C++ Mutable keyword
C++ mutable keyword
Mutable class storage specifier in C++ Storage class specifiers in C are auto, register, static, and external. Typedef is also considered as a specifier of the storage class in C. C++ also supports all of those specifiers for storage class. In addition to this C++, adds an absolutely essential specifier for storage class whose name is mutable.
What is the use of mutability?
There is often a need to change one or more class/struct data members by the const method, even though you don't want the method to update other class/struct members. Using a mutable keyword, we can easily accomplish this task.
Consider this example where it may be useful to use the mutable. Suppose you go to the hotel and give an order to the waiter to bring some dishes of food. You suddenly decide to change the order of the food after you give an order. Suppose that the hotel provides the services for changing the ordered food and start ordering new food within ten min after the first order is issued. The order can't be canceled after 10 minutes, and the old order cannot be replaced with the new order. For details, see the code below.
#include <iostream> #include <string.h> using std::cout; using std::endl; class Customer { char name[25]; mutable char placedorder[50]; int tableno; mutable int bill; public: Customer(char* q, char* r, int b, int p) { strcpy(name, q); strcpy(placedorder, r); tableno = b; bill = p; } void changePlacedOrder(char* p) const { strcpy(placedorder, p); } void changeBill(int q) const { bill = q; } void display() const { cout << "Customer name is: " << name << endl; cout << "Product placed by customer is: " << placedorder << endl; cout << "table no is: " << tableno << endl; cout << "Total amount: " << bill << endl; } }; int main() { const Customer cus1("Peter Johenson", "Cold drink", 4, 250); cus1.display(); cus1.changePlacedOrder("chicken fry"); cus1.changeBill(420); cus1.display(); return 0; }
Output:

Watch the output of the above program closely placed order qualities and bill information. The main is modified from const feature since they are decided to declare to be mutable.
The mutable keyword is primarily used to change a given const object member. The feature passed to this pointer has become const when we declare a feature to be const. Adding mutable to a variable allows for a change of members by a const pointer. Mutable is especially useful if most members are constant, but a few need to be updated. Members of the data clarified as mutable may be updated even if they are the part of the object defined const. The mutable specifier cannot be used with names declared static or const or reference.
As an exercise, it predicts the output of two programs that follow.
// PROGRAM 1 #include <iostream> using std::cout; class Test { public: int t; mutable int p; Test() { t = 8; p = 15; } }; int main() { const Test l1; l1.p = 50; cout << l1.p; return 0; }
Output:

//PROGRAM2
#include <iostream> using namespace std; class Test { public: int w; mutable int k; Test(int e=0, int f=0) { w=e; k=f; } void setw(int e=0) { w = e; } void setk(int f=0) { k = f; } void disp() { cout<<endl<<"w: "<<w<<" k: "<<k<<endl; } }; int main() { const Test t(25,30); cout<<t.w<<" "<<t.k<<"\n"; t.k=200; //k still can be changed, because k is mutable. cout<<t.w<<" "<<t.k<<"\n"; return 0; }
Output:
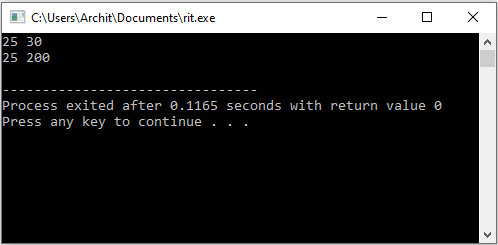