Structure and Class in C++
C++ has data types that the user defines; these groups of related sets of data members and member functions in C++ are referred to as classes or structures. In both cases, you need to declare member functions and attributes or data members. The main difference is in the default access specifier: By default, classes have private access, which provides encapsulation and data hiding, whereas structures are given public reference, thus more appropriate for convenient grouping of related data. Despite this difference, C++ programs can be made more modular and comprehensible when organized into classes and structures.
In this tutorial, we will discuss both structures and classes in C++
Structures in C++
C++ offers a data type that is user-defined, as the structure allows many varied types of data to be united and combined altogether under one name. It is declared with the `struct` keyword and then comes a block of members enclosed in curly brackets. Structures help in organizing and describing complex data by enabling related variables to be connected. A structure's members can be of multiple data types, and the dot ({.}) operator is used to fetch them. Given all members of a structure are publicly accessible by default, it is easy for outside parties to access the data.
Although member functions are less frequent than classes, C++ permits their inclusion in structures, which generally concentrate on data grouping. Structures are useful for a variety of C++ programming scenarios since they are frequently employed in situations where simplicity and public access to data are sought.
What is Structure?
In C/C++, a structure is a custom data type that allows different parts to be grouped into a single type. Users can create a compound data type by designing a structure that combines variables of different types into a single entity.
Syntax to create structure:
The struct keyword, the structure name, and a block of members encircled by curly brackets are the steps in the syntax for defining a structure in C++:
struct Sample{
// Members (variables of different data types)
int member1;
double member2;
char member3;
// ... additional members as needed
};
Structures in C++ can have two main types of members:
Data Members: These are standard C++ variables that help you to define a structure with various forms of variables. This ability allows several data kinds to be gathered under one structure.
Member Function: They are standard C++ functions that can be used when declaring structures. This means that C++ structures can also have functions aside from variables; this enhances structure adaptability by appealing to behavior alongside data.
Code:
#include <iostream>
#include <string> // Include the necessary header for using the string
using namespace std;
// Data Members
string bhavya; // Represents the name of a student
string place; // Represents the favorite place of a student
string animal; // Represents the favorite animal of a student
// Member Function
void college()
{
// Member function to print details of a student
cout << "Her Name is: " << bhavya << "\n";
cout << "Her fav place is: " << place << "\n";
cout << "Her fav animal is: "<< animal;
}
int main() {
// Example usage in main
bhavya = "Bhavya sree";
place = "Paris";
animal = "rabbit";
College();
return 0;
}
Output:

Declaring Structure Variables in C++:
Declaring a structure variable in C++ is similar to declaring other data types, where you use the name of this type then followed by some names.
Code:
// create a structure Tutorial;
struct Tutorial {
int obj;
double obj2;
// ... additional members as needed
};
int main() {
// Declare a structure variable
Tutorial instance1;
// Declare and initialize at one go.
Tutorial instance2 = {42, 3.14};
// Initialize member and set up another one – the second member
instance1.obj = 10; // Initialize the first member
instance1.obj2 = 5.5; // Initialize the second member
// Your code logic goes here...
return 0;
}
When declaring a variable in C++, the Use of the "struct" keyword is required, whereas in C, it is not.
Initialization of Structure Members:
It is not possible to initialize structure members during declaration. For example, a compilation error would occur when running the following C program. However, such initialization is considered permissible in C++11 and later versions.
struct Point
{
int var1 = 0; //There will be a compilation error as here we can't initialize members
int var2 = 0; //There will be a compilation error as here we can't initialize members
};
A compilation issue occurs when the provided code tries to initialize members right within a struct declaration. The reason is simple: memory allocation does not happen when a datatype is specified. Only when variables of that datatype are created is memory allotted.
Struct members in C++ can be initialized at declaration time, and the following C++ program runs error-free and successfully.
