C++ Algorithms
There are plenty of programming paradigms that are closely associated with the implementations of code and simulate them into a proper functional one. This is done with the help of some important functions which are present in a workbook called the library.
There are various types of functions present in the C++ Algorithms Library. Let us straightway look at the categories.
Min/Max:
Function | Description |
min | Return smallest element in the range |
max | Return the largest element in the range |
minmax | Return smallest and largest element |
min_element | Returns smallest element |
max_element | Returns largest element |
minmax_element | Returns both(smallest and largest) |
Merge:
Function | Description |
merge | merges two elements in sorted order |
includes | Searches inclusion of another range in sorted range |
inplace_merge | Returns two consecutive merged range |
set_union | Return union of two sorted ranges |
set_intersection | Returns intersecting sorted ranges |
set_difference | Return the difference of two ranges |
set_symmetric_difference | Returns symmetric sorted difference |
Sorting:
Function | Description |
sort | Sorts all the elements in a range |
is_sorted | Checks whether elements are sorted |
is_sorted_until | Checks range till range of sorting |
nth_element | Check nth sorts of elements in range |
partial_sort | Sorts range elements partially |
partial_sort_copy | Copies the sorted elements in range |
stable_sort | Maintains specific equivalent order while sorting elements in the range |
The above functions are immensely used while programming. Since there are hundreds of such functions present in the STL library, we'll be discussing some of them using a coding example.
Simplest sorting algorithm: Bubble Sort
Code:
#include<bits/stdc++.h> using namespace std; int main() { #include<bits/stdc++.h> using namespace std; int main () { int i, j,temp,pass=0; int a[10] = {1,2,3,4,5,6,7,8}; cout <<"Input list: \n"; for(i = 0; i<10; i++) { cout <<a[i]<<"\t"; } cout<<endl; for(i = 0; i<10; i++) { for(j = i+1; j<10; j++) { if(a[j] < a[i]) { temp = a[i]; a[i] = a[j]; a[j] = temp; } } pass++; } cout <<"Elements after sorting: \n"; for(i = 0; i<10; i++) { cout <<a[i]<<"\t"; } cout<<"\nNumber of passes taken: "<<pass<<endl; return 0; }
Output:
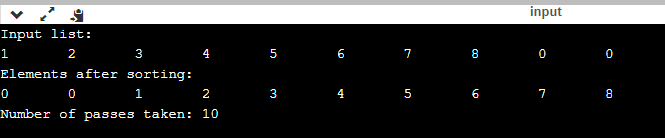
Explanation:
The above code is a simple depiction of a sorting technique popularly known as Bubble Sort. Bubble sort works on the following algorithm:
START Step 1: For i = 0 to N-1 repeat Step 2 Step 2: For J = i + 1 to N – I Repeat Step 3: if A[J] > A[i] Swap A[J] and A[i] [first loop ends] [second loop ends] END
The first loop is started from the 0th element and the next loop is iterated from an adjacent element. In the inner loop, we compare each associated element and swap them if they are found not in order. Additionally, at the end of each iteration performed by the outer loop, the heaviest or the greatest element is bubbled up at the end.
The comparison is done using passes. Passes are nothing but the swapped value that occurs while iterating through each element. In this case, 3 passes are occurring to sort the given items. With each pass the largest element is shifted last i.e. after the first pass, the largest element is shifted last. In second pass, the largest item is shifted at 2nd last position, and so on.
Min/Max Algorithm
Minmax function example:
#include<bits/stdc++.h>
using namespace std;
int main ()
{
auto values = minmax({100,222,324,401,500});
cout << "Minimum and Maximum values are: ";
cout << values.first << ' ' << values.second << endl;
return 0;
}
Output:
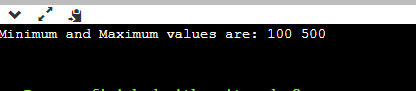
Explanation:
The above code is a simple depiction of the minmax function discussed earlier. It is present in the <algorithm> header file thus using <bits/stdc++> imports all the necessary files required. In the above code, we have defined a variable 'value' which stores all the elements given. These elements are passed as an argument to minmax function and the result is displayed on the console in the form of a minimum and maximum values.
Searching Algorithm:
Binary Search:
#include<bits/stdc++.h> using namespace std; int binarySearch(int array[], int x, int low, int high) { while (low <= high) { int mid = low + (high - low) / 2; if (array[mid] == x) return mid; if (array[mid] < x) low = mid + 1; else high = mid - 1; } return -1; } int main() { int array[] = {5,6,8,1,9,4,10}; int x; cin>>x; int n = sizeof(array) / sizeof(array[0]); int result = binarySearch(array, x, 0, n - 1); if (result == -1) cout<<"Not found"; else cout<<"Element is found at index: "<<result; }
Output:

Explanation:
Binary search works on the concept of following algorithm:
START Step1: x is compared to middle element. Step 2: Return the middle element if x matches. Step 3: If x is greater than middle element, it is shifted right. Step 4: Else x (if smaller than mid-element) is shifted left. END
The binary search algorithm is typically used to find an element present in a huge data-set. It can be compared to a search of a contact in the telephone directory where the directory is divided into two equal parts and the range in which the proximity of getting element is maximum, the range yet again divided to reduce time in traversing through each element present.