Free vs delete() in C++
Free vs delete() in C++
In this section, we will learn about the free() function and also create a C ++ program of the delete operator.
What is free() Function in C++?
In C++, the free() function is used to dynamically de-allocate the memory. It is essentially a library feature used in C++ and is specified in the header file stdlib.h. This library function can be used when either the pointers refer to the allocated memory using malloc() or the Null pointer.
Syntax of free() function
Assume that we defined a 'ptr' pointer, and now we want its memory to be de-allocated:
free(ptr);
The syntax above will de-allocate the pointer variable 'ptr' memory.
free() parameters
In the syntax above, ptr is a parameter within the function free(). The ptr is a pointer pointing towards the allocated memory block using malloc(), calloc() or realloc() feature. Even this pointer may be null or a pointer allocated using malloc but not referring to any other block of memory.
- If the pointer is null, then the function free() will do nothing.
- Unless the pointer is allocated using malloc, calloc, or realloc but does not point to any main memory, then this feature can trigger undefined behavior.
free() Return Value
The function free() refused to return any value. The key feature is memory free.
By an example, let’s understand.
#include <iostream> #include <cstdlib> using namespace std; int main() { int *ptr; ptr = (int*) malloc(7*sizeof(int)); cout << "Enter 7 integer" << endl; for (int k=0; k<7; k++) { // *(ptr+k) can be replaced by ptr[k] cin >>ptr[k]; } cout << endl << "user detail"<< endl; for (int k=0; k<7; k++) { cout <<*(ptr+k) << " "; } free(ptr); /* prints a garbage value after ptr is free */ cout << "Garbage Value" << endl; for (int k=0; k<7; k++) { cout << *(ptr+k)<< " "; } return 0; }
The code above demonstrates how function free() works with malloc(). First, we define the integer pointer * ptr, and then we assign the memory to that pointer variable using malloc(). Now, ptr points to the uninitialized 7-integer memory block. We use the free() function after allocating the memory to delete this allocated memory.
Output:
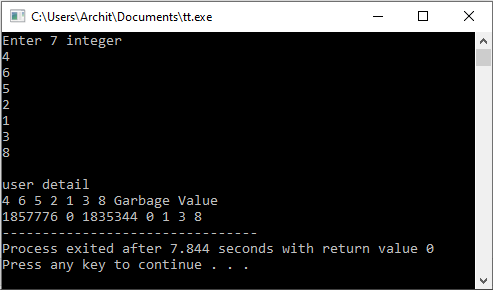
Example: malloc() and free()
#include <stdio.h> #include <stdlib.h> int main() { int s, k, *ptr, sum = 0; printf("Enter the amount of elements: "); scanf("%d", &s); ptr = (int*) malloc(s * sizeof(int)); if(ptr == NULL) { printf("Error! memory not allocated."); exit(0); } printf("Enter elements: "); for(k = 0; k < s; ++k) { scanf("%d", ptr + k); sum += *(ptr + k); } printf("Sum = %d", sum); // deallocating the memory free(ptr); return 0; }
Output:
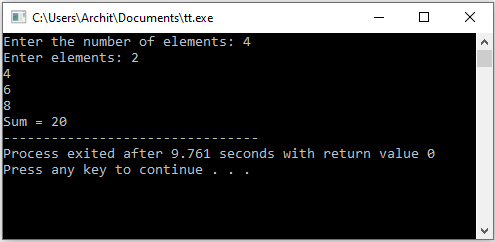
Example 3: realloc()
#include <stdio.h> #include <stdlib.h> int main() { int *ptr, k , s1, s2; printf("Enter size: "); scanf("%d", &s1); ptr = (int*) malloc(s1 * sizeof(int)); printf("Addresses of previously allocated memory: "); for(k = 0; k < s1; ++k) printf("%u\n",ptr + k); printf("\nEnter the new size: "); scanf("%d", &s2); // rellocating the memory ptr = realloc(ptr, s2 * sizeof(int)); printf("Addresses of newly allocated memory: "); for(k = 0; k < s2; ++k) printf("%u\n", ptr + k); free(ptr); return 0; }
Output:
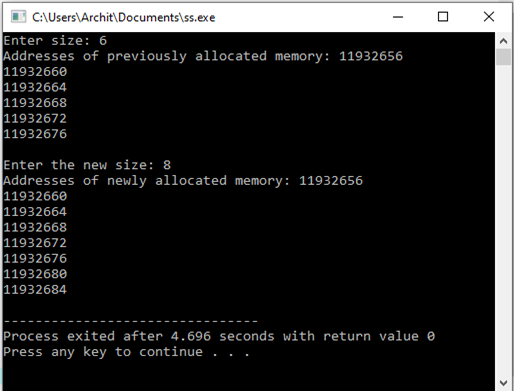
Delete Operator
It is an operator used in programming language for C++, and it is used to dynamically de-allocate the memory. This operator is primarily used for the allocation of many references using a new operator or NULL pointer.
Syntax
delete pointer_name
For example, if we use the new operator to allocate the memory to the pointer, then we want to erase it now. We use the following statement to delete the Pointer:
delete p;
Some significant delete operator related points are:
- Either it is used to delete the array or non-array objects which are allocated using the new keyword.
- We use delete [] and delete operator to delete the array or non-array object, respectively.
- The latest keyword allocates the memory in a heap, and we can assume the delete operator must still deallocate the memory from the heap.
- It does not destroy the pointer, but it destroys the value or the block of memory which the pointer points to.
Example of delete operator:
#include <iostream> #include <string> using namespace std; int main() { int *ptr = NULL; ptr = new int(); int *var = new int(11); if(!ptr) { cout<<"bad memory allocation"<<endl; } else { cout<<"memory allocated successfully"<<endl; *ptr = 6; cout<<"*ptr = "<<*ptr<<endl; cout<<"*var = "<<*var<<endl; } double *myarray = NULL; myarray = new double[6]; if(!myarray) {cout<<"memory not allocated"<<endl;} else { for(int j=0;j<6;j++) myarray[j] = j+1; cout<<"myarray values : "; for(int j=0;j<6;j++) cout<<myarray[j]<<"\t"; } delete ptr; delete var; delete[] myarray; return 0; }
Output:

We have illustrated the use of new and delete operators in the example of the code above. We used the "new" operator to allocate memory for a variable, arrays, and also initialize a value for another variable. Then we use delete operator to delete these entities.