C++ Object Class
C++ Object Class Overview: C++ is a high-level programming language and an object-oriented programming language. An object-oriented language always has some properties of classes and objects. In this article, we would be looking forward to discussing object classes. But before going any further, let us discover what classes and objects are.
C++ Class
In C++, a class is the basic building block that leads to object-oriented programming. Class is user-defined data that has its data members and member functions. These members can be easily accessed by creating an instance of that class. In popular terms, the class is a blueprint for an object. A typical class syntax looks like the following:
class Animals { public: int category; //field or data member float population; //field or data member String name; //field or data member }
Note: Whenever a class is defined, no memory is allocated. But when the field or data members are initialized inside a class, memory is allocated.
C++ Object
In C++, an object is an entity of a class. It is also called an instance of the class. In simple words, an object is a real-world entity. The entities can be anything like cars, bars, mobiles, tablets, etc. An object always has a state and behavior. By state, we mean data, and by behavior, we mean functionality. An object in C++ typically looks like:
Animal a1 ; // creating an object of class Animal
Now that we have seen how class and objects look, let’s look at an example to understand how class and object work together.
Class and Object
#include <bits/stdc++.h> using namespace std; class Animals { public: int population; string name; }; int main() { Animals a1; a1.population = 2000; a1.name = "One-horned Rhino"; cout<<a1.population<<endl; cout<<a1.name<<endl; return 0; }
Output:
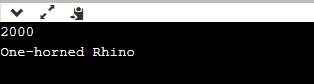
The above code is a classic example for implementing class and object in C++. We have defined a class Animals having data members population and name. We kept the data members public so that they are easily accessible. In the driver code, we have initialized these data members with some names and values and printed them on the console as output.
Class and Objects using Methods
#include <bits/stdc++.h> using namespace std; class Animal { public: int population; string name; void push(int i, string n) { population = i; name = n; } void show() { cout<<population<<" "<<name<<endl; } }; int main() { Animal a1; Animal a2; a1.push(21, "Zebra"); a2.push(22, "Horse"); a1.show(); a2.show(); return 0; }
Output:
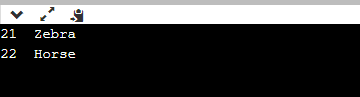
The above code demonstrates the activity of class and object by using methods. Methods are nothing but functions that are defined inside the class Animal for some specific purpose. Here, we have defined two methods, namely, push and show. Push method inserts or assigns the values, and the show method is used to display the inserted values. To do this, we have made two objects, a1 and a2, to be associated with both methods. These objects access the values through methods and display them on the console.
Creating multiple Objects for same class
#include<bits/stdc++.h> using namespace std; class SuperCar { public: string brand; string model; int year; }; int main() { SuperCar carObj1; // creating object for car1 carObj1.brand = "Lamborghini Aventador"; carObj1.model = "Sunflex S2"; carObj1.year = 2020; SuperCar carObj2; // creating object for car2 carObj2.brand = "Lycan HyperSport"; carObj2.model = "Agira"; carObj2.year = 1969; cout << carObj1.brand << " " << carObj1.model << " " << carObj1.year <<endl; cout << carObj2.brand << " " << carObj2.model << " " << carObj2.year <<endl; return 0; }
Output:

In the above code, we have defined a class SuperCar with their data members brand, model, and a year respectively. We created two objects for the class SuperCar namely car Obj1 and car Obj2 which are then initialized by the values for the brand, model, and year.
The benefit of creating multiple objects is to make the class reusable. This enables the program to set or get multiple values using only a single class definition. Also, the properties of this class are reflected by creating multiple objects. In a similar sense, we created two objects in the program and then displayed the values associated with the object class.
Advantages
- The benefit of creating an object class is that the properties of one class can be accessible to its multiple objects.
- The object class is that it increases code readability. The code is turned more easily to debug and implement.
- Object class is an object-oriented methodology that follows strict rules which are needed while writing complex systems.
- We can build programs from the standard working modules that communicate with one another, rather than having to start writing the code from scratch, which is a procedure-oriented approach. This leads to saving of development time and higher productivity.
- By creating an object class, we can easily manage different types of software complexity.