Abstraction in Python
What is meant by abstraction generally?
A very general notion of a thing or work is known as abstract. To be more precise, the process of having a brief idea but not a detailed overview of the content is known as Abstraction.
What is Abstraction in Python?
The process where the user is exposed only to the outer view but not the detailed structure or functioning of the program is known as Abstraction.
Abstraction can be defined as:
The process of giving access only to certain essential or required details to the user and hiding the unnecessary or unwanted details from the user so that the task that is supposed to be completed is not affected is known as Abstraction.
Abstraction is a process in which the complexity of the problem is hidden, and just the outwork is showcased.
So, let us consider a real-time example that describes abstraction.
Example:
When we play a game on a PC or a mobile phone, we are just aware of the controls, how to play, what makes you score more, what makes you move forward, what makes you move backwards, etc.
We are just allowed to know the game's outer view and the keys or controls required to play the game. We do not know how the game's functioning happens within the program, what program the game consists of, and how the keys are being enabled right after they are addressed.
All these features have been hidden from the user interface so that there will be no duplication and verifying that the main motive of the task is not being disturbed. In the same way, this is the actual process that takes place within a python program while undergoing abstraction.
Let us look forward to the advantages of using abstraction.
Advantages of using Abstraction:
- Redundancy or duplication of the programs can be easily avoided.
- Provides high security for the program or an application.
- No changes can be made in the internal parts of the system program as there will be no allowance or access to the user.
- Generates code reusability without any errors.
- Code optimization.
Abstraction in python can be implemented in two ways:
- By using Abstract class
- By using Interface
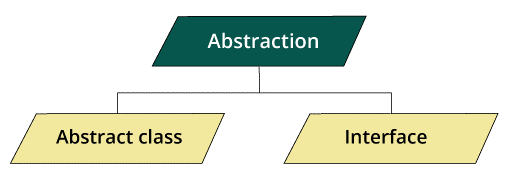
What is an Abstract Class and an Abstract Method?
Abstract class:
A class defined or declared with at least one Abstract method within its body is known as an Abstract class.
Abstract classes can be used when we want to implement large functions within the body. The subclass inherits the abstract class, and the abstract methods get their definitions in the subclass. This is how the flow of code processing occurs while using abstract classes and abstract methods.
In python, we cannot create an abstract class directly. To use abstract classes, we are supposed to import ABC, i.e., " Abstract Base Class ", from an inbuilt module " abc “.
Abstract Base Classes ( ABC ) :
The classes that act as intermediate classes in order to perform implementations within the other classes are known as " Abstract Base Classes ". It works similarly to the Application Programming Interface ( API ). We use plugins while implementing ABC. We can import Abstract Base Classes from the " abc module ”. We must use ABC to include abstraction in python. A method is said to be an abstract method when declared with the keyword " @abstractmethod ".
The syntax for creating an Abstract class:
from abc import ABC
class ClassName(ABC):
#statements
Let’s consider an example program:
Example program:
#importing abstract base class from abc module
from abc import ABC, abstractmethod
class Vehicles(ABC):
@abstractmethod
def noofwheels(self):
pass
class Car(Vehicles):
#overriding the abstract method
def noofwheels(self):
print("Car: I have 4 wheels")
class Cycle(Vehicles):
#overriding the abstract method
def noofwheels(self):
print("Cycle: I have 2 wheels")
class Bike(Vehicles):
#overriding the abstract method
def noofwheels(self):
print("Bike: I have 2 wheels")
class Auto(Vehicles):
#overriding the abstract method
def noofwheels(self):
print("Auto: I have 3 wheels")
class Truck(Vehicles):
#overriding the abstract method
def noofwheels(self):
print("Truck: I have 6 wheels")
obj1 = Car()
obj1.noofwheels()
obj2 = Cycle()
obj2.noofwheels()
obj3 = Bike()
obj3.noofwheels()
obj4 = Auto()
obj4.noofwheels()
obj5 = Truck()
obj5.noofwheels()
Output:
Car: I have 4 wheels
Cycle: I have 2 wheels
Bike: I have 2 wheels
Auto: I have 3 wheels
Truck: I have 6 wheels
Explanation:
Initially, " abstract base class " is imported from the " abc module “. A class “ Vehicles ” is declared with an abstract method which is represented by “ @abstractmethod ", and then a common method " noofwheels() ", is declared, which describes the number of wheels that the specified vehicle generally consists of. Thereafter, every class with different vehicles used the abstract method " noofwheels() “ to print separate statements.
The statements have been printed successfully. We've used abstract classes to optimize the code. The code might have been lengthier if the abstract class is not used here.