class Base and declared member function print. Then we declared another class Derived. We have inherited the Base class properties into the Dervied class. In the Derived class, we created the same member function as in the Base class. We have created an object for the Derived class in the main function. So when we printed the print function using the Derived object, then the statement in the print function in the Derived class got printed.