Sizeof() Operators in C++
sizeof() Operators in C++
The sizeof() operator in C++ defines the size of variables, constants, or data types. It is a unique operator that manipulates other operators and returns the size of the variables or constants in the compile time.
Syntax:
sizeof()(data_type)
Here, the data_type may be a constant, a variable, or any other structures or union.
Lets us see the coding illustration to visualize how sizeof() operator works.
Example 1:
#include<bits/stdc++.h> using namespace std; int main() { cout << "Size of Integer : " <<sizeof(int)<<endl; cout << "Size of float : " <<sizeof(float)<<endl; cout << "Size of double : " <<sizeof(double)<<endl; cout << "Size of char : " <<sizeof(char)<<endl; return 0; }
Output:

Explanation:
As already discussed, the sizeof() operator returns the size of any data type, constants, or any variable. In the above program, we have implemented the sizeof() operator to visualize how the sizeof() operator works in close integration with different data types.
Example 2:
#include<bits/stdc++.h> using namespace std; class Main { int x; }; int main() { Main y; cout << "Size of Main class is : "<<sizeof(y) <<endl; return 0; }
Output:

Explanation:
In the above, we have implemented the use of the sizeof() operator using class. We have defined a class 'Main,' with a data member 'x' of integer type. We have defined another integer variable in the driver code, 'b,' associate with class Main. Since we know that member 'a' is an integer, so in the driver code, it should print as 4 if we use the sizeof() operator. Therefore, 4 is printed on the console as the size of an integer variable in the class 'Main.'
Similarly, we can add more data members be it a char or a float. The sizeof() operator will return the total size of the variables or the data members assigned to some classes.
Lets us look how the sizeof() operator works for arrays.
Example 3:
#include <bits/stdc++.h> using namespace std; int main() { int array[]={101,202,303,404,505,606}; cout << "Size of the given array is : "<< sizeof(array)<<endl; return 0; }
Output:
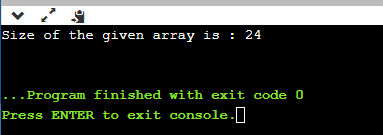
Explanation:
In the above code, we have used the sizeof() operator to calculate the total size of the linear data type i.e., array. The array in the above code is initialized with the 6 different values. As we know that an integer is of 4 bytes, so the sizeof() operator returns the value of the integer on the console as 24.
Example 4:
#include <bits/stdc++.h> using namespace std; int main() { int *pointer1=new int(11); cout << "size of pointer 1 : " <<sizeof(pointer1)<<endl; cout << "size of *pointer 1 : " <<sizeof(*pointer1)<<endl; char *pointer2=new char('b'); cout <<"size of pointer 2 : " <<sizeof(pointer2)<<endl; cout <<"size of *pointer 2 : "<<sizeof(*pointer2)<<endl; double *pointer3=new double(13.70); cout <<"size of pointer 3 : " <<sizeof(pointer3)<<endl; cout <<"size of *pointer 3 : "<<sizeof(*pointer3)<<endl; return 0; }
Output:

Explanation:
In the above code, we have used the sizeof() operator to illustrate its working with the pointers. Therefore, we have constructed three-pointers, namely 'pointer1', 'pointer2', 'pointer3' associated with three different data types int, char, and double(float). We have assigned values for these data types, respectively. Since we are very familiar with the size of different data types, the program returns their size concerning their original value and their associated pointer. The sizes are then displayed on the console as outputs.
Advantages of using sizeof() operator
The advantages are listed below:
- Acts as a savior
Whenever we want to know the number of elements in an array or the type of fashion in which they are arranged, the sizeof() operator acts as a savior as it automatically does that.
2. Helps in Memory allocation
It adds a great advantage in dynamic programming as it allows the programmer to dynamically allocate the size of the block of memory. It helps in memory allocation with enough prior knowledge to allocate enough memory. The memory is allocated in a particular machine which is difficult to do with other operators.
3. Versatile and Flexible
It is a versatile and flexible operator in C++, which helps in streamlining for the allocation of the memory.
Points to Remember:
- sizeof() operator is a compile-time unary operator that can be used to get the size of the operand.
- The result of the size of the result returned by the sizeof() operator is an unsigned integer value which depends on the nature of the data-type.
- Normally, the size of an object is the sum of its constituent data members. It is guaranteed that the sizeof() operator will never be smaller than that provided compiler keeps padding between data members to ensure alignment requirements of the platform.