How to convert integer to float in Python
Python is an Object-Oriented high-level language. Python has an English-like syntax, which is very easy to read and write codes. Python is an interpreted language which means that it uses an interpreter instead of the compiler to run the code. The interpreted language is processed at the run time thus takes less time to run. Python is also interactive, so we can directly run programs in the terminal itself.
In this article, we are going to discuss how to convert an integer data type to a float data type. Datatypes are the categorization of the data items. Every value in Python has its datatype. The classification of data items to put in some category is called data types. As we know, Python is an object-oriented programming language, and everything in Python is an object. Data types represent classes, and the instance of these classes are called variables.
These are the built-in data types in Python:
- Set Types: set
- Mapping: dict
- Boolean: Bool
- Numeric Types: Integer, Float, Complex
- Binary Types: Bytes, Bytearray
- Sequence Types: List, Tuples
- Text Types: String
There are three types of Numeric Datatype in Python. Both Integer and Float are categorized into Numeric Data Types. Numeric data types take the numeric value. These data types are created when a value is assigned to them. For example, var = 12.2 will assign a memory block to var with the value 12.2. In Python, we can also delete the variable using the del keyword, thus freeing up the memory.
- Int: They are positive or negative integer objects numbers without any decimal. The size of the integer is limited by memory. We can also define the integers in other representations like binary, octal etc.
- Float: The float data type is used to store the decimal point negative and non-negative integers. We can also define float with scientific notation forex: number 1.2e2 represents 120.0.
- Complex: Complex numbers are those which has an imaginary number in them. These numbers cannot be represented in the number line. Complex numbers are represented with a + ib where i is (-1)1/2.
Number Type Conversion
Type conversion functions are defined in Python to convert data types. In Python, we can convert one data type to another. Conversing data types is very useful in day to day life or while solving coding questions.
We can do Data Conversion in two ways in Python, either to let the compiler decide itself the data type of the variable or to specifically tell the compiler to convert the data type of a variable to which data type. The former is called the Implicit Type Conversion, and the latter is the Explicit Type Conversion.
Let us discuss these types of conversions in detail:
Implicit Data Type Conversion
Implicit Type conversion which is also known as Coercion is when converting data type take place during compilation time and is done by compiler itself for us. To get the better understanding of the topic let us see an example below:
x = 10
print("Data type of x is: ",type(x))
y = 10.5
print("Data type of y is: ",type(y))
z = x + y
print("Sum of x and y is: ",z)
print("Data type of z is: ",type(x))
Output
Data type of x is: <class 'int'>
Data type of y is: <class 'float'>
Sum of x and y is: 20.5
Data type of z is: <class 'int'>
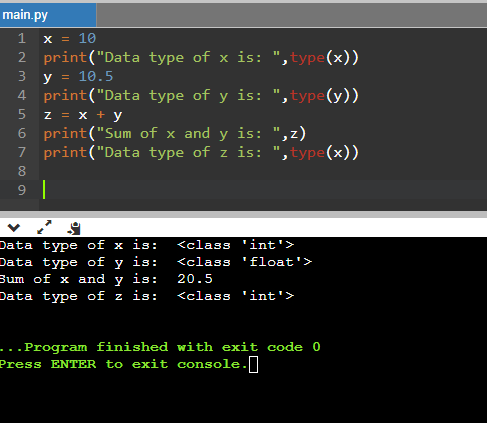
- In the above code, we have defined two variables, x and y.
- In this code, x is of data type int, and y is of data type float. We can use the in-built type() function to check the data type in Python.
- Then we have added the two variables x and y and storing the sum in z.
- The datatype of z is of type float. This is called the implicit data type conversion.
You might have a question that why the data type of z is not an integer. This is because of the concept of type promotion in computer science. The compiler converts the data into the data type, which does not cause the loss of information. Python always converts the smaller data type to a larger one. So the data type of z is not defined as an integer because it will cause the loss of information since integers cannot hold decimal numbers.
Explicit Data Type Conversion
In Explicit Type Conversion, the user's data type conversion is done manually as per the requirements. Explicit Conversion is also referred to as Type Casting because the user casts the datatype to which he wants to convert to. To do the type conversion we use some pre-defined functions like float(), int(), str(), etc.
The general syntax of explicitly converting data type is:
(required_data_type)(expression)
With the explicit data type conversion, there is a risk of loss of information since we are forcing a data type to a variable.
