C++ Fibonacci Series
What is a Fibonacci series?
A Fibonacci series or sequence is a very popular programming paradigm. The next element occurring in the N terms series is determined by the sum of the previous two elements. It simply means that the upcoming term will be easily figured out by applying the previous terms' logic. To be clearer with how the Fibonacci series work, let us consider an example.
For example: 1 2 3 5 8 13 …………N
Here, we can see that the previous elements starting from 1 then 2. We have assigned 1 and 2 as 1st and 2nd terms initially. Then we added 1 & 2, then 2 & 3 and so on till nth term.
Let us now discover different approaches of generating Fibonacci series till N terms.
- The novice approach
Consider the code given below:
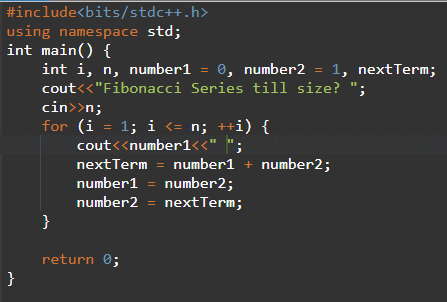
Output:
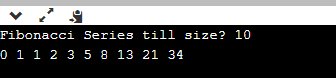
Explanation: In the above code, we are taking input from the sequence. We took number 10 so ten terms of the Fibonacci numbers are generated on the console. The logic behind is to simply loop over the terms till 10 and then consequently adding them using next term variable. It is quite easy to understand since the approach is traditional.
- The recursive approach
Consider the code given below:
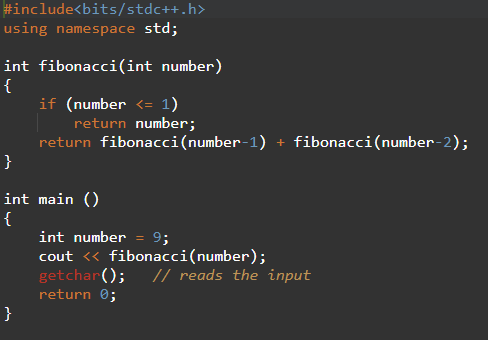
Output:
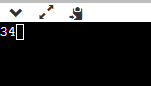
Explanation:
In this program, we expect to get the nth element at the position in the palindrome series. We are taking input from the user and have constructed a function named “fibonacci” where the parameter is the number that is being taken from the user and is compared using condition such that if the number is <=1. The Fibonacci series will be the addition of (n-1) and (n-2) terms. This is because we are gradually finding the next elements respective of their positions in the container.
Logic and formulations of Fibonacci series
The Fibonacci numbers are usually of the following sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144...N
Mathematically, a Fibonacci series is formulated using the following recurrence relation

Here,
Fn = Fibonacci sequence where n is the no. of terms.
F(n-1) = one element before the last occurrence .
F(n-2) = second-last element the last occurrence.
We always assign first and second element in the series as 0 and 1 and in the novice approach we will start our loop from 2 since the first and second position are already assigned as 0 and 1.
Let us now look at some other approaches associated with Fibonacci series.
- Dynamic Programming Method
Consider the code given below:
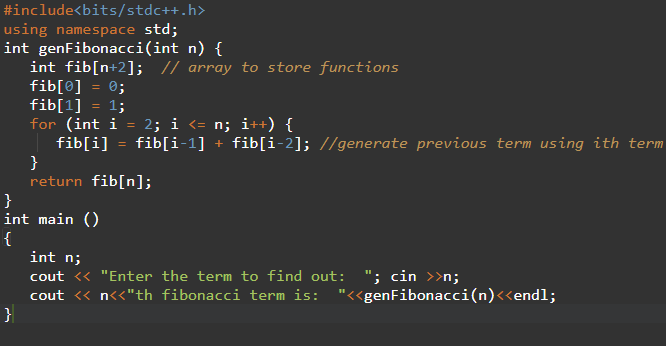
Output:
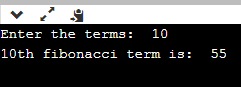
Explanation:
Here, sequence is generated using the summation of (n-1) and (n-2).
We have previously used recursive approach to solve the Fibonacci series but it is simpler in dynamic approach. It can store numbers in a table and we can easily generate the next sequential terms.