C++ Program For FCFS (First Come First Serve)
The most basic scheduling technique is FCFS, often known as "FIFO (First In, First Out)". In this procedure, the first one is utilized and executed first, while the second one begins only when the prior one has been finished and fully executed.
FCFS (First Come, First Serve) in the C++ programming language is a non-pre-emptive scheduling mechanism. The FIFO method prioritizes processes based on the sequence in which users request the processor. The CPU is allotted to the process that wants it first. This is simple to build, using a FIFO queue to manage the tasks.
As the process arrives, it is placed at the bottom of the queue. When a job is completed, the CPU deletes it from the queue and continues with the next task.
The First Come First Serve (FCFS) algorithm employs the following terms:
1. Completion Time (C.T.): The period of time taken until the process is finished.
2. Waiting Time (W.T.): The time difference between the burst time (B.T.) and the turnaround time (T.A.T.). Waiting Time (W.T.) = T.A.T (Time Around Time) – B.T (Burst Time)
3. Turn Around Time (T.A.T.): The time difference between the arrival time (A.T.) and the completion time (C.T.). Turn Around Time (T.A.T) = C.T (Completion Time) – A.T (Arrival Time)
We assumed arrival time = 0, thus completion time and turn-around time are the same.
Example
/* FCFS implementation code is written in the C++ programming language. */
#include<iostream>
using namespace std;
/* Function for calculating the total waiting_time for all the processes */
void find_Waiting_Time(int process[], int n, int b_t[], int w_t[])
{
// waiting time for first process is 0
w_t[0] = 0;
// calculating waiting time
for (int x = 1; x < n ; x++ )
w_t[x] = b_t[x-1] + w_t[x-1] ;
}
/* Calculating turn_around time using this function */
void find_TurnAround_Time( int process[], int n, int b_t[], int w_t[], int ta_t[])
{
/* adding b_t[x] + w_t[x] to calculate the turn_around time */
for (int x = 0; x < n ; x++)
ta_t[x] = b_t[x] + w_t[x];
}
/* Calculating avg_time time using this function */
void find_avg_Time( int process[], int n, int b_t[])
{
int w_t[n], ta_t[n], wt_total = 0, tat_total = 0;
/* Function for calculating the waiting_time of all the operations */
find_Waiting_Time(process, n, b_t, w_t);
/* Function for calculating turn_around time for all the processes available */ find_TurnAround_Time(process, n, b_t, w_t, ta_t);
/* Display all the procedures with all the available details */
cout << "Processes "<< " Burst time "
<< " Waiting time " << " Turn around time\n";
/* The total_turn_around time and the total_wait time should be calculated */
for (int x=0; x<n; x++)
{
wt_total = wt_total + w_t[x];
tat_total = tat_total + ta_t[x];
cout << " " << x+1 << "\t\t" << b_t[x] <<"\t "
<< w_t[x] <<"\t\t " << ta_t[x] <<endl;
}
cout << "Average waiting time = "
<< (float)wt_total / (float)n;
cout << "\nAverage turn around time = "
<< (float)tat_total / (float)n;
}
int main()
{
/* identifiers for all the processes */
int process[] = { 1, 2, 3};
int n = sizeof process / sizeof process[0];
/* burst_time of all the available processes */
int burst_time[] = {10, 5, 8};
find_avg_Time(process, n, burst_time);
return 0;
}
Output:
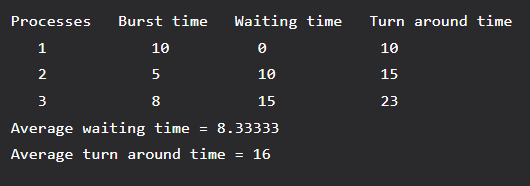
How is it Implemented?
- Enter the processes and their b_t (burst time).
- Determine the w_t (waiting time) for all the processes.
- Because the first process that arrives does not need to wait, w_t[0] = 0, i.e., the waiting time for process 1 is zero.
- Determine the waiting time for all the other processes, i.e., for process i:
w_t[i] = b_t[i-1] + w_t[i-1].
- Calculate turnaround time for all processes as (waiting_time) + (burst_time).
- Find the average waiting time by (total_waiting_time) / (no_of_processes).
- Similarly, the average turn-around time is calculated as (total_turn_around_time) / (no_of_processes).
Some Important points on First Come First Serve (FCFS):
- FCFS (First Come, First Serve) in the C++ language is a non-preemptive system.
- The average wait time for FCFS (First Come, First Serve) is insufficient.
- Resources cannot be used concurrently:
The results that in the Convoy effect (Imagine a situation with multiple I/O (input/output) bound processes and only one CPU bound process. The I/O (input/output) bound processes must wait until the CPU bound process receives the CPU. The I/O (input/output) bound process should have used the CPU for a while before using I/O (input/output) devices).