The data types are classified into two data structures Primitive and Non-Primitive.
Primitive Data Structure
These are the primary data structure and are the building blocks in Python. They are the primary data structure for data manipulation.
Some forms of explicit Conversion in the primitive data structure are described below:
- Int(): It is used to convert the data type to integer.
- Float(): It is used to convert any data type to a floating integer.
- Hex(): Hex is used to convert an integer to a hexadecimal string.
- Oct(): Oct is used to convert integer to octal decimal.
- Ord(): It is used to convert a data type to a string.
Let us see some examples of type conversion.
Integer to Float:
Num1 = 123
Num2 = 324
Num3 = float(Num1+ Num2)
Print(Num3, type(Num3))
Output
447.0 <class 'float'>
Addition of String to Integer
num = 123
str = "321"
print("Data type of int:",type(num))
print("Data type of str:",type(str))
str = int(str)
print("Data type of str after Type Casting:",type(str))
sum = num + str
print("Sum of num and str:",sum)
print("Data type of the sum:",type(sum))
Output
Data type of int: <class 'int'>
Data type of str: <class 'str'>
The data type of str after Type Casting: <class 'int'>
Sum of num and str: 444
The data type of the sum: <class 'int'>
Non-Primitive Data Structure
Non-Primitive data structure stores the collection of primitive data structures. The non-primitive data structures are Lists, Tuple, Dictionaries, etc.
Some forms of explicit Conversion in the non-primitive data structure are described below:
- Tuple(): Tuple is used to convert a data type to tuples.
- List(): List is used to converting a data type to lists.
- Set(): Set is used to convert a data type to sets.
- Dict(): Dict is used to convert a data type to dictionaries.
#Data Type Conversion to Tuple, Set and List
str = 'javatpoint'
# converting to tuple
tup= tuple(str)
print ("After converting string to tuple : ",end="")
print (tup)
# converting to set
st = set(str)
print ("After converting string to set : ",end="")
print (st)
# converting to list
lst = list(str)
print ("After converting string to list : ",end="")
print (lst)
Output
After converting string to tuple : ('j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't')
After converting string to set : {'p', 'j', 'i', 'a', 't', 'v', 'n', 'o'}
After converting string to list : ['j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't']
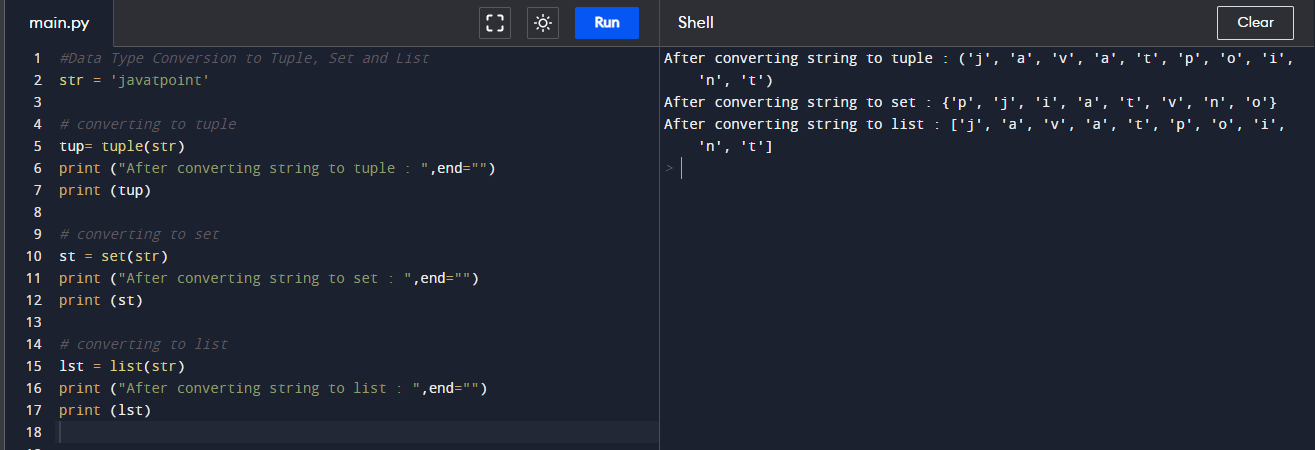