Function Pointer in C++
Definition:
A function pointer in C++ is the same as a usual pointer which is used to point some variables. Function pointers are used to point functions or say store the address of a function. A function pointer can be used to pass the pointer as a parameter for the other functions and we can call them easily.
Function Address:
We already know that a computer understands only the binary language. In high-level programming languages like C++, a compiler is a program that converts the code into machine understandable and stores it in the executable file. The copy of this executable file is stored in the RAM. When the CPU starts the execution from the main() method, it copies the file from RAM and not the original file.
This process of execution involves occupying some space in the memory. The source code may be a generic one or functional. All these executable source codes occupy space that has an address in RAM. The function pointer has the RAM address of the first instruction of a function.
Syntax:
int (*foo) (int);
OR
int (*FuncPtr) (int,int);
The above syntax is a function declaration. We know that C++ is a type-safe language and the functions are not simple as defining variables. Therefore, function pointers possess a parameter list and return type. In the above syntax, we have defined the return type and the name of the pointer. The function pointer uses the * operator. There are two integers which are the parameters that are passed with them. In simple words, the function pointer FuncPtr can point to any function which has two integer type parameters and a return value of integer type.
Storing function address:
The function pointer stores the address of the function. We do not need to call the function in the driver code, we just need to mention the function name so that we can easily get the address of the function.
Let us now look at some of the ways through which function pointer stores addresses of functions.
Example 1:
#include<bits/stdc++.h> using namespace std; int fool() { return 5; } int main() { cout << fool << '\n'; return 0; }
Output:
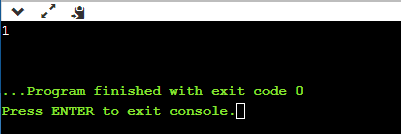
Explanation:
In the above code, we have declared a function named fool() which returns an integer value 5. To understand this, we need to be clear with the compilers. We have 1 as output in the online GCC compiler while in the other compiler the output may look like - 0xfd23d421.This is nothing but an unreadable memory address that is accessed using a function pointer.
Example 2: Indirect function calling
#include<bits/stdc++.h> using namespace std; int multiply(int x , int y) { return x*y; } int main() { int (*funcptr)(int,int); funcptr=multiply; int total=funcptr(4,7); cout << "Value of multiplication is :" <<total<<endl; return 0; }
Output:
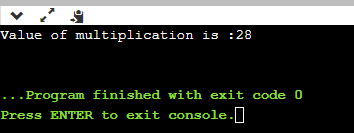
Explanation:
In the above code, we have used a function pointer to point the function multiply which takes two integer parameters x and y, and returns the multiplication. We have defined a function pointer funcptr that points to the function 'multiply'. The function multiply is passed to the funcptr. This is called an indirect function call. Another variable 'total' stores the value of multiplication which is indirectly passed with both the integer parameters.
The result is then printed on the console.
Note: There can be cases where the function is passed with more or fewer arguments. In such cases, the compiler may return 'too few' or 'too many argument' error.
Example 3: Passing function pointer as a parameter.
#include<bits/stdc++.h> using namespace std; void function1() { cout<<"Function1 is being called!"; } void function2(void (*funcptr)()) { funcptr(); } int main() { function2(function1); return 0; }
Output:

Explanation:
In the above code, we have defined two functions named 'function1' and 'function2'. The task is to use function is to pass function pointer as a parameter. To do this, we defined a function pointer 'funcptr' in function2 as a parameter and the pointer is called itself inside the function. When function1 is passed as an argument in function2 then the function pointer 'funcptr' points to function1 and executes the code inside it. By this action, function1 is called as a result and is then displayed on the console.
Note: The main() method calls the function2() in which the address of the function1 is passed. The function2 is calling function1 indirectly.
Points to Remember:
- The main task of the function pointer is to allow us to pass a function to a variable and store them for later use.
- A function pointer is used to point to any function with the same signature.
- Unlike other pointers, the function pointer does not de-allocate memory.
- Unlike other pointers, the function pointer points to code, not data.