Python NewLine
In python, a new line character denoted by "\n" is known as an escape character or escape sequence, and it will force the cursor to change its position to the
next line from the beginning on the screen. This will result in the text to a new line.
Here we will learn about:
- Identification of character in a newline in python.
- This newline character will be used in strings and print statements.
- How to print statements without adding newline characters in them.
There are other escape sequences. They are:
- \t - It creates a horizontal tab.
- \\- It will insert a backslash character.
- \"- It will insert a double quote character.
NewLine Character in Python
As we all know, in python, the newline character is indicated by "\n".
It consists of two characters:
- The first one is backslash "\".
- The second one is a letter that is "n".
If we see this newline character in a string or a program, it means that the current position of the cursor and the current line will end at that particular point, and a new line will be started at the same time. Thedefault value of the end parameter of the built-in print function is "\n". This shows that a newline character is appended to the string.
Example Program in C
#include<stdio.h>
int main()
{
Printf("Hello! Welcome!\n");
Printf("I was learning python");
Return 0;
}
OUTPUT
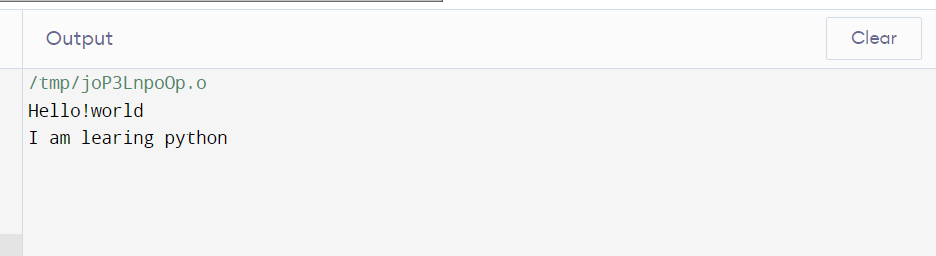
The program newline character "\n" shows that the second printf () statement is returning on the next line. This newline character is used where there is no misunderstanding of the program and will be as clear as anything.
Example Program in Python
print("Hii, Welcome\n")
print("This is for you")
OUTPUT

This shows from the above program that" \n" will return a new line or next line in an output of a program.
NOTE: When two newline characters, that is, two "\n" characters one by one in the same line, will return a blank line, and an output will be generated.
Example Program
print("Nice to meet you\n\n")
print("same here")
OUTPUT

The above output shows that there is a blank line between two sentences. This shows that two "\n\n" characters will result in a blank line.
We can also use the "\n" character in f-strings.
For example,
print(f"Hello\nWorld")
OUTPUT

We observed from the above sample program that the "\n" character is also used in f-strings. This also produces a new line after being given "\n".
We can also use any number of print statements for printing statements in a new line. We can change the default behavior of the end parameter "\n" by customizing its value which belongs to the print function shown below.
For example,
print("user")
print("password")
OUTPUT

From the above example, we observed that by using two print functions, it would print both statements in two separate lines without using the newline character "\n".
When we customize the value of the end parameter and set it to " ". Adding " "to the end parameter will create a space at the end of the string rather than using a newline character "\n". So, the output of two print statements will be displayed in the same line.
For example,
print("Hii! Welcome", end=" ")
print("World")
OUTPUT

This example shows us that by using the end parameter and setting it to " ", we get the result in the same line as we used two print statements.
We can use this type end=" "to print a sequence of values in a single line. Below is an example of how it works.
Program for above type
for i in range(7):
if i<6:
print(i,end=" ")
else:
print(i)
OUTPUT

From the above program, by using the end parameter and setting to " "it prints a sequence of values in a single line.
NOTE: We added a conditional statement to avoid commas for the sequence's last value.
NewLine Character Techniques
Here we will learn different ways to add a newline character in python "\n" to print the data output.
Technique 1: Adding NewLine Character in a Multiple line String
In python, a multiple-line string must provide a good way to represent multiple strings sequentially. The example below will let you know by adding a newline character "\n" to a multiline string.
Syntax
Str = '''string1\nstring2\n.....\nstringN'''
We should use the newline character "\n" before every string we need to display in output as a newline in a multi-string.
Example
str='''Welcome to class! \nI am your professor \nplease take your seat'"
print(str)
OUTPUT

As we discussed above, the newline character(\n) is used before the string we want to display in a newline. Here gives you the output of the above technique.
Technique 2: Adding NewLine to Python List
As we know, the python list is considered a dynamic array that stores multiple types of elements into it. For this technique, the string.join() function is used to add new lines for the list elements.
Syntax
'\n'.join(list)
Example
list=['Java','python','Cpp']
print("List before adding a newline character:",list)
list='\n'.join(list)
print("List after adding newline character:\n",list)
OUTPUT

Technique 3: Displaying NewLine using Print Statement
We can use multiple print statements to print output in a new line. The newline character(\n) is added to the print() function for displaying string on a new line.
Syntax
print("str\nstr2\n.....\nsrtN")
Example
print("Hello all")
print("good evening")
print("Let us start learning")
OUTPUT

