Passing a Vector to a function in C++
A pointer is sent to the function when we feed it an array. However, there are two ways to pass a vector:
- Pass by Value
- Pass by Reference
A copy of a vector is made when a vector is handed to a function. As a result, any modifications performed to the vector in the process do not alter the original vector. This new copy of the vector is then used in the function.
For instance, changes performed inside a function are not reflected outside because the position has a copy, as we can see the following program.
Pass By Value
#include <bits/stdc++.h>
using namespace std;
// making a function that receives a vector of values as input
void func(vector <int> duplicate) { //The primary vector is duplicated in the secondary
// Allow me to multiply each even value in the vector by 1.
int l = duplicate.size(); // saving the vector duplicate's size in l
// dividing each duplicate even number by 1
for(int i = 0; i < l; i++){
if(duplicate[i]%2 == 0)
duplicate[i]--;
}
// showing the altered vector
cout<<"Modified vector in the function-->"<<endl;
for(int i = 0;i < l;i++)
cout<<duplicate[i]<<" ";
cout<<endl;
}
int main(){
vector <int> original = {1,2,3,4,5,6,7,8,10}; // the original vector's creation
func( original ); // utilising the func function
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++)
cout<<original[i]<<" ";
return 0;
}
Output:
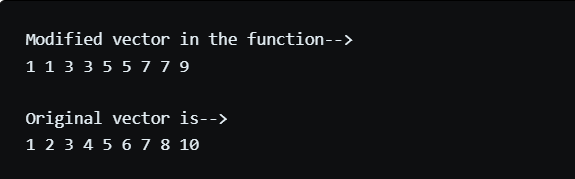
The original vector is kept intact, and its initial values are not modified by passing by value. However, when dealing with significant vectors, the passing method described above may also take a long time. So, passing by reference is a wise move.
Pass By Reference
A vector passed by reference causes the function to work with the original vector rather than a copy of it. As a result, any modifications made to the function's vector are also permanent or reflect themselves in the initial vector.
Additionally, because it doesn't make a new copy, passing by reference is faster and uses less time and space.
Reference Passing is required for following:
- To alter the initial vector.
- To speed up the code in specific circumstances. For instance, it is preferable to pass by reference when the function does not modify the vector to speed up the code.
Example:
#include <bits/stdc++.h>
using namespace std;
// making a function that allows the vector to be passed by reference
void func( vector <int>&duplicate) { // By reference, the initial vector is passed
// Allow me to multiply each even value in the vector by 1.
int l = duplicate.size(); // saving the vector duplicate's size in l
// dividing each duplicate even number by 1
for(int i = 0; i < l; i++){
if(duplicate[i]%2 == 0)
duplicate[i]--;
}
// showing the altered vector
cout<<"Modified vector in the function-->"<<endl;
for(int i = 0;i < l;i++)
cout<<duplicate[i]<<" ";
cout<<endl;
}
int main(){
vector <int> original = {1,2,3,4,5,6,7,8,10}; // the original vector's creation
func( original ); // utilising the func function
cout<<endl<<"Original vector is-->"<<endl;
for(int i=0;i<original.size();i++)
cout<<original[i]<<" ";
return 0;
}
Output:
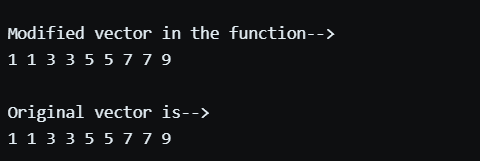
Because, we passed the original vector to the function by reference using the & operator in the code above, we can see that after modifying the vector in the function, the changes are also reflected in the original vector.
// Vectors can be passed by reference with restrictions using a C++ application that shows how.
#include<bits/stdc++.h>
using namespace std;
// This function cannot alter the fact because it is passed by constant reference
void func(const vector<int>&vect)
{
for (int i = 0; i < vect.size(); i++)
cout << vect[i] << " ";
}
int main()
{
vector<int> vect;
vect.push_back(10);
vect.push_back(20);
func(vect);
return 0;
}
Output:

Passing the function, a global vector
Any function in the program can access a global vector, which is a vector that is declared outside the primary role. We do not need to send the vector to the function since any process that accesses a global vector has the ability to alter the original vector.This indicates that the initial vector is utilized in this application rather than a clone.