Example:
#include <iostream>
using namespace std;
// Define a structure with member initialization
struct Example {
int num1 = 0; // Default initialization with zero
int num2 = 54; // Default initialization with 54
};
int main() {
// Create an instance of the Example structure
Example s;
// Display the default initialized values of num1 and num2
cout << "Default values: num1 = " << s.num1 << ", num2 = " << s.num2 << endl;
// Modify the value of num2 to 20
s.num2 = 20;
// Display the modified values of num1 and num2
cout << "Modified values: num1 = " << s.num1 << ", num2 = " << s.num2;
return 0;
}
Output:

Structural elements can be initialized using curly braces {}. As an example, this is an acceptable initialization:
Example:
#include <iostream>
using namespace std;
// Define a structure with member initialization
struct Example {
int var1; // Define a member variable named var.
int var2;
};
int main() {
Example sample = {987, 453};
cout << "Initialized values: var1 = " << sample.var1 << " var2 =" << sample.var2;
return 0;
}
Output:

Accessing Structure Elements in C++:
The dot (.) operator allows accessing items in a structure.
An array of Structures in C++:
With C++, you can classify similar data into a collection so that its members are largely instances of the same predefined structure. A set of structures does this.
Example:
#include <iostream>
using namespace std;
// To construct a structure Hello
struct Hello {
int ans; // The member that will store the outcome of a calculation.
int ans1; // the member that holds the value of
};
int main() {
Hello a[3];
// Manipulating the array elements
a[0].ans = 1;
a[0].ans1 = 2;
a[1].ans = 3;
a[1].ans1 = 4;
a[2].ans = 5;
a[2].ans1 = 6;
// Display values
for(int i=0;i<3;++i) {
cout << "The Point " << i + 1 << ": ans1" << a[i].ans1 << endl;
}
return 0;
}
Output:
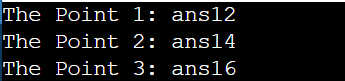
Structure Pointers in C++
It is referred to as structure pointers in C++, which means a type of variable that points towards the structured element. Additionally, structures can have pointers to primitive types. When using a pointer to access their respective members, the arrow -> operator is used rather than the dot.
Example:
#include <iostream>
using namespace std;
// Structure Point
struct Point {
int x;
int y;
};
int main() {
// Instantiate the Point structure
Point myPoint = {3, 7};
//Create a pointer that would point to my position, which was created as POINT.
Point* ptrToPoint = &myPoint;
Accessing structure members can be done using pointer and arrow operators.
cout << "Using Structure Pointer: " << endl;
//Use pointer to change its structure members
ptrToPoint->x = 10;
ptrToPoint->y = 20;
cout << "Modified values through Pointer: " << endl;
cout << "x = " << ptrToPoint->x << ", y = " << ptrToPoint->y << endl;
return 0;
}
Output:

A class is the foundation for C++ object-oriented programming. It is a user-defined type of data that contains not only the member functions but also data members. To access these characteristics and actions, you generate some instances of the class; think of your object's blueprint.
Now, we are going to use a car class as an example. All these automobiles have different names and brands, but every one of them has four wheels, a speed restriction, and a mileage range. In this case, the class is "Car," having its object characteristics – such as wheels, mileage, and speed limit.
The variables that hold data in a class are called Data Members, and the functions used to manipulate these values are referred to as Member Functions. It can determine the properties and behaviors of objects that would be created from a class by typing in some of its member functions or data members.
For instance, in the given case, saying we have a Car class, then data members would be like mileage and speed limit. The member functions, on the other hand, could be after breaking or changing speeds. Basically, a C++ class is an entity that allows the organized and modular level of programming by opening up instances for the state of an object (data members) as well as its behavior through member functions.
Example:
#include <iostream>
//Definition of the Car class
class Car {
Public:
// Data members
int wheels;
int speedLimit;
double mileage;
// Member functions
void applyBrakes() {
std::cout << "Brakes applied." <<std::endl;
}
void increaseSpeed() {
std::cout << "Speed raised." << std::endl;
}
void displayInfo() {
std::cout << "Car Information:"<<std::endl;
std::cout << "Wheels: " << wheels << std::endl;
std::cout << "Speed Limit: "<< speedLimit <<" km/h"<< std::endl;
std::cout << "Mileage: " << mileage << " km/l" << std::endl;
}
};
int main() {
// Instantiating a Car class instance
Car myCar;
// Initializing data members
myCar.wheels = 4;
myCar.speedLimit = 120;
myCar.mileage = 15.5;
// Using member functions
myCar.displayInfo();
myCar.applyBrakes();
myCar.increaseSpeed();
return 0;
}
Output:
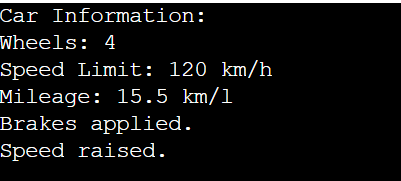
Class key aspects:
1. Declaration and Definition: The declaration defines the structure of a class in terms of its members' functions and data. Typically, it resides in a header file.
