Leap Year Program in C++
What is a Leap Year?
A solar year is the length of time that it takes for Earth to orbit the Sun - approximately 365.25 days. In a calendar year, we usually round the number of days to 365 as a standard. We add a day to our calendar in every four years to make up for the missing, partial day, and that year is a leap year.
How to find a leap year using Programming?
Generally, if any year is divisible by 4, it is a leap year. But a few years do not leap years and are divisible by 4. Generally, those years will end with zeros (Centurian years). Example; The year 2100 is the year which will be divisible by 4, but it is not a leap year similarly.
- So first, we need to check whether the entered year is a Centurian year.
- If it is a Centurian year, we need to check if it is divisible by 400.
- If it is divisible by 400, then it is a leap year.
- Then we need to write another statement to check if the entered year is divisible by 4 but not divisible by 100. If yes, then it is a leap year. Else it is not a leap year.
First, we will write a code to check if the year is divisible by 4 or not:
Note: This is not the final code
//The below code is written to check if the year entered is a leap year or not
//Please note that this is not the final code.
#include <iostream>
using namespace std;
// create the main function
int main(){
int a;
cout<<"Enter any year:"<<endl;
//Taking user input.
cin>>a;
//If it is divisible by 4, it is a leap year.
if(a%4==0){
cout<<"The entered year is a leap year"<<endl;
}
else{
cout<<"The entered year is not a leap year"<<endl;
}
}
Output:
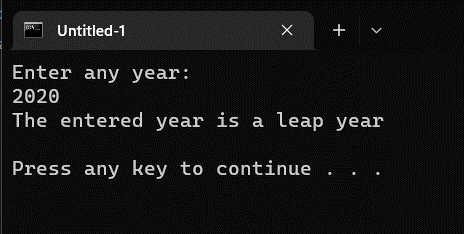
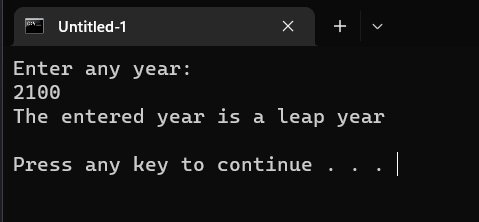
Explanation:
The above code is written to check whether the year is a leap year. We have taken input from the user using the cin statement in the code. Using the if statement, we have checked if the year is divisible by 4. If the year is divisible by 4, then the output will be the year is a leap year. Else the year will not be a leap year. In output1, we can see that 2020 is a leap year as 2020 will be divisible by 4, whereas in output2, we can see that the year 2100 is stated as a leap year which is not a leap year. So before checking if the year is divisible by 4, we need to check if the year is a Centurian year. If it is a Centurian year, then we need to check if it is divisible by 400.
Final code
//The below code is written to check if the year entered is a leap year or not
#include<iostream>
using namespace std;
int main() {
int year;
//Taking input from the user.
cout<<"Enter any year: "<<endl;
cin>>year;
//The first condition is to check if the year is a Centurian year.
if(year%100==0){
//If the year is a centurion year, then we need to check
//if it is divisible by 400 or not if divisible then it is a leap year
if(year%400==0){
cout<<"The entered year is a leap year"<<endl;
}
else{
cout<<"The entered year is not a leap year"<<endl;
}
}
//If the year is not Centurian, this block will execute.
else{
//We need to check if the non-centurion year is divisible by 4 or not
//If it is divisible by 4, the output will be a leap year.
if(year%4==0){
cout<<"The entered year is a leap year"<<endl;
}
else{
cout<<"The entered year is not a leap year"<<endl;
}
}
}
Output:
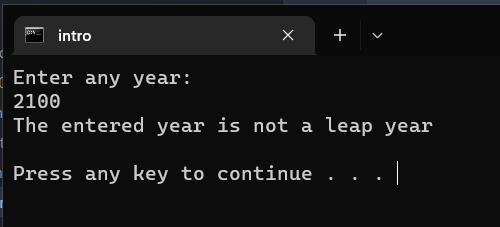
Explanation:
The above code is written to check whether the year is a leap year. We have taken the year input from the user using the cin statement in the main function. Then we need to check whether the year is a Centurian. We need to check if the year is divisible by 100 or not. If the year is divisible by 100, then we need to check whether the year is divisible by 400. If it is divisible by 400, then the centurion year is a leap year. If not, then the year is not a leap year. If the entered year is not a Centurian year, then we need to check if the year is divisible by 4. If it is divisible by 4, then it is a leap year. If not divisible by 4, then it is not a leap year.