Reverse String Word-Wise in C++
What is a reversed String?
Reversing the words of a sentence is called reversed string by words. The difference between the reverse a string and the reverse a string word-wise is that in reverse a string, we reverse every alphabet and in the reverse a word we reverse every word in a sentence. For example, take the sentence "I love to code" then the reverse string word will be code to love I.
Program
Example-1: Using Standard Template Library
Now lets us see how to write a c++ code for this using the standard templet library:
// This c++ program is written to reverse words of a string.
//As we are using string and input and output operations, we are using the bits/stdc++.h header files
// This header file will import all the files which are needed.
#include <bits/stdc++.h>
using namespace std;
// We are creating a function to reverse a string.
string reverseString(string str)
{
// First, we need to reverse a string using the built-in reverse keyword.
reverse(str.begin(), str.end());
//We need to add space to the End of the reversed string so that the last word will also be reversed
str.insert(str.end(), ' ');
//integer n will have a length of the string
int n = str.length();
int j = 0;
// We need to find the space and then reverse the string
//We are using the for loop to iterate through every letter;
for (int i = 0; i < n; i++) {
// If space is encountered
if (str[i] == ' ') {
reverse(str.begin() + j,
str.begin() + i);
// Update the starting index
// for the next word to reverse
j = i + 1;
}
}
// Remove spaces from the End of the
// word that we appended
str.pop_back();
// Return the reversed string
return str;
}
// Creating the main function
int main()
{
string str = "I love to code";
// calling the function
string rev = reverseString(str);
// Print the reversed string
cout << rev;
return 0;
}
//End of the code to reverse a string using the standard template library.
Output:
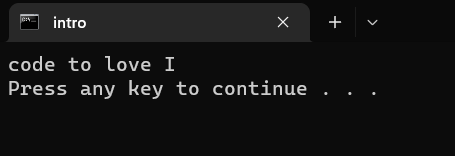
Explanation:
The above code demonstrates how to reverse string words using the standard template library. In the code, we have used the function to reverse the words. In the function, we used the reverse keyword to reverse the string. In the main function, we gave a string and passed it to the function by calling it. Then we added a space to the End of the reversed string so that we could even reverse the last word. Then we created a for loop to iterate through every letter of the string. During the iteration, if we find any space, we need to reverse that part of the word. To reverse the word, we have used the reverse keyword. Then we returned the word.
Example-2 Without Using Standard Template Library
Now let's see code how we can write the program without using the standard templet library:
// This c++ program is written to reverse words of a string.
//As we are using string and input and output operations, we are using the bits/stdc++.h header files
// This header file will import all the files which are needed.
#include <bits/stdc++.h>
using namespace std;
//function used to reverse a string
void reversed(string& s, int l, int r)
{
while (l < r) {
// Swap characters at l and r
swap(s[l], s[r]);
l++;
r--;
}
}
//function to reverse the given string
string reverseString(string str)
{
// Add space at the End so that the
// last word is also reversed
str.insert(str.end(), ' ');
int n = str.length();
int j = 0;
// Find spaces and reverse all words
// before that
for (int i = 0; i < n; i++) {
// If space is encountered
if (str[i] == ' ') {
//function call to our custom
// reverse function()
reversed(str, j, i - 1);
// Update the starting index
// for the next word to reverse
j = i + 1;
}
}
// Remove spaces from the End of the
// word that we appended
str.pop_back();
// Reverse the whole string
reversed(str, 0, str.length() - 1);
// Return the reversed string
return str;
}
// Driver code
int main()
{
string str = "I love to code";
// Function call
string rev = reverseString(str);
// Print the reversed string
cout << rev;
return 0;
}
Output:
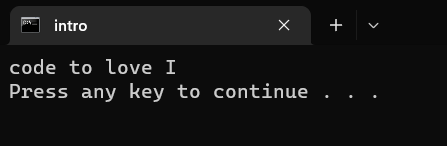
Explanation:
The above code is written to demonstrate how to reverse a string word. In the above code, we have created a function to swap the letters. We have also created another function that will loop through each letter. If we find any space, the swap function in the another function will be called by the End of the for loop, and the words will be reversed.