Definition: Specifies how data members and member methods function, incorporating the particulars of this class. 0 usually contains it in a source file.
2. Data Members: Data members are variables that hold attributes or information within a class.
By default, they are private and only accessible within a class. They define the state of an object.
3. Member Functions: The methods that define how a class acts are referred to as the member functions of a class.
They can include any set of processes or actions and work on the data members.
4. Access Modifiers: Class members' visibility can be managed using the public, private, and protected access modifiers.
- Public: Individuals can reach members from outside the classroom.
- Private: Only those in the class can access the members.
- Protected: Like private but gives derived classes access.
5. constructor and Destructor:
Constructor: A unique member function that is called upon object creation. Used to set the initial properties of an object.
When an object leaves its scope, a special member function called the Destructor is called. Utilized in cleaning operations.
6. Object Creation: Using the class_name object_name syntax, objects are formed as instances of a class.
The information and actions specified in the class are included in the objects.
Choosing Between Structures and Classes in C++
If not, by default C++ structures can be used to collect purely data without behavior and provide public access for these members. By default, classes use private data members to hide behavior and data. The classes promote code modularity and are effective in situations where a more intricate approach like inheritance or polymorphism is needed.
It depends on the individual needs of a certain program whether to use structures and classes. Structures are more straightforward for accessing data, whereas classes are generally better at encapsulation and higher-level functionality. Sometimes, structures and classes are used together in a well-rounded strategy.
Differences between Structure and Class in C++
In C++, there are only two minor dissimilarities in the way a structure and class functions. The main distinction is the default visibility of implementation details. Unlike a class, the structure does not hide implementation details by default; thus, everyone who uses it in the code can access Be's automatically. However, a class does not specifically allow the programmer direct access to it by default instead of hiding from him all information regarding its implementation. These important differences are briefly summarized in the table below:
Aspect | Structure | Class |
Inheritance in C++ | Structures in C++ do not provide a mechanism for one structure to inherit the properties or behaviors of another. | Classes in C++ support inheritance, allowing a new class (derived class) to inherit properties and behaviors from an existing class (base class). |
Accessibility Control | No access control keywords are required. | Access control keywords (public, private, protected) are often used to manage visibility. |
Default Member Visibility | Members (variables and functions) have public visibility by default. All members declared within a structure are accessible without any access specifier. | Members have private visibility by default. If access specifiers are not explicitly provided, all members are private. |
Member Initialization in C++ | Members cannot be initialized in the structure definition. | Members can be initialized in the class definition. |
Member Functions in C++ | Structures in C++ do not support the inclusion of member functions. A structure typically serves as a container for data members but cannot encapsulate behavior. | Classes, being a fundamental part of object-oriented programming (OOP), support the inclusion of member functions. |
Initialization of Objects | Objects are initialized without using a constructor. | Objects can be initialized using constructors. |
Object Instantiation | Used for lightweight data containers. | Used for complex data structures and OOP. |
Typical Use Cases | For basic data organization | For modeling entities with data and behavior. |
Compile-Time Compatibility | Maintains backward compatibility with C. | Introduces additional features for OOP in C++. |
Declaration Keyword | It is declared using the "struct" keyword. | It is declared using the "class" keyword. |
Key points in the above differences:
Members of a structure are, by default, public, enabling external access without explicit access specifiers, but members of a class are private by default, limiting direct external access.
Code 1:
// C++ Program to demonstrate that
// Members of a class are private
// by default
#include <iostream>
using namespace std;
class Sample {
// x is private
int x;
};
int main()
{
Sample s;
// compiler error because x
// is private
s.x = 20;
return s.x;
}
In the above code, there will be a compilation error.
Code 2:
// C++ Program to demonstrate that
// members of a structure are public
// by default
#include <iostream>
using namespace std;
struct Test {
// x is public
int x;
};
int main() {
Test t;
t.x = 20;
// Works fine because x is public
cout << "Value of x: " << t.x;
return 0;
}
Output:
