Vector resize() in C++
Vector resize() in C++
Vectors are called dynamic arrays and can automatically adjust their size when a component is added or deleted. This container is used for storage.
The function modifies the real content of the container by inserting or deleting the components from it. Thus it occurs,
- If at present the given value of n is less than the size, then additional elements are demolished.
- If n is greater than the current container size, then the next components are modified at the end of the vector.
Syntax:
vectorname.resize(int n, int val)
Parameters:
N – The container size is new, displayed in the number of objects.
Val -If this variable is specified, new features with this value are initialized.
Return value:
This method contains nothing.
Exception:
The only exception if this occurs is thrown by Bad alloc, if it keeps failing to reallocate.
The programs below show how the function works
The size of the vector container is lowered.
// resizing of the vector #include <iostream> #include <vector> using namespace std; int main() { vector<int> vect; // 5 elements are inserted // in the vector vect.push_back(5); vect.push_back(6); vect.push_back(7); vect.push_back(8); vect.push_back(9); cout << "vector before resizing:" << endl; // displaying the contents of the // vector before resizing for (int t = 0; t < vect.size(); t++) cout << vect[t] << " "; cout << endl; // vector is resized vect.resize(4); cout << "vector after resizing:" << endl; // displaying the contents of the // vector after resizing for (int t = 0; t < vect.size(); t++) cout << vect[t] << " "; return 0; }
Output:
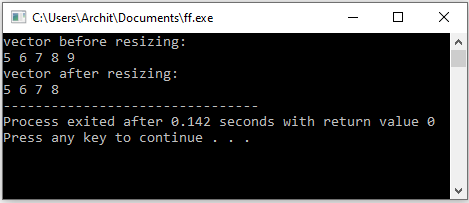
Size of the vector container is increased.
// resizing of the vector #include <iostream> #include <vector> using namespace std; int main() { vector<int> vect; // 10 elements are inserted // in the vector vect.push_back(1); vect.push_back(3); vect.push_back(5); vect.push_back(7); vect.push_back(9); vect.push_back(11); vect.push_back(13); vect.push_back(15); vect.push_back(17); vect.push_back(19); cout << "Contents of vector before resizing:" << endl; // displaying the contents of the // vector before resizing for (int a = 0; a < vect.size(); a++) cout << vect[a] << " "; cout << endl; // vector is resized vect.resize(12); cout << "Contents of vector after resizing:" << endl; // displaying the contents of // the vector after resizing for (int a = 0; a < vect.size(); a++) cout << vect[a] << " "; return 0; }
Output:
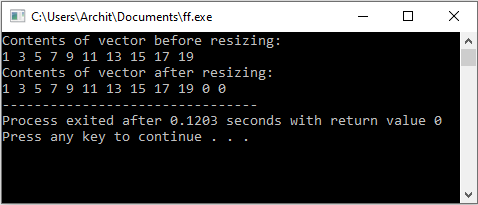
The size of the vector container is increased, and new elements are initialized with the specified value.
// resizing of the vector #include <iostream> #include <vector> using namespace std; int main() { vector<int> vect; // 11 elements are inserted // in the vector vect.push_back(2); vect.push_back(4); vect.push_back(6); vect.push_back(8); vect.push_back(10); vect.push_back(12); vect.push_back(14); vect.push_back(16); vect.push_back(18); vect.push_back(20); vect.push_back(22); cout << "Contents of vector before resizing:" << endl; // displaying the contents of // the vector before resizing for (int k = 0; k < vect.size(); k++) cout << vect[k] << " "; cout << endl; // vector is resized vect.resize(20, 9); cout << "Contents of vector after resizing:" << endl; // displaying the contents // of the vector after resizing for (int k = 0; k < vect.size(); k++) cout << vect[k] << " "; return 0; }
Output:
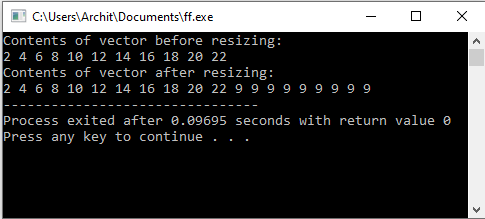