As we discussed above, by giving many print() functions in many lines, the result will be displayed in a new line until and unless there are no more print() statements.
Technique 4: By adding NewLine Character within Python f-String
Python f-string will sequentially represent the statements of strings. To add a newline character within an f-string, use the below syntax:
Syntax
newline='\n'
string=f"string11{newline}string2"
Example
newline='\n'
string=f"java{newline}python{newline}CPP"
print(string)
OUTPUT

From the above output, we noticed that by using the newline character within the f-string, it would be displayed sequentially.
Technique 5: Printing a NewLine on Console
At the starting stage of python, it is more important to know that the execution of python functions on the console. For adding a newline on the console just follow below code.
Syntax
Print ("string 1\nstring 2")
Example
print("Python\nJava\nCpp")
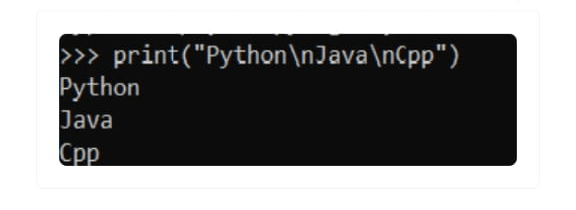
From the above example we observed that printing a newline on console works in python. Console is nothing but a SHELL and it is command -line interpreter that takes an input from the user that is it takes one command at a time and it will interpret that command. If the given input is error free then that input will run the command and it gives the output, if any error occurs it shows the error message.
In other words, python console will enable the execution of python commands as well as scripts one after the other. The console in python will appear as a tool window when we choose the particular command on TOOLS menu. There is a shortcut for opening python console that is ctrl+alt+S, it will navigate to a KEYMAP that specifies a shortcut for MAINMENU | TOOLS | PYTHON or DEBUG CONSOLE.
The main advantage to use python console using PyCharm is we can get main IDE features, such as completion of the code, analysis of the code, and also quick fixes.
Technique 6: By writing a NewLine into a file
By using new line character “\n”, it can be appended with Python file with the given below syntax.
Syntax
file_object.write("\n")
Example
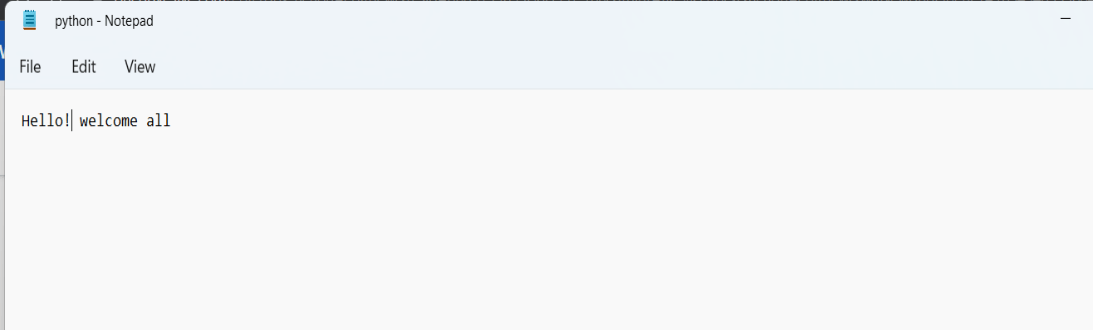
From the above picture, we have used hello.txt file which we gave a predefined content. It is a python text file.
Exampl
import os
file= "/hello.txt"
with open(file,'a') as file:
file.wrie("\n")
OUTPUT
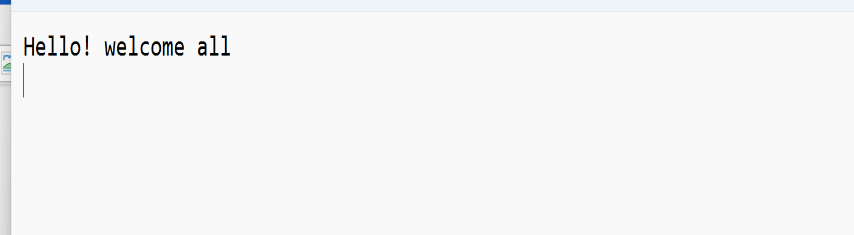
Above example shows that a new line was added to file content. From the above code we have used hello.txt file as a file name and opens in an append mode.
Advantages of NewLine Character
- The main advantage of a newline character is it is most important in any programming languages because it allows a programmer to understand the code in a better way and can search for the line break mainly in text files.
- It says that line ends in this place and remaining strings would be displayed in the new line.
- New line character will allow us to read in a efficient manner without collision of words between them.
Applications of NewLine Character
- A new line character will shift the cursor to the next line without moving the print function.
- We can add newline character in a multiple line string that is, it consists of many strings followed by “\n”.
- New line character can be added using python list.