Copy constructor
Let's start by learning what a constructor is before diving into the copy constructor in C++.
What is a constructor?
A constructor is a particular type of class member function that configures the objects of a given class. In C++ also, a constructor is a special member function with the exact name as its class that is used to set certain valid values for an object's data members. When a class object is generated, it gets executed by itself. The constructor is solely limited by the fact that it cannot have a return statement or be void. It's because the constructor is used to initialize values and is called automatically by the compiler. The constructor’s name is generally different from various other member functions of the present class, which is the same as the name of the class. In C++, ctorz is an acronym for constructor.
Example :
Calculating the total of given numbers using a constructor.
#include<iostream>
using namespace std;
class total {
private: int num,ans;
public: totalsum(){
ans=0;
cout<<"enter number of limit = ";
cin>>num;
for(int j=1; j<=num; j++){
ans+=j;
}
cout<<"Sum of given numbers = "<<ans;
}
};
int main() {
total obj1;
return 0;
}
Output :
enter number of limit =4
Sum of given numbers = 10
Explanation –
In the preceding example, we constructed an object obj1 that automatically runs the totalSum() function.
The sum of the first four nos, i.e. 1 to 4, is discovered.
totalSum() function is the constructor here, which is automatically executed when an object gets produced. It further returns the answer 10, which is the sum of all given numbers.
What are Copy Constructors in C++ :
These are constructors that accept an object as an input and are used to replicate the entries of data members from one object to another. All classes have a default Copy Constructor provided by the compiler.
Basically, if the class members are all basic types like scalar values, you may use the compiler-generated copy constructor instead of writing our own. We'll need to write a custom copy constructor if our class needs more complicated initialization. If a class member is a pointer, forexample, we must specify a copy constructor to allot new memory and copy the data from the pointed-to object. The copy constructor created by the compiler simply duplicates the pointer, ensuring that the resulting pointer points to the same memory address as the original.
Syntax :
ClassNames (const ClassNames &old_objct);
Example –
#include <iostream>
using namespace std;
class XYZ
{
public: int k;
XYZ (int j){ // parameterized constructor
k=j;
}
XYZ (XYZ &i){ // copy constructor
k = i.k;
}
};
int main ()
{
XYZ j1(100); // Called parameterized constructor.
XYZ j2(j1); // Called copy constructor.
cout<<j2.k;
return 0;
}
Output :
100
Explanation :
In the shown example, both parameterised and copied constructors are used. The object that contains the copy of variable 'k' is variable 'i'.
Here, XYZ class was created with integer k, having parameterised constructor j and copy constructor i. Then in the main function the parameterised and copy constructor are called and the copy constructor is printed as an output.
Why is it essential to use a copy constructor ?
A Copy Constructor in C++ can be used in the following situations when :
1. An object of the class is reinstated as a value.
2. An object of the class is provided by giving the values in the form of an argument to a method.
3. An object is generated from a class from which another object was already generated.
4. A temporary object is created by the compiler.
However, because the C++ Standard permits the compiler to optimise the copy away in certain instances, it is not assured that a copy constructor will be invoked in all of these cases.
When do we require a copy constructor that is user-defined in nature ?
If we don't specify our own copy constructor, the C++ compiler constructs one for each class that performs a member-wise copy across objects. Mostly, the copy constructor generated by the compiler functions as it is expected to. But at only those times when any object has pointers such as a file handler or a network connection, we are required to implement our own or we can say a user-defined copy constructor.
The Different Types of Copy Constructors :
It's a fantastic approach to create new object initializations, and programmers continue to utilise it. There are two sorts of copy constructors:
- Default Copy Constructors (Uses Shallow Copying)
- User Defined Copy Constructors (Uses Deep Copying)
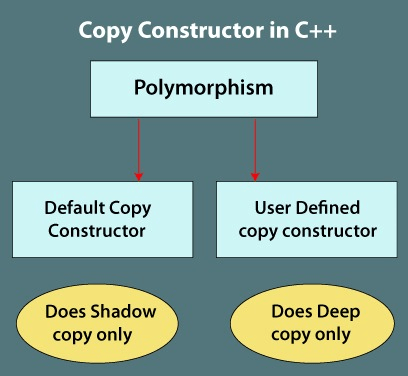
Fig. Types of C++ Copy Constructors
Below, we'll discover more about these two in depth —
Shallow Copy :
It's the process of making a replica of an object by replicating all of the member variables' data in their current state.
A shallow copy is only produced by the default Constructor.
A constructor that does not have any kind of parameters is known as a Default Constructor.
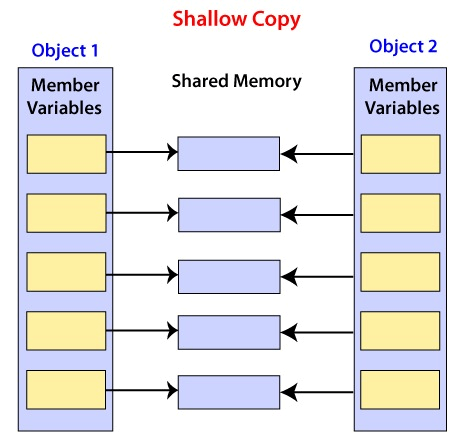
Fig. Shallow Copy
Let’s take the help of an example to understand this –
Example –
#include <iostream>
using namespace std;
class Op {
int x;
int y;
int *k;
public: Op() {
k=new int;
}
void input(int i, int j, int l) {
x=i;
y=j;
*k=l;
}
void show() {
cout<<"value of x:" <<x<<endl;
cout<<"value of y:" <<y<<endl;
cout<<"value of k:" <<*k<<endl;
}
};
int main() {
Op objt1;
objt1.input(4,8,12);
Op objt2 = obj1;
objt2.show();
return 0;
}
Output :
value of x:4
value of y:8
value of k:12
Explanation :
Both 'objt1' and 'objt2' will have the same input in the above code, and both object variables will point to the identical memory locations. Variables x, y and k were defined in class Op and their values got copied to variables i, j and l in the input function. Then the values of x, y and k were printed using default constructors with the help of objects objt1 and objt2. Changes to one object will have an impact on others. The user-defined constructor, which employs deep copy, will be used to overcome this problem.
Deep Copy :
First it creates memory for the copy dynamically, then copies the real value.
Both the items that must be duplicated and the objects that must be copied will have different memory addresses in a deep copy.
As a result, any modifications made to one will have no effect on the other.
A user-defined copy constructor uses this.
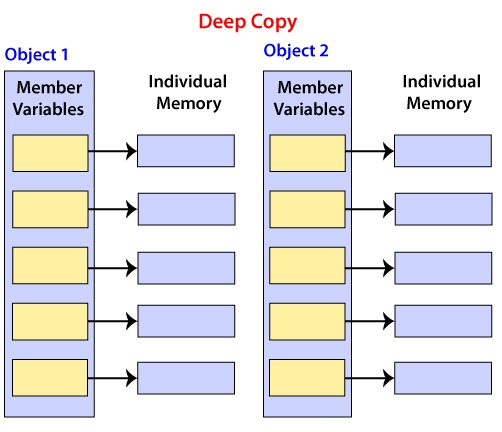
Fig. Deep Copy
Let’s take the help of an example to understand this –
Example –
#include<iostream>
using namespace std;
class Numbers {
private: int i;
public: Numbers(){}{
Numbers(int num) //default constructor // {
i=num;
}
Numbers(Numbers &z) {
i=z.i;
cout<<"copy constructor is invoked";
}
void show() {
cout<<"value of i:"<<i<<endl;
}
};
int main() {
Numbers Num1(50); // creating obj and assigning value to the member variable
Numbers Num2(Num1); // invoking the user-defined copy constructor
Num1.show();
Num2.show();
return 0;
}
Output :
copy constructor is invoked
value of i:50
Explanation :
Num1 and Num2 are the two items in the preceding case. The object 'Num2' is used to hold the value of object 'Num1'. 'Num1' accepts 50 as an input and sets the value to 'Num2'. Then, Num1 and Num2 will be at separate places.
Note : Any kind of changes made to one of the copy constructors won’t affect the other copy constructor.
Supplying reference to a copy constructor as a parameter :
When an object of the class is provided by giving the values, then the copy constructor is used. For replicating data, the constructo is a function of itself and in itself too. If we send a parameter by value to a copy constructor, the call to copy constructor will call copy constructor, resulting in a non-terminating series of calls. Thus, the compiler does not gives the permission to pass the arguments by a value.
How to make a copy constructor private:
It is very much possible to make a copy constructor private. When this copy constructor is made restricted in a class, then the objects in that class become non-reusable. This is advantageous, especially when our class contains any pointers in it or contains any resources that are dynamically allotted. In such cases, we may either create our own copy constructor or create a private copy constructor, resulting in compiler warnings rather than runtime surprises for users.
Difference between the Copy Constructor and the Assignment Operator :
Copy Constructor | Assignment Operator |
It's an overworked constructor. | It is a command. |
The new object is initialized with an existing object. | One item's value is allocated to another object, both of which already exist. |
Both objects utilize different or independent memory locations in this case. | Distinct variables point to the same memory address, but only one of these is used. |
If the class does not have a copy constructor, then it is created by the compiler for the user. | If the assignment operator that is in use is not overloaded, a bitwise copy will be performed. |
When a new object is generated with the help of an already existing element then the Copy Constructor is used. | When we need to allot an existing object of our program to a newly created object then we use the assignment